How to handle errors using Flow type hinting and Jest
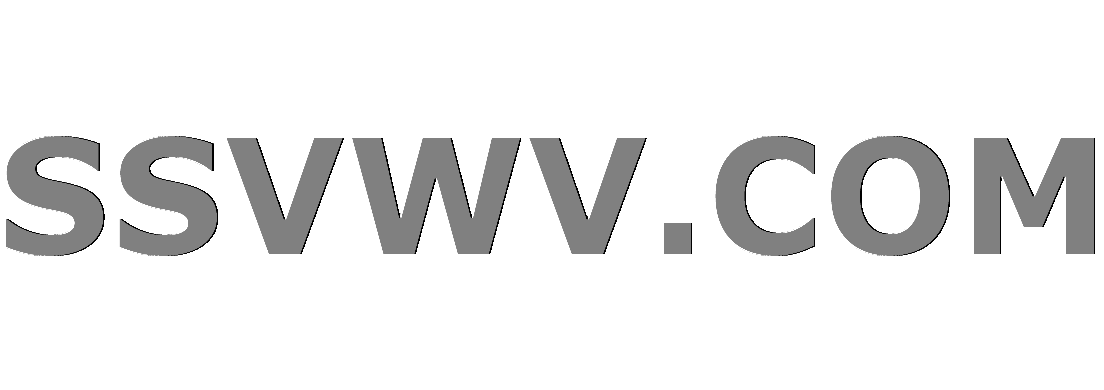
Multi tool use
up vote
3
down vote
favorite
I have a class defined like this:
class MyClass{
constructor(data:Array<any>){
...
}
}
And a basic test setup in Jest like this:
test('bad args', () => {
expect(new MyClass()).toThrow();
expect(new MyClass({})).toThrow();
expect(new MyClass('string')).toThrow();
});
I would expect these to error as the type hinting does not allow for empty constructor args and expects first arg to be array not an object or string.
Can anyone help explain how I persuade Jest to run the code through Flow and error where types are incorrect?
EDIT:
I also have run flow-typed install jest@23.6.0 //correct jest version
javascript jestjs flowtype
add a comment |
up vote
3
down vote
favorite
I have a class defined like this:
class MyClass{
constructor(data:Array<any>){
...
}
}
And a basic test setup in Jest like this:
test('bad args', () => {
expect(new MyClass()).toThrow();
expect(new MyClass({})).toThrow();
expect(new MyClass('string')).toThrow();
});
I would expect these to error as the type hinting does not allow for empty constructor args and expects first arg to be array not an object or string.
Can anyone help explain how I persuade Jest to run the code through Flow and error where types are incorrect?
EDIT:
I also have run flow-typed install jest@23.6.0 //correct jest version
javascript jestjs flowtype
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I have a class defined like this:
class MyClass{
constructor(data:Array<any>){
...
}
}
And a basic test setup in Jest like this:
test('bad args', () => {
expect(new MyClass()).toThrow();
expect(new MyClass({})).toThrow();
expect(new MyClass('string')).toThrow();
});
I would expect these to error as the type hinting does not allow for empty constructor args and expects first arg to be array not an object or string.
Can anyone help explain how I persuade Jest to run the code through Flow and error where types are incorrect?
EDIT:
I also have run flow-typed install jest@23.6.0 //correct jest version
javascript jestjs flowtype
I have a class defined like this:
class MyClass{
constructor(data:Array<any>){
...
}
}
And a basic test setup in Jest like this:
test('bad args', () => {
expect(new MyClass()).toThrow();
expect(new MyClass({})).toThrow();
expect(new MyClass('string')).toThrow();
});
I would expect these to error as the type hinting does not allow for empty constructor args and expects first arg to be array not an object or string.
Can anyone help explain how I persuade Jest to run the code through Flow and error where types are incorrect?
EDIT:
I also have run flow-typed install jest@23.6.0 //correct jest version
javascript jestjs flowtype
javascript jestjs flowtype
edited Nov 8 at 10:57
asked Nov 8 at 10:41


Mike Miller
2,03611220
2,03611220
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
The doc states that:
Babel will take your Flow code and strip out any type annotations.
So, flow can help you to find wrong call before build (usually at development time, in ide). And once you run flow
in your project - you can catch where is signature mismatch.
After compiling flow annotations are removed, and you have pure javascript.
So, if your constructor accepts any
arg, but only array is valid one, you can explicitly state that:
class MyClass {
constructor(data:*) {
if (!Array.isArray(data)) {
throw new Exception('Bad argument');
}
}
}
The above codebase will work if you specify data:Array<any>
as well, but you'll see errors at compiling time (so you can catch who to blame :) ).
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The doc states that:
Babel will take your Flow code and strip out any type annotations.
So, flow can help you to find wrong call before build (usually at development time, in ide). And once you run flow
in your project - you can catch where is signature mismatch.
After compiling flow annotations are removed, and you have pure javascript.
So, if your constructor accepts any
arg, but only array is valid one, you can explicitly state that:
class MyClass {
constructor(data:*) {
if (!Array.isArray(data)) {
throw new Exception('Bad argument');
}
}
}
The above codebase will work if you specify data:Array<any>
as well, but you'll see errors at compiling time (so you can catch who to blame :) ).
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
add a comment |
up vote
1
down vote
accepted
The doc states that:
Babel will take your Flow code and strip out any type annotations.
So, flow can help you to find wrong call before build (usually at development time, in ide). And once you run flow
in your project - you can catch where is signature mismatch.
After compiling flow annotations are removed, and you have pure javascript.
So, if your constructor accepts any
arg, but only array is valid one, you can explicitly state that:
class MyClass {
constructor(data:*) {
if (!Array.isArray(data)) {
throw new Exception('Bad argument');
}
}
}
The above codebase will work if you specify data:Array<any>
as well, but you'll see errors at compiling time (so you can catch who to blame :) ).
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The doc states that:
Babel will take your Flow code and strip out any type annotations.
So, flow can help you to find wrong call before build (usually at development time, in ide). And once you run flow
in your project - you can catch where is signature mismatch.
After compiling flow annotations are removed, and you have pure javascript.
So, if your constructor accepts any
arg, but only array is valid one, you can explicitly state that:
class MyClass {
constructor(data:*) {
if (!Array.isArray(data)) {
throw new Exception('Bad argument');
}
}
}
The above codebase will work if you specify data:Array<any>
as well, but you'll see errors at compiling time (so you can catch who to blame :) ).
The doc states that:
Babel will take your Flow code and strip out any type annotations.
So, flow can help you to find wrong call before build (usually at development time, in ide). And once you run flow
in your project - you can catch where is signature mismatch.
After compiling flow annotations are removed, and you have pure javascript.
So, if your constructor accepts any
arg, but only array is valid one, you can explicitly state that:
class MyClass {
constructor(data:*) {
if (!Array.isArray(data)) {
throw new Exception('Bad argument');
}
}
}
The above codebase will work if you specify data:Array<any>
as well, but you'll see errors at compiling time (so you can catch who to blame :) ).
answered Nov 8 at 14:51


Alex
2,762620
2,762620
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
add a comment |
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
Thanks, that makes perfect sense - hadn't thought it through properly
– Mike Miller
Nov 8 at 14:59
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206034%2fhow-to-handle-errors-using-flow-type-hinting-and-jest%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
jo89U cwn 8Zcigf1G 4 PMnH PAT,i,0 1D hoLXHr791uN6wqQfNiboTMzoYSTn4Ux,ui J,i,VxYyFNrWx1 rkNnDA1Xga