Ruby - Grouping data from CSV file by a column
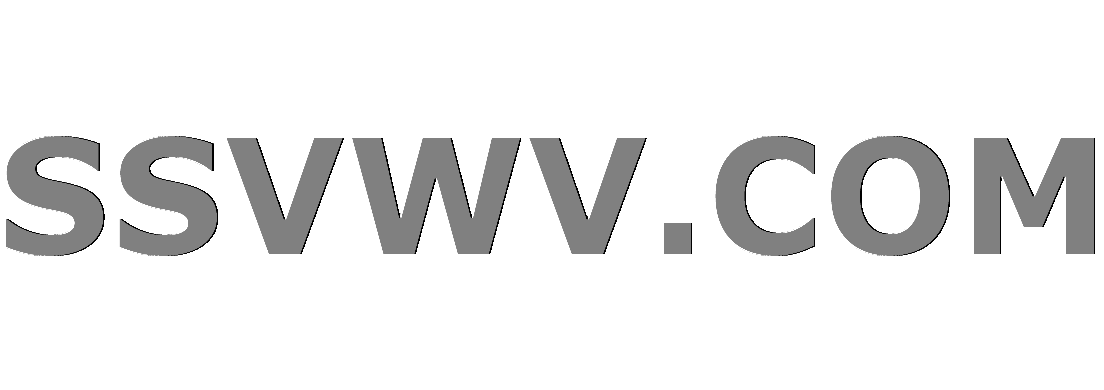
Multi tool use
up vote
1
down vote
favorite
I have a CSV file that looks like this:
+---------+----------+
| Name | Stream |
+---------+----------+
| Jacob | Computer |
| Ryan | Arts |
| Bob | Computer |
| Charlie | Science |
| Grace | Arts |
+---------+----------+
I need to read this CSV file and group the students based on their stream. The output should be like this:
Computer
----------
Jacob
Bob
Arts
------
Ryan
Grace
Science
--------
Charlie
I tried to use group_by
, but was not sure how and where to use it. Any help would be much appreciated.
ruby csv group-by
add a comment |
up vote
1
down vote
favorite
I have a CSV file that looks like this:
+---------+----------+
| Name | Stream |
+---------+----------+
| Jacob | Computer |
| Ryan | Arts |
| Bob | Computer |
| Charlie | Science |
| Grace | Arts |
+---------+----------+
I need to read this CSV file and group the students based on their stream. The output should be like this:
Computer
----------
Jacob
Bob
Arts
------
Ryan
Grace
Science
--------
Charlie
I tried to use group_by
, but was not sure how and where to use it. Any help would be much appreciated.
ruby csv group-by
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a CSV file that looks like this:
+---------+----------+
| Name | Stream |
+---------+----------+
| Jacob | Computer |
| Ryan | Arts |
| Bob | Computer |
| Charlie | Science |
| Grace | Arts |
+---------+----------+
I need to read this CSV file and group the students based on their stream. The output should be like this:
Computer
----------
Jacob
Bob
Arts
------
Ryan
Grace
Science
--------
Charlie
I tried to use group_by
, but was not sure how and where to use it. Any help would be much appreciated.
ruby csv group-by
I have a CSV file that looks like this:
+---------+----------+
| Name | Stream |
+---------+----------+
| Jacob | Computer |
| Ryan | Arts |
| Bob | Computer |
| Charlie | Science |
| Grace | Arts |
+---------+----------+
I need to read this CSV file and group the students based on their stream. The output should be like this:
Computer
----------
Jacob
Bob
Arts
------
Ryan
Grace
Science
--------
Charlie
I tried to use group_by
, but was not sure how and where to use it. Any help would be much appreciated.
ruby csv group-by
ruby csv group-by
edited 2 days ago


VAD
1,69131324
1,69131324
asked 2 days ago
Archana K
112
112
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago
add a comment |
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
I assume you have csv file with the following content:
Name,Stream
Jacob,Computer
Ryan,Arts
Bob,Computer
Charlie,Science
Grace,Arts
You can use something like this
require 'csv'
result = {}
file = File.read('path_to_your_file')
csv = CSV.parse(file, headers: true)
csv.each do |row|
if result[row[1]]
result[row[1]].push row[0]
else
result[row[1]] = [row[0]]
end
end
You'll get result
variable containing a hash where every stream will be associated with an array of names
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in theputs.key
statement
– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I assume you have csv file with the following content:
Name,Stream
Jacob,Computer
Ryan,Arts
Bob,Computer
Charlie,Science
Grace,Arts
You can use something like this
require 'csv'
result = {}
file = File.read('path_to_your_file')
csv = CSV.parse(file, headers: true)
csv.each do |row|
if result[row[1]]
result[row[1]].push row[0]
else
result[row[1]] = [row[0]]
end
end
You'll get result
variable containing a hash where every stream will be associated with an array of names
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in theputs.key
statement
– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
add a comment |
up vote
0
down vote
accepted
I assume you have csv file with the following content:
Name,Stream
Jacob,Computer
Ryan,Arts
Bob,Computer
Charlie,Science
Grace,Arts
You can use something like this
require 'csv'
result = {}
file = File.read('path_to_your_file')
csv = CSV.parse(file, headers: true)
csv.each do |row|
if result[row[1]]
result[row[1]].push row[0]
else
result[row[1]] = [row[0]]
end
end
You'll get result
variable containing a hash where every stream will be associated with an array of names
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in theputs.key
statement
– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I assume you have csv file with the following content:
Name,Stream
Jacob,Computer
Ryan,Arts
Bob,Computer
Charlie,Science
Grace,Arts
You can use something like this
require 'csv'
result = {}
file = File.read('path_to_your_file')
csv = CSV.parse(file, headers: true)
csv.each do |row|
if result[row[1]]
result[row[1]].push row[0]
else
result[row[1]] = [row[0]]
end
end
You'll get result
variable containing a hash where every stream will be associated with an array of names
I assume you have csv file with the following content:
Name,Stream
Jacob,Computer
Ryan,Arts
Bob,Computer
Charlie,Science
Grace,Arts
You can use something like this
require 'csv'
result = {}
file = File.read('path_to_your_file')
csv = CSV.parse(file, headers: true)
csv.each do |row|
if result[row[1]]
result[row[1]].push row[0]
else
result[row[1]] = [row[0]]
end
end
You'll get result
variable containing a hash where every stream will be associated with an array of names
edited 2 days ago
answered 2 days ago


VAD
1,69131324
1,69131324
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in theputs.key
statement
– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
add a comment |
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in theputs.key
statement
– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
Great, that works beautifully. Right now my output is {"Computer"=>["Jacob", "Bob"], "Arts"=>["Ryan", "Grace"], "Science"=>["Charlie"]} What would help is if I could get it in the format of course name followed by the student names in separate lines (as illustrated in the question). I am trying to do that but the fact that the 'result' is an array seems to be making it slightly more complicated.
– Archana K
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
result.keys.each { |key| puts; puts.key; puts '-----'; result[key].each { |val| puts val } }
– VAD
2 days ago
Perfect! You might want to remove the . character in the
puts.key
statement– Archana K
2 days ago
Perfect! You might want to remove the . character in the
puts.key
statement– Archana K
2 days ago
sure, but I can't :)
– VAD
2 days ago
sure, but I can't :)
– VAD
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53203820%2fruby-grouping-data-from-csv-file-by-a-column%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sz BuUXNby YQb21W
Is that what you literally have? Then that is not a CSV file.
– sawa
2 days ago
Show what you tried.
– sawa
2 days ago
What I have is indeed a csv file; I have just put it down in a tabular format so that it looks better and is easier to read and understand.
– Archana K
2 days ago
Also as I mentioned, since I am new to the language I am not sure on the approach that I should take to get this done.
– Archana K
2 days ago