Find the row with the highest value in SQL
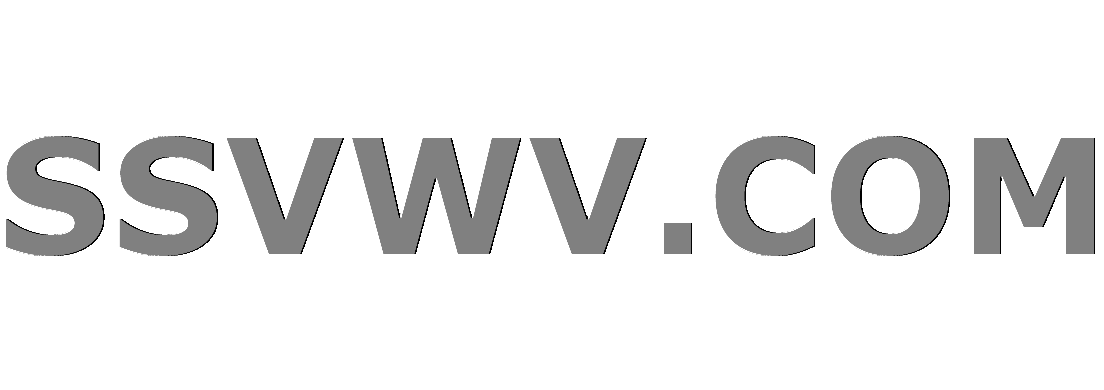
Multi tool use
up vote
1
down vote
favorite
According to the schema, the Orders
table has the following columns:
OrderId integer, CustomerId integer, RetailerId integer, ProductId integer, Count integer
I'm trying to figure out what product has the highest number of orders for each retailer.
So, to sum up all the orders per product, I have the following query:
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
ORDER BY RetailerId, ProductTotal DESC;
This gives an output like this:
RETAILERID PRODUCTID PRODUCTTOTAL
---------- ---------- ------------
1 5 115
1 10 45
1 1 15
1 4 2
1 8 1
2 9 12
2 11 10
2 7 1
3 3 3
4 2 1
5 11 1
Now, all I want to do is find the product with the highest number of orders per retailer. Right now, it shows all the products; I want only one.
I have tried way too many things. Following is one particularly abhorrent example:
SELECT O.RetailerId, O.ProductId, O.ProductTotal
FROM (
SELECT Orders.ProductId, MAX(ProductTotal) AS MaxProductTotal
FROM (
SELECT Orders.ProductId AS PID, SUM(Orders.Count) AS ProductTotal
FROM Orders
GROUP BY Orders.ProductId
) AS O INNER JOIN Orders ON Orders.ProductId = PID
GROUP BY Orders.ProductId
) AS X INNER JOIN O ON O.RetailerId = X.RetailerId AND O.ProductTotal = X.MaxProductTotal;
The solution to this is probably the simplest thing ever, but I just can't right now. So, I'd like some help.
sql oracle greatest-n-per-group
add a comment |
up vote
1
down vote
favorite
According to the schema, the Orders
table has the following columns:
OrderId integer, CustomerId integer, RetailerId integer, ProductId integer, Count integer
I'm trying to figure out what product has the highest number of orders for each retailer.
So, to sum up all the orders per product, I have the following query:
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
ORDER BY RetailerId, ProductTotal DESC;
This gives an output like this:
RETAILERID PRODUCTID PRODUCTTOTAL
---------- ---------- ------------
1 5 115
1 10 45
1 1 15
1 4 2
1 8 1
2 9 12
2 11 10
2 7 1
3 3 3
4 2 1
5 11 1
Now, all I want to do is find the product with the highest number of orders per retailer. Right now, it shows all the products; I want only one.
I have tried way too many things. Following is one particularly abhorrent example:
SELECT O.RetailerId, O.ProductId, O.ProductTotal
FROM (
SELECT Orders.ProductId, MAX(ProductTotal) AS MaxProductTotal
FROM (
SELECT Orders.ProductId AS PID, SUM(Orders.Count) AS ProductTotal
FROM Orders
GROUP BY Orders.ProductId
) AS O INNER JOIN Orders ON Orders.ProductId = PID
GROUP BY Orders.ProductId
) AS X INNER JOIN O ON O.RetailerId = X.RetailerId AND O.ProductTotal = X.MaxProductTotal;
The solution to this is probably the simplest thing ever, but I just can't right now. So, I'd like some help.
sql oracle greatest-n-per-group
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
According to the schema, the Orders
table has the following columns:
OrderId integer, CustomerId integer, RetailerId integer, ProductId integer, Count integer
I'm trying to figure out what product has the highest number of orders for each retailer.
So, to sum up all the orders per product, I have the following query:
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
ORDER BY RetailerId, ProductTotal DESC;
This gives an output like this:
RETAILERID PRODUCTID PRODUCTTOTAL
---------- ---------- ------------
1 5 115
1 10 45
1 1 15
1 4 2
1 8 1
2 9 12
2 11 10
2 7 1
3 3 3
4 2 1
5 11 1
Now, all I want to do is find the product with the highest number of orders per retailer. Right now, it shows all the products; I want only one.
I have tried way too many things. Following is one particularly abhorrent example:
SELECT O.RetailerId, O.ProductId, O.ProductTotal
FROM (
SELECT Orders.ProductId, MAX(ProductTotal) AS MaxProductTotal
FROM (
SELECT Orders.ProductId AS PID, SUM(Orders.Count) AS ProductTotal
FROM Orders
GROUP BY Orders.ProductId
) AS O INNER JOIN Orders ON Orders.ProductId = PID
GROUP BY Orders.ProductId
) AS X INNER JOIN O ON O.RetailerId = X.RetailerId AND O.ProductTotal = X.MaxProductTotal;
The solution to this is probably the simplest thing ever, but I just can't right now. So, I'd like some help.
sql oracle greatest-n-per-group
According to the schema, the Orders
table has the following columns:
OrderId integer, CustomerId integer, RetailerId integer, ProductId integer, Count integer
I'm trying to figure out what product has the highest number of orders for each retailer.
So, to sum up all the orders per product, I have the following query:
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
ORDER BY RetailerId, ProductTotal DESC;
This gives an output like this:
RETAILERID PRODUCTID PRODUCTTOTAL
---------- ---------- ------------
1 5 115
1 10 45
1 1 15
1 4 2
1 8 1
2 9 12
2 11 10
2 7 1
3 3 3
4 2 1
5 11 1
Now, all I want to do is find the product with the highest number of orders per retailer. Right now, it shows all the products; I want only one.
I have tried way too many things. Following is one particularly abhorrent example:
SELECT O.RetailerId, O.ProductId, O.ProductTotal
FROM (
SELECT Orders.ProductId, MAX(ProductTotal) AS MaxProductTotal
FROM (
SELECT Orders.ProductId AS PID, SUM(Orders.Count) AS ProductTotal
FROM Orders
GROUP BY Orders.ProductId
) AS O INNER JOIN Orders ON Orders.ProductId = PID
GROUP BY Orders.ProductId
) AS X INNER JOIN O ON O.RetailerId = X.RetailerId AND O.ProductTotal = X.MaxProductTotal;
The solution to this is probably the simplest thing ever, but I just can't right now. So, I'd like some help.
sql oracle greatest-n-per-group
sql oracle greatest-n-per-group
edited 14 hours ago
a_horse_with_no_name
284k45426524
284k45426524
asked 18 hours ago
Sam Fischer
9951327
9951327
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
up vote
0
down vote
You can try using window function row_number()
select * from
(
select *,row_number() over(partition by RetailerId order by ProductTotal desc) as rn from
(
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)A
)X where rn=1
add a comment |
up vote
0
down vote
you can use row_number()
with cte
with cte as
(
SELECT o.RetailerId,o.ProductId AS PID, SUM(o.Count) AS ProductTotal
FROM Orders o
GROUP BY o.ProductId,o.RetailerId
), cte2 as
(
select RetailerId,PID,ProductTotal,
row_number() over(partition by RetailerId order by ProductTotal desc) as rn
from cte
) select RetailerId,PID,ProductTotal from cte2 where rn=1
add a comment |
up vote
0
down vote
Have you tried using rank?
Try this query
select OrderC, retailer_id,product_id from ( select sum(count_order) as OrderC,retailer_id,product_id,row_number() over(order by OrderC desc) as RN from order_t group by retailer_id,product_id) as d where RN=1;
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Here is a version without sub-query using FIRST/LAST:
WITH t(RETAILERID, PRODUCTID, PRODUCTTOTAL) AS (
SELECT 1, 5, 115 FROM dual UNION ALL
SELECT 1, 10, 45 FROM dual UNION ALL
SELECT 1, 1, 15 FROM dual UNION ALL
SELECT 1, 4, 2 FROM dual UNION ALL
SELECT 1, 8, 1 FROM dual UNION ALL
SELECT 2, 9, 12 FROM dual UNION ALL
SELECT 2, 11, 10 FROM dual UNION ALL
SELECT 2, 7, 1 FROM dual UNION ALL
SELECT 3, 3, 3 FROM dual UNION ALL
SELECT 4, 2, 1 FROM dual UNION ALL
SELECT 5,11, 1 FROM dual)
SELECT
RETAILERID,
MAX(PRODUCTID) KEEP (DENSE_RANK LAST ORDER BY PRODUCTTOTAL) AS PRODUCTID,
MAX(PRODUCTTOTAL)
FROM t
GROUP BY RETAILERID;
+--------------------------------------+
|RETAILERID|PRODUCTID|MAX(PRODUCTTOTAL)|
+--------------------------------------+
|1 |5 |115 |
|2 |9 |12 |
|3 |3 |3 |
|4 |2 |1 |
|5 |11 |1 |
+--------------------------------------+
add a comment |
up vote
0
down vote
Select the maximum total per customer with a window function:
SELECT RetailerId, ProductId, ProductTotal
FROM
(
SELECT
RetailerId, ProductId, SUM(Count) AS ProductTotal,
MAX(SUM(Count)) OVER (PARTITION BY RetailerId) AS MaxProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)
WHERE ProductTotal = MaxProductTotal
ORDER BY RetailerId;
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You can try using window function row_number()
select * from
(
select *,row_number() over(partition by RetailerId order by ProductTotal desc) as rn from
(
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)A
)X where rn=1
add a comment |
up vote
0
down vote
You can try using window function row_number()
select * from
(
select *,row_number() over(partition by RetailerId order by ProductTotal desc) as rn from
(
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)A
)X where rn=1
add a comment |
up vote
0
down vote
up vote
0
down vote
You can try using window function row_number()
select * from
(
select *,row_number() over(partition by RetailerId order by ProductTotal desc) as rn from
(
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)A
)X where rn=1
You can try using window function row_number()
select * from
(
select *,row_number() over(partition by RetailerId order by ProductTotal desc) as rn from
(
SELECT RetailerId, ProductId, SUM(Count) AS ProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)A
)X where rn=1
answered 17 hours ago
fa06
7,7601515
7,7601515
add a comment |
add a comment |
up vote
0
down vote
you can use row_number()
with cte
with cte as
(
SELECT o.RetailerId,o.ProductId AS PID, SUM(o.Count) AS ProductTotal
FROM Orders o
GROUP BY o.ProductId,o.RetailerId
), cte2 as
(
select RetailerId,PID,ProductTotal,
row_number() over(partition by RetailerId order by ProductTotal desc) as rn
from cte
) select RetailerId,PID,ProductTotal from cte2 where rn=1
add a comment |
up vote
0
down vote
you can use row_number()
with cte
with cte as
(
SELECT o.RetailerId,o.ProductId AS PID, SUM(o.Count) AS ProductTotal
FROM Orders o
GROUP BY o.ProductId,o.RetailerId
), cte2 as
(
select RetailerId,PID,ProductTotal,
row_number() over(partition by RetailerId order by ProductTotal desc) as rn
from cte
) select RetailerId,PID,ProductTotal from cte2 where rn=1
add a comment |
up vote
0
down vote
up vote
0
down vote
you can use row_number()
with cte
with cte as
(
SELECT o.RetailerId,o.ProductId AS PID, SUM(o.Count) AS ProductTotal
FROM Orders o
GROUP BY o.ProductId,o.RetailerId
), cte2 as
(
select RetailerId,PID,ProductTotal,
row_number() over(partition by RetailerId order by ProductTotal desc) as rn
from cte
) select RetailerId,PID,ProductTotal from cte2 where rn=1
you can use row_number()
with cte
with cte as
(
SELECT o.RetailerId,o.ProductId AS PID, SUM(o.Count) AS ProductTotal
FROM Orders o
GROUP BY o.ProductId,o.RetailerId
), cte2 as
(
select RetailerId,PID,ProductTotal,
row_number() over(partition by RetailerId order by ProductTotal desc) as rn
from cte
) select RetailerId,PID,ProductTotal from cte2 where rn=1
answered 17 hours ago
Zaynul Abadin Tuhin
9,8502730
9,8502730
add a comment |
add a comment |
up vote
0
down vote
Have you tried using rank?
Try this query
select OrderC, retailer_id,product_id from ( select sum(count_order) as OrderC,retailer_id,product_id,row_number() over(order by OrderC desc) as RN from order_t group by retailer_id,product_id) as d where RN=1;
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Have you tried using rank?
Try this query
select OrderC, retailer_id,product_id from ( select sum(count_order) as OrderC,retailer_id,product_id,row_number() over(order by OrderC desc) as RN from order_t group by retailer_id,product_id) as d where RN=1;
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
Have you tried using rank?
Try this query
select OrderC, retailer_id,product_id from ( select sum(count_order) as OrderC,retailer_id,product_id,row_number() over(order by OrderC desc) as RN from order_t group by retailer_id,product_id) as d where RN=1;
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Have you tried using rank?
Try this query
select OrderC, retailer_id,product_id from ( select sum(count_order) as OrderC,retailer_id,product_id,row_number() over(order by OrderC desc) as RN from order_t group by retailer_id,product_id) as d where RN=1;
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 16 hours ago
Brekhnaa
162
162
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Brekhnaa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
up vote
0
down vote
Here is a version without sub-query using FIRST/LAST:
WITH t(RETAILERID, PRODUCTID, PRODUCTTOTAL) AS (
SELECT 1, 5, 115 FROM dual UNION ALL
SELECT 1, 10, 45 FROM dual UNION ALL
SELECT 1, 1, 15 FROM dual UNION ALL
SELECT 1, 4, 2 FROM dual UNION ALL
SELECT 1, 8, 1 FROM dual UNION ALL
SELECT 2, 9, 12 FROM dual UNION ALL
SELECT 2, 11, 10 FROM dual UNION ALL
SELECT 2, 7, 1 FROM dual UNION ALL
SELECT 3, 3, 3 FROM dual UNION ALL
SELECT 4, 2, 1 FROM dual UNION ALL
SELECT 5,11, 1 FROM dual)
SELECT
RETAILERID,
MAX(PRODUCTID) KEEP (DENSE_RANK LAST ORDER BY PRODUCTTOTAL) AS PRODUCTID,
MAX(PRODUCTTOTAL)
FROM t
GROUP BY RETAILERID;
+--------------------------------------+
|RETAILERID|PRODUCTID|MAX(PRODUCTTOTAL)|
+--------------------------------------+
|1 |5 |115 |
|2 |9 |12 |
|3 |3 |3 |
|4 |2 |1 |
|5 |11 |1 |
+--------------------------------------+
add a comment |
up vote
0
down vote
Here is a version without sub-query using FIRST/LAST:
WITH t(RETAILERID, PRODUCTID, PRODUCTTOTAL) AS (
SELECT 1, 5, 115 FROM dual UNION ALL
SELECT 1, 10, 45 FROM dual UNION ALL
SELECT 1, 1, 15 FROM dual UNION ALL
SELECT 1, 4, 2 FROM dual UNION ALL
SELECT 1, 8, 1 FROM dual UNION ALL
SELECT 2, 9, 12 FROM dual UNION ALL
SELECT 2, 11, 10 FROM dual UNION ALL
SELECT 2, 7, 1 FROM dual UNION ALL
SELECT 3, 3, 3 FROM dual UNION ALL
SELECT 4, 2, 1 FROM dual UNION ALL
SELECT 5,11, 1 FROM dual)
SELECT
RETAILERID,
MAX(PRODUCTID) KEEP (DENSE_RANK LAST ORDER BY PRODUCTTOTAL) AS PRODUCTID,
MAX(PRODUCTTOTAL)
FROM t
GROUP BY RETAILERID;
+--------------------------------------+
|RETAILERID|PRODUCTID|MAX(PRODUCTTOTAL)|
+--------------------------------------+
|1 |5 |115 |
|2 |9 |12 |
|3 |3 |3 |
|4 |2 |1 |
|5 |11 |1 |
+--------------------------------------+
add a comment |
up vote
0
down vote
up vote
0
down vote
Here is a version without sub-query using FIRST/LAST:
WITH t(RETAILERID, PRODUCTID, PRODUCTTOTAL) AS (
SELECT 1, 5, 115 FROM dual UNION ALL
SELECT 1, 10, 45 FROM dual UNION ALL
SELECT 1, 1, 15 FROM dual UNION ALL
SELECT 1, 4, 2 FROM dual UNION ALL
SELECT 1, 8, 1 FROM dual UNION ALL
SELECT 2, 9, 12 FROM dual UNION ALL
SELECT 2, 11, 10 FROM dual UNION ALL
SELECT 2, 7, 1 FROM dual UNION ALL
SELECT 3, 3, 3 FROM dual UNION ALL
SELECT 4, 2, 1 FROM dual UNION ALL
SELECT 5,11, 1 FROM dual)
SELECT
RETAILERID,
MAX(PRODUCTID) KEEP (DENSE_RANK LAST ORDER BY PRODUCTTOTAL) AS PRODUCTID,
MAX(PRODUCTTOTAL)
FROM t
GROUP BY RETAILERID;
+--------------------------------------+
|RETAILERID|PRODUCTID|MAX(PRODUCTTOTAL)|
+--------------------------------------+
|1 |5 |115 |
|2 |9 |12 |
|3 |3 |3 |
|4 |2 |1 |
|5 |11 |1 |
+--------------------------------------+
Here is a version without sub-query using FIRST/LAST:
WITH t(RETAILERID, PRODUCTID, PRODUCTTOTAL) AS (
SELECT 1, 5, 115 FROM dual UNION ALL
SELECT 1, 10, 45 FROM dual UNION ALL
SELECT 1, 1, 15 FROM dual UNION ALL
SELECT 1, 4, 2 FROM dual UNION ALL
SELECT 1, 8, 1 FROM dual UNION ALL
SELECT 2, 9, 12 FROM dual UNION ALL
SELECT 2, 11, 10 FROM dual UNION ALL
SELECT 2, 7, 1 FROM dual UNION ALL
SELECT 3, 3, 3 FROM dual UNION ALL
SELECT 4, 2, 1 FROM dual UNION ALL
SELECT 5,11, 1 FROM dual)
SELECT
RETAILERID,
MAX(PRODUCTID) KEEP (DENSE_RANK LAST ORDER BY PRODUCTTOTAL) AS PRODUCTID,
MAX(PRODUCTTOTAL)
FROM t
GROUP BY RETAILERID;
+--------------------------------------+
|RETAILERID|PRODUCTID|MAX(PRODUCTTOTAL)|
+--------------------------------------+
|1 |5 |115 |
|2 |9 |12 |
|3 |3 |3 |
|4 |2 |1 |
|5 |11 |1 |
+--------------------------------------+
answered 15 hours ago
Wernfried Domscheit
22.9k42757
22.9k42757
add a comment |
add a comment |
up vote
0
down vote
Select the maximum total per customer with a window function:
SELECT RetailerId, ProductId, ProductTotal
FROM
(
SELECT
RetailerId, ProductId, SUM(Count) AS ProductTotal,
MAX(SUM(Count)) OVER (PARTITION BY RetailerId) AS MaxProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)
WHERE ProductTotal = MaxProductTotal
ORDER BY RetailerId;
add a comment |
up vote
0
down vote
Select the maximum total per customer with a window function:
SELECT RetailerId, ProductId, ProductTotal
FROM
(
SELECT
RetailerId, ProductId, SUM(Count) AS ProductTotal,
MAX(SUM(Count)) OVER (PARTITION BY RetailerId) AS MaxProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)
WHERE ProductTotal = MaxProductTotal
ORDER BY RetailerId;
add a comment |
up vote
0
down vote
up vote
0
down vote
Select the maximum total per customer with a window function:
SELECT RetailerId, ProductId, ProductTotal
FROM
(
SELECT
RetailerId, ProductId, SUM(Count) AS ProductTotal,
MAX(SUM(Count)) OVER (PARTITION BY RetailerId) AS MaxProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)
WHERE ProductTotal = MaxProductTotal
ORDER BY RetailerId;
Select the maximum total per customer with a window function:
SELECT RetailerId, ProductId, ProductTotal
FROM
(
SELECT
RetailerId, ProductId, SUM(Count) AS ProductTotal,
MAX(SUM(Count)) OVER (PARTITION BY RetailerId) AS MaxProductTotal
FROM Orders
GROUP BY RetailerId, ProductId
)
WHERE ProductTotal = MaxProductTotal
ORDER BY RetailerId;
answered 14 hours ago
Thorsten Kettner
49.6k22441
49.6k22441
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53201588%2ffind-the-row-with-the-highest-value-in-sql%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
m1VlFzp F,S,WjdlJIo3HbUMFT5cvR6YrPez8,G4Cu,sY YqT qxpFB z5ezF,8chvvhMw1rk At