Implementation of find the largest and the n/3 largest elements
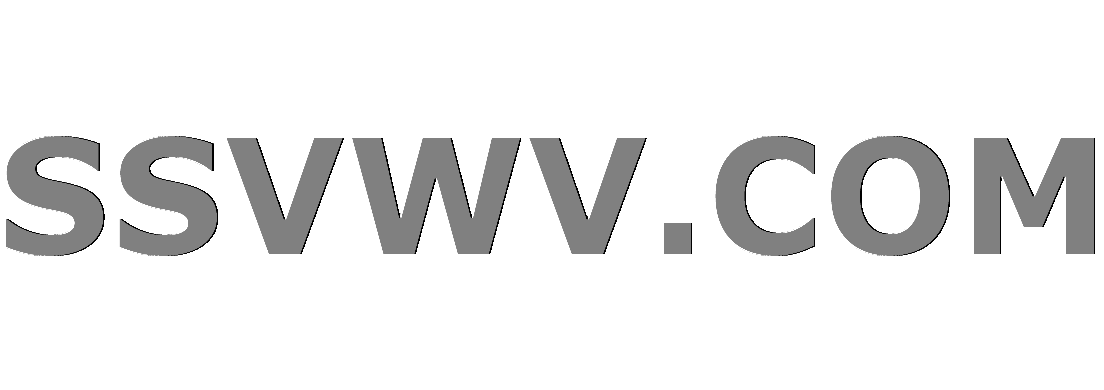
Multi tool use
up vote
3
down vote
favorite
Suppose you get numbers all the time and you don't know how many numbers will come at the beginning. At any time I want to be able to output the largest number and the n/3
largest number (where n
is the number of numbers entered up to this point) immediately (in O(1))
. The maximum time for entering new numbers is O(log(n))
.
What is the best way to implement this ?
algorithm
add a comment |
up vote
3
down vote
favorite
Suppose you get numbers all the time and you don't know how many numbers will come at the beginning. At any time I want to be able to output the largest number and the n/3
largest number (where n
is the number of numbers entered up to this point) immediately (in O(1))
. The maximum time for entering new numbers is O(log(n))
.
What is the best way to implement this ?
algorithm
1
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
1
Largest is easy. Then/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.
– user3386109
Nov 9 at 21:03
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
Suppose you get numbers all the time and you don't know how many numbers will come at the beginning. At any time I want to be able to output the largest number and the n/3
largest number (where n
is the number of numbers entered up to this point) immediately (in O(1))
. The maximum time for entering new numbers is O(log(n))
.
What is the best way to implement this ?
algorithm
Suppose you get numbers all the time and you don't know how many numbers will come at the beginning. At any time I want to be able to output the largest number and the n/3
largest number (where n
is the number of numbers entered up to this point) immediately (in O(1))
. The maximum time for entering new numbers is O(log(n))
.
What is the best way to implement this ?
algorithm
algorithm
edited Nov 9 at 20:57
bobbyrne01
2,09343791
2,09343791
asked Nov 9 at 20:35
azeug
212
212
1
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
1
Largest is easy. Then/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.
– user3386109
Nov 9 at 21:03
add a comment |
1
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
1
Largest is easy. Then/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.
– user3386109
Nov 9 at 21:03
1
1
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
1
1
Largest is easy. The
n/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.– user3386109
Nov 9 at 21:03
Largest is easy. The
n/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.– user3386109
Nov 9 at 21:03
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
You could implement it with two heaps: a min-heap (A), and a max-heap (B).
The idea is to store in A the n/3 greatest values, and the rest in B so that the maximum in B is the n/3 greatest value (counting zero-based). Assuming that n/3 is truncated down in case n is not a multiple of three, and that the resulting value is zero-based (n/3 == 0
means the maximum is also the n/3 greatest element), this means that the following invariance must be maintained:
A.size == floor((A.size + B.size)/3)
...which comes down to:
0 <= B.size - 2*A.size < 3
The above condition might vary a bit depending on the interpretation what the n/3 element is (is it 0-based, 1-based, rounded, truncated,...), and some special care might be needed when there are fewer than 3 elements in the total collection, depending on that definition.
For adding a value v you would first decide in which heap it belongs. If it is smaller than the current n/3th greatest value -- the max value in B -- then it belongs in B, otherwise in A. After that addition the invariance might be broken and must be restored by pulling one value from either heap and adding it to the other.
More formally v is added as follows:
if v < B.peek():
B.add(v)
if B.size - 2*A.size >= 3:
A.add(B.pull())
else:
A.add(v)
if B.size - 2*A.size < 0:
B.add(A.pull())
The implementation for getting the n/3th greatest value would be:
B.peek()
The time complexity for the above used methods is:
- size: O(1)
- peek: O(1)
- pull: O(logn)
- add: O(logn)
To maintain the overall maximum is an easy task. The heaps only serve for keeping track of the n/3th greatest value.
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name forpull
isextract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.
– trincot
Nov 9 at 23:11
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You could implement it with two heaps: a min-heap (A), and a max-heap (B).
The idea is to store in A the n/3 greatest values, and the rest in B so that the maximum in B is the n/3 greatest value (counting zero-based). Assuming that n/3 is truncated down in case n is not a multiple of three, and that the resulting value is zero-based (n/3 == 0
means the maximum is also the n/3 greatest element), this means that the following invariance must be maintained:
A.size == floor((A.size + B.size)/3)
...which comes down to:
0 <= B.size - 2*A.size < 3
The above condition might vary a bit depending on the interpretation what the n/3 element is (is it 0-based, 1-based, rounded, truncated,...), and some special care might be needed when there are fewer than 3 elements in the total collection, depending on that definition.
For adding a value v you would first decide in which heap it belongs. If it is smaller than the current n/3th greatest value -- the max value in B -- then it belongs in B, otherwise in A. After that addition the invariance might be broken and must be restored by pulling one value from either heap and adding it to the other.
More formally v is added as follows:
if v < B.peek():
B.add(v)
if B.size - 2*A.size >= 3:
A.add(B.pull())
else:
A.add(v)
if B.size - 2*A.size < 0:
B.add(A.pull())
The implementation for getting the n/3th greatest value would be:
B.peek()
The time complexity for the above used methods is:
- size: O(1)
- peek: O(1)
- pull: O(logn)
- add: O(logn)
To maintain the overall maximum is an easy task. The heaps only serve for keeping track of the n/3th greatest value.
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name forpull
isextract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.
– trincot
Nov 9 at 23:11
add a comment |
up vote
3
down vote
accepted
You could implement it with two heaps: a min-heap (A), and a max-heap (B).
The idea is to store in A the n/3 greatest values, and the rest in B so that the maximum in B is the n/3 greatest value (counting zero-based). Assuming that n/3 is truncated down in case n is not a multiple of three, and that the resulting value is zero-based (n/3 == 0
means the maximum is also the n/3 greatest element), this means that the following invariance must be maintained:
A.size == floor((A.size + B.size)/3)
...which comes down to:
0 <= B.size - 2*A.size < 3
The above condition might vary a bit depending on the interpretation what the n/3 element is (is it 0-based, 1-based, rounded, truncated,...), and some special care might be needed when there are fewer than 3 elements in the total collection, depending on that definition.
For adding a value v you would first decide in which heap it belongs. If it is smaller than the current n/3th greatest value -- the max value in B -- then it belongs in B, otherwise in A. After that addition the invariance might be broken and must be restored by pulling one value from either heap and adding it to the other.
More formally v is added as follows:
if v < B.peek():
B.add(v)
if B.size - 2*A.size >= 3:
A.add(B.pull())
else:
A.add(v)
if B.size - 2*A.size < 0:
B.add(A.pull())
The implementation for getting the n/3th greatest value would be:
B.peek()
The time complexity for the above used methods is:
- size: O(1)
- peek: O(1)
- pull: O(logn)
- add: O(logn)
To maintain the overall maximum is an easy task. The heaps only serve for keeping track of the n/3th greatest value.
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name forpull
isextract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.
– trincot
Nov 9 at 23:11
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You could implement it with two heaps: a min-heap (A), and a max-heap (B).
The idea is to store in A the n/3 greatest values, and the rest in B so that the maximum in B is the n/3 greatest value (counting zero-based). Assuming that n/3 is truncated down in case n is not a multiple of three, and that the resulting value is zero-based (n/3 == 0
means the maximum is also the n/3 greatest element), this means that the following invariance must be maintained:
A.size == floor((A.size + B.size)/3)
...which comes down to:
0 <= B.size - 2*A.size < 3
The above condition might vary a bit depending on the interpretation what the n/3 element is (is it 0-based, 1-based, rounded, truncated,...), and some special care might be needed when there are fewer than 3 elements in the total collection, depending on that definition.
For adding a value v you would first decide in which heap it belongs. If it is smaller than the current n/3th greatest value -- the max value in B -- then it belongs in B, otherwise in A. After that addition the invariance might be broken and must be restored by pulling one value from either heap and adding it to the other.
More formally v is added as follows:
if v < B.peek():
B.add(v)
if B.size - 2*A.size >= 3:
A.add(B.pull())
else:
A.add(v)
if B.size - 2*A.size < 0:
B.add(A.pull())
The implementation for getting the n/3th greatest value would be:
B.peek()
The time complexity for the above used methods is:
- size: O(1)
- peek: O(1)
- pull: O(logn)
- add: O(logn)
To maintain the overall maximum is an easy task. The heaps only serve for keeping track of the n/3th greatest value.
You could implement it with two heaps: a min-heap (A), and a max-heap (B).
The idea is to store in A the n/3 greatest values, and the rest in B so that the maximum in B is the n/3 greatest value (counting zero-based). Assuming that n/3 is truncated down in case n is not a multiple of three, and that the resulting value is zero-based (n/3 == 0
means the maximum is also the n/3 greatest element), this means that the following invariance must be maintained:
A.size == floor((A.size + B.size)/3)
...which comes down to:
0 <= B.size - 2*A.size < 3
The above condition might vary a bit depending on the interpretation what the n/3 element is (is it 0-based, 1-based, rounded, truncated,...), and some special care might be needed when there are fewer than 3 elements in the total collection, depending on that definition.
For adding a value v you would first decide in which heap it belongs. If it is smaller than the current n/3th greatest value -- the max value in B -- then it belongs in B, otherwise in A. After that addition the invariance might be broken and must be restored by pulling one value from either heap and adding it to the other.
More formally v is added as follows:
if v < B.peek():
B.add(v)
if B.size - 2*A.size >= 3:
A.add(B.pull())
else:
A.add(v)
if B.size - 2*A.size < 0:
B.add(A.pull())
The implementation for getting the n/3th greatest value would be:
B.peek()
The time complexity for the above used methods is:
- size: O(1)
- peek: O(1)
- pull: O(logn)
- add: O(logn)
To maintain the overall maximum is an easy task. The heaps only serve for keeping track of the n/3th greatest value.
edited Nov 9 at 22:33
answered Nov 9 at 21:46


trincot
114k1477109
114k1477109
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name forpull
isextract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.
– trincot
Nov 9 at 23:11
add a comment |
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name forpull
isextract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.
– trincot
Nov 9 at 23:11
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Thank you very much!! But what do you mean with pull?
– azeug
Nov 9 at 23:06
Another name for
pull
is extract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.– trincot
Nov 9 at 23:11
Another name for
pull
is extract
. It means taking the value from the root of the heap, and removing it from the heap, which then needs to get a new root via the heapify algorithm.– trincot
Nov 9 at 23:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53232931%2fimplementation-of-find-the-largest-and-the-n-3-largest-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0OcDhwYljEjD4HiO,0uyDy Z,0XGcZINJsUWPymMxp3oJfetp3DNaho nGDJ0Xp
1
In essence this is a variant of a selection algorithm: cs.stackexchange.com/questions/1914/…
– Willem Van Onsem
Nov 9 at 20:46
1
Largest is easy. The
n/3
largest I would handle with two heaps, a min heap and and a max heap. There are many variants but even the simple ones meet your requirements.– user3386109
Nov 9 at 21:03