How to prevent from docker to stop/remove the container on error
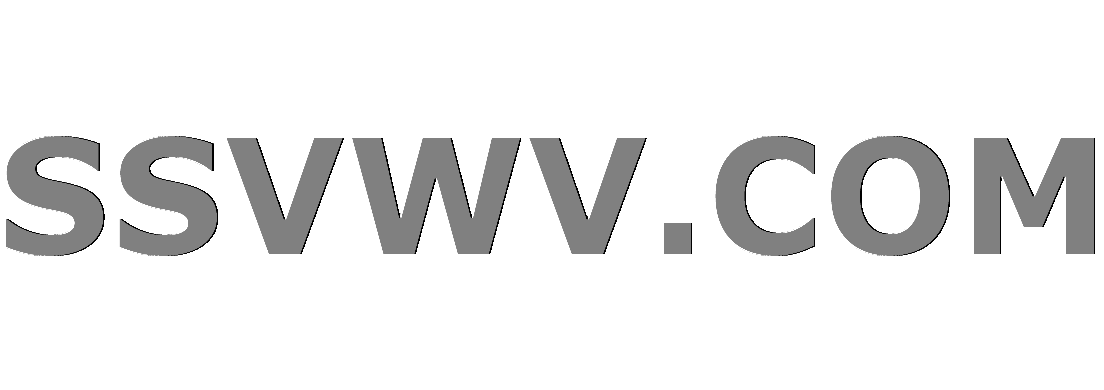
Multi tool use
up vote
1
down vote
favorite
Consider I have a Jenkins groovy code that runs docker container and some bash will run inside of it:
docker.withRegistry(dockerRegistry, dockerCredentials) {
docker.image(new String(dockerImage + ":" + dockerVersion)).inside(mountCommand) {
try {
withEnv(["AWS_PROFILE=${mappings['stackEnv']}",
"FULL_PACKAGE_VERSION=${mappings['revision']}",
"ARTIFACTORY_USER=${IC_ARTIFACTORY_USER}"]) {
withCredentials([
string(credentialsId: IC_ARTIFACTORY_KEY, variable: 'ARTIFACTORY_KEY')
]) {
sh '''
bash -x Jenkinsfile-e2e-test.sh
'''
}
}
} finally { }
}
}
If Jenkinsfile-e2e-test.sh
falls for some reason then Jenkins will automatically stop and remove the container:
09:40:08 $ docker stop --time=1 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
09:40:09 $ docker rm -f 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
How do I prevent Jenkins to destroy the container on failure?
linux bash docker jenkins-pipeline jenkins-groovy
add a comment |
up vote
1
down vote
favorite
Consider I have a Jenkins groovy code that runs docker container and some bash will run inside of it:
docker.withRegistry(dockerRegistry, dockerCredentials) {
docker.image(new String(dockerImage + ":" + dockerVersion)).inside(mountCommand) {
try {
withEnv(["AWS_PROFILE=${mappings['stackEnv']}",
"FULL_PACKAGE_VERSION=${mappings['revision']}",
"ARTIFACTORY_USER=${IC_ARTIFACTORY_USER}"]) {
withCredentials([
string(credentialsId: IC_ARTIFACTORY_KEY, variable: 'ARTIFACTORY_KEY')
]) {
sh '''
bash -x Jenkinsfile-e2e-test.sh
'''
}
}
} finally { }
}
}
If Jenkinsfile-e2e-test.sh
falls for some reason then Jenkins will automatically stop and remove the container:
09:40:08 $ docker stop --time=1 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
09:40:09 $ docker rm -f 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
How do I prevent Jenkins to destroy the container on failure?
linux bash docker jenkins-pipeline jenkins-groovy
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Consider I have a Jenkins groovy code that runs docker container and some bash will run inside of it:
docker.withRegistry(dockerRegistry, dockerCredentials) {
docker.image(new String(dockerImage + ":" + dockerVersion)).inside(mountCommand) {
try {
withEnv(["AWS_PROFILE=${mappings['stackEnv']}",
"FULL_PACKAGE_VERSION=${mappings['revision']}",
"ARTIFACTORY_USER=${IC_ARTIFACTORY_USER}"]) {
withCredentials([
string(credentialsId: IC_ARTIFACTORY_KEY, variable: 'ARTIFACTORY_KEY')
]) {
sh '''
bash -x Jenkinsfile-e2e-test.sh
'''
}
}
} finally { }
}
}
If Jenkinsfile-e2e-test.sh
falls for some reason then Jenkins will automatically stop and remove the container:
09:40:08 $ docker stop --time=1 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
09:40:09 $ docker rm -f 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
How do I prevent Jenkins to destroy the container on failure?
linux bash docker jenkins-pipeline jenkins-groovy
Consider I have a Jenkins groovy code that runs docker container and some bash will run inside of it:
docker.withRegistry(dockerRegistry, dockerCredentials) {
docker.image(new String(dockerImage + ":" + dockerVersion)).inside(mountCommand) {
try {
withEnv(["AWS_PROFILE=${mappings['stackEnv']}",
"FULL_PACKAGE_VERSION=${mappings['revision']}",
"ARTIFACTORY_USER=${IC_ARTIFACTORY_USER}"]) {
withCredentials([
string(credentialsId: IC_ARTIFACTORY_KEY, variable: 'ARTIFACTORY_KEY')
]) {
sh '''
bash -x Jenkinsfile-e2e-test.sh
'''
}
}
} finally { }
}
}
If Jenkinsfile-e2e-test.sh
falls for some reason then Jenkins will automatically stop and remove the container:
09:40:08 $ docker stop --time=1 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
09:40:09 $ docker rm -f 6e78cf6d940cb1ca1cb1c729617fd3e6ba3fa4085c2750908dff8f2a1e7ffeed
How do I prevent Jenkins to destroy the container on failure?
linux bash docker jenkins-pipeline jenkins-groovy
linux bash docker jenkins-pipeline jenkins-groovy
edited 2 days ago
asked 2 days ago


Yuri
7281022
7281022
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
You can ensure the command will not fail using this :
bash -x Jenkinsfile-e2e-test.sh || true
Or even better :
bash -x Jenkinsfile-e2e-test.sh > e2e.log 2>&1 & || true
# check the log file and do something based on the log
add a comment |
up vote
2
down vote
Jenkins will always stop and remove the container, once the body of your .inside
command is finished. No matter if your bash script succeeds or fails.
The rational behind is that jenkins takes care of the housekeeping for you, to prevent that you end up with lots of stopped containers hanging around on your build machine.
This is the case for the Image.withRun
and Image.inside
methods.
If you want to have control over the lifecycle of the container you should use Image.run
:
Uses docker run to run the image, and returns a Container which you could stop later
(from docs)
Thanks, great explanation
– Yuri
2 days ago
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can ensure the command will not fail using this :
bash -x Jenkinsfile-e2e-test.sh || true
Or even better :
bash -x Jenkinsfile-e2e-test.sh > e2e.log 2>&1 & || true
# check the log file and do something based on the log
add a comment |
up vote
1
down vote
accepted
You can ensure the command will not fail using this :
bash -x Jenkinsfile-e2e-test.sh || true
Or even better :
bash -x Jenkinsfile-e2e-test.sh > e2e.log 2>&1 & || true
# check the log file and do something based on the log
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can ensure the command will not fail using this :
bash -x Jenkinsfile-e2e-test.sh || true
Or even better :
bash -x Jenkinsfile-e2e-test.sh > e2e.log 2>&1 & || true
# check the log file and do something based on the log
You can ensure the command will not fail using this :
bash -x Jenkinsfile-e2e-test.sh || true
Or even better :
bash -x Jenkinsfile-e2e-test.sh > e2e.log 2>&1 & || true
# check the log file and do something based on the log
answered 2 days ago
chenchuk
1,91521322
1,91521322
add a comment |
add a comment |
up vote
2
down vote
Jenkins will always stop and remove the container, once the body of your .inside
command is finished. No matter if your bash script succeeds or fails.
The rational behind is that jenkins takes care of the housekeeping for you, to prevent that you end up with lots of stopped containers hanging around on your build machine.
This is the case for the Image.withRun
and Image.inside
methods.
If you want to have control over the lifecycle of the container you should use Image.run
:
Uses docker run to run the image, and returns a Container which you could stop later
(from docs)
Thanks, great explanation
– Yuri
2 days ago
add a comment |
up vote
2
down vote
Jenkins will always stop and remove the container, once the body of your .inside
command is finished. No matter if your bash script succeeds or fails.
The rational behind is that jenkins takes care of the housekeeping for you, to prevent that you end up with lots of stopped containers hanging around on your build machine.
This is the case for the Image.withRun
and Image.inside
methods.
If you want to have control over the lifecycle of the container you should use Image.run
:
Uses docker run to run the image, and returns a Container which you could stop later
(from docs)
Thanks, great explanation
– Yuri
2 days ago
add a comment |
up vote
2
down vote
up vote
2
down vote
Jenkins will always stop and remove the container, once the body of your .inside
command is finished. No matter if your bash script succeeds or fails.
The rational behind is that jenkins takes care of the housekeeping for you, to prevent that you end up with lots of stopped containers hanging around on your build machine.
This is the case for the Image.withRun
and Image.inside
methods.
If you want to have control over the lifecycle of the container you should use Image.run
:
Uses docker run to run the image, and returns a Container which you could stop later
(from docs)
Jenkins will always stop and remove the container, once the body of your .inside
command is finished. No matter if your bash script succeeds or fails.
The rational behind is that jenkins takes care of the housekeeping for you, to prevent that you end up with lots of stopped containers hanging around on your build machine.
This is the case for the Image.withRun
and Image.inside
methods.
If you want to have control over the lifecycle of the container you should use Image.run
:
Uses docker run to run the image, and returns a Container which you could stop later
(from docs)
answered 2 days ago
fab
7231924
7231924
Thanks, great explanation
– Yuri
2 days ago
add a comment |
Thanks, great explanation
– Yuri
2 days ago
Thanks, great explanation
– Yuri
2 days ago
Thanks, great explanation
– Yuri
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53203812%2fhow-to-prevent-from-docker-to-stop-remove-the-container-on-error%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
9 9,4jUZnijKTxog0STrVYRE9g5YsDrw8CH9v zL8ebVyX,i,izWNeSfieU s1Vxzz5,Zuu EIMu,L5,fNp1mg8