Sorted dictionary to linked list
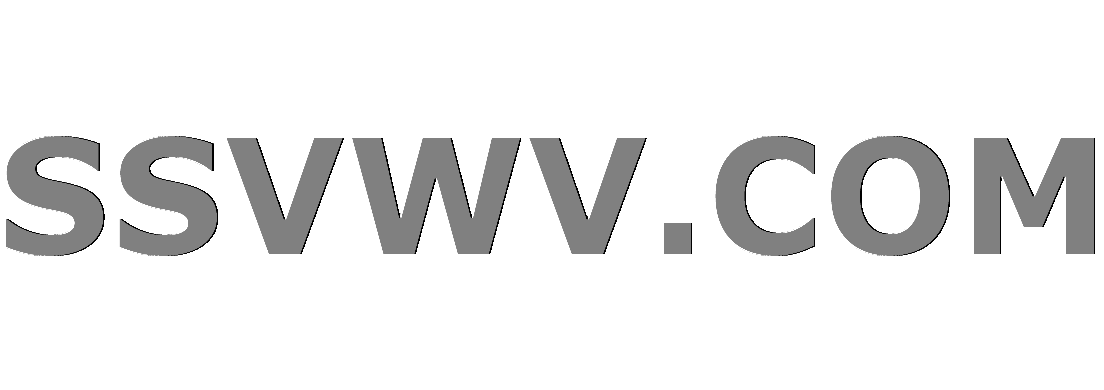
Multi tool use
up vote
-1
down vote
favorite
I have a program that reads an ASCII text file and counts the number of times each character appears in the file. It then creates an output file with each character and the number of times it appears. An example output is:
H(72) = 1
e(101) = 1
l(108) = 2
o(111) = 1
.(46) = 1
In my program, I use a sorted dictionary to store the characters. I want to use a Linked List instead to store the values but I am note sure the best way to go about this. My program is below:
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.IO;
using System.Text;
using System.Reflection;
//CharacterFrequency class
namespace ASCII
{
class CharacterFrequency
{
private char ch;
private int frequency;
public char Char
{
get { return ch; }
set { ch = value; }
}
public int Frequency
{
get { return frequency; }
set { frequency = value; }
}
}
class Counter
{
public string InputFileName = "example.txt";
public string OutputFileName = "example.txt";
//use a sorted dictionary to store values and keys of characters
public static SortedDictionary<char, ulong> Count(string stringToCount)
{
SortedDictionary<char, ulong> characterCount = new SortedDictionary<char, ulong>();
//loop through the sorted dictionary
foreach (var character in stringToCount)
{
if (!characterCount.ContainsKey(character))
{
characterCount.Add(character, 1);
}
else
{
characterCount[character]++;
}
}
return characterCount;
}
static void Main(string args)
{
CharacterFrequency charfreq = new CharacterFrequency();
Counter c = new Counter();
try
{
//gets input ASCII file
Console.WriteLine("Enter the input file path: ");
c.InputFileName = Console.ReadLine();
//gets output file path location
Console.WriteLine("Enter the output file path: ");
c.OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(c.OutputFileName);
string data = File.ReadAllText(c.InputFileName);
var count = Counter.Count(data);
foreach (var character in count)
{
streamWriter.WriteLine(character.Key + "(" + (int)character.Key + ")" + "t" + character.Value);
}
streamWriter.Close();
Console.ReadLine();
}
catch (Exception ex)
{
Console.WriteLine("Exception occured:" + ex.Message.ToString());
}
}
}
}
I would like to keep the basic functionality the same and mainly just change the sorted dictionary to a linked list. Is there a way to do this? Thanks.
c# linked-list sorteddictionary
|
show 4 more comments
up vote
-1
down vote
favorite
I have a program that reads an ASCII text file and counts the number of times each character appears in the file. It then creates an output file with each character and the number of times it appears. An example output is:
H(72) = 1
e(101) = 1
l(108) = 2
o(111) = 1
.(46) = 1
In my program, I use a sorted dictionary to store the characters. I want to use a Linked List instead to store the values but I am note sure the best way to go about this. My program is below:
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.IO;
using System.Text;
using System.Reflection;
//CharacterFrequency class
namespace ASCII
{
class CharacterFrequency
{
private char ch;
private int frequency;
public char Char
{
get { return ch; }
set { ch = value; }
}
public int Frequency
{
get { return frequency; }
set { frequency = value; }
}
}
class Counter
{
public string InputFileName = "example.txt";
public string OutputFileName = "example.txt";
//use a sorted dictionary to store values and keys of characters
public static SortedDictionary<char, ulong> Count(string stringToCount)
{
SortedDictionary<char, ulong> characterCount = new SortedDictionary<char, ulong>();
//loop through the sorted dictionary
foreach (var character in stringToCount)
{
if (!characterCount.ContainsKey(character))
{
characterCount.Add(character, 1);
}
else
{
characterCount[character]++;
}
}
return characterCount;
}
static void Main(string args)
{
CharacterFrequency charfreq = new CharacterFrequency();
Counter c = new Counter();
try
{
//gets input ASCII file
Console.WriteLine("Enter the input file path: ");
c.InputFileName = Console.ReadLine();
//gets output file path location
Console.WriteLine("Enter the output file path: ");
c.OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(c.OutputFileName);
string data = File.ReadAllText(c.InputFileName);
var count = Counter.Count(data);
foreach (var character in count)
{
streamWriter.WriteLine(character.Key + "(" + (int)character.Key + ")" + "t" + character.Value);
}
streamWriter.Close();
Console.ReadLine();
}
catch (Exception ex)
{
Console.WriteLine("Exception occured:" + ex.Message.ToString());
}
}
}
}
I would like to keep the basic functionality the same and mainly just change the sorted dictionary to a linked list. Is there a way to do this? Thanks.
c# linked-list sorteddictionary
2
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
1
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
1
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
1
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09
|
show 4 more comments
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I have a program that reads an ASCII text file and counts the number of times each character appears in the file. It then creates an output file with each character and the number of times it appears. An example output is:
H(72) = 1
e(101) = 1
l(108) = 2
o(111) = 1
.(46) = 1
In my program, I use a sorted dictionary to store the characters. I want to use a Linked List instead to store the values but I am note sure the best way to go about this. My program is below:
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.IO;
using System.Text;
using System.Reflection;
//CharacterFrequency class
namespace ASCII
{
class CharacterFrequency
{
private char ch;
private int frequency;
public char Char
{
get { return ch; }
set { ch = value; }
}
public int Frequency
{
get { return frequency; }
set { frequency = value; }
}
}
class Counter
{
public string InputFileName = "example.txt";
public string OutputFileName = "example.txt";
//use a sorted dictionary to store values and keys of characters
public static SortedDictionary<char, ulong> Count(string stringToCount)
{
SortedDictionary<char, ulong> characterCount = new SortedDictionary<char, ulong>();
//loop through the sorted dictionary
foreach (var character in stringToCount)
{
if (!characterCount.ContainsKey(character))
{
characterCount.Add(character, 1);
}
else
{
characterCount[character]++;
}
}
return characterCount;
}
static void Main(string args)
{
CharacterFrequency charfreq = new CharacterFrequency();
Counter c = new Counter();
try
{
//gets input ASCII file
Console.WriteLine("Enter the input file path: ");
c.InputFileName = Console.ReadLine();
//gets output file path location
Console.WriteLine("Enter the output file path: ");
c.OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(c.OutputFileName);
string data = File.ReadAllText(c.InputFileName);
var count = Counter.Count(data);
foreach (var character in count)
{
streamWriter.WriteLine(character.Key + "(" + (int)character.Key + ")" + "t" + character.Value);
}
streamWriter.Close();
Console.ReadLine();
}
catch (Exception ex)
{
Console.WriteLine("Exception occured:" + ex.Message.ToString());
}
}
}
}
I would like to keep the basic functionality the same and mainly just change the sorted dictionary to a linked list. Is there a way to do this? Thanks.
c# linked-list sorteddictionary
I have a program that reads an ASCII text file and counts the number of times each character appears in the file. It then creates an output file with each character and the number of times it appears. An example output is:
H(72) = 1
e(101) = 1
l(108) = 2
o(111) = 1
.(46) = 1
In my program, I use a sorted dictionary to store the characters. I want to use a Linked List instead to store the values but I am note sure the best way to go about this. My program is below:
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.IO;
using System.Text;
using System.Reflection;
//CharacterFrequency class
namespace ASCII
{
class CharacterFrequency
{
private char ch;
private int frequency;
public char Char
{
get { return ch; }
set { ch = value; }
}
public int Frequency
{
get { return frequency; }
set { frequency = value; }
}
}
class Counter
{
public string InputFileName = "example.txt";
public string OutputFileName = "example.txt";
//use a sorted dictionary to store values and keys of characters
public static SortedDictionary<char, ulong> Count(string stringToCount)
{
SortedDictionary<char, ulong> characterCount = new SortedDictionary<char, ulong>();
//loop through the sorted dictionary
foreach (var character in stringToCount)
{
if (!characterCount.ContainsKey(character))
{
characterCount.Add(character, 1);
}
else
{
characterCount[character]++;
}
}
return characterCount;
}
static void Main(string args)
{
CharacterFrequency charfreq = new CharacterFrequency();
Counter c = new Counter();
try
{
//gets input ASCII file
Console.WriteLine("Enter the input file path: ");
c.InputFileName = Console.ReadLine();
//gets output file path location
Console.WriteLine("Enter the output file path: ");
c.OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(c.OutputFileName);
string data = File.ReadAllText(c.InputFileName);
var count = Counter.Count(data);
foreach (var character in count)
{
streamWriter.WriteLine(character.Key + "(" + (int)character.Key + ")" + "t" + character.Value);
}
streamWriter.Close();
Console.ReadLine();
}
catch (Exception ex)
{
Console.WriteLine("Exception occured:" + ex.Message.ToString());
}
}
}
}
I would like to keep the basic functionality the same and mainly just change the sorted dictionary to a linked list. Is there a way to do this? Thanks.
c# linked-list sorteddictionary
c# linked-list sorteddictionary
edited Nov 9 at 17:02
Wai Ha Lee
5,639123662
5,639123662
asked Nov 9 at 15:30
Zane
33
33
2
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
1
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
1
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
1
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09
|
show 4 more comments
2
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
1
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
1
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
1
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09
2
2
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
1
1
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
1
1
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
1
1
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09
|
show 4 more comments
1 Answer
1
active
oldest
votes
up vote
-1
down vote
First you'll need to declare a class for your linked list, which has a Successor
or Next
property, and contains the other values you wish to retain.
public class LinkedListElement
{
public char Char { get; set; }
public ulong Count { get; set; }
public LinkedListElement Next { get; set; }
}
Then you'll need to order your SortedDictionary<char,ulong>
by it's Value
properties, and select each item out as a LinkedListElement
, assigning the Next
property of the parent as you go, which you could do like this...
LinkedListElement first = null;
LinkedListElement parent = null;
count.OrderByDescending(x => x.Value).ToList().ForEach(x =>
{
var element = new LinkedListElement { Char = x.Key, Count = x.Value };
if (parent == null)
{
first = element;
}
else
{
parent.Next = element;
}
parent = element;
});
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go beforeclass CharacterFrequency
and the logic can go aftervar count = Counter.Count(data);
. Then thefirst
variable will contain the root node of your linked list.
– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
First you'll need to declare a class for your linked list, which has a Successor
or Next
property, and contains the other values you wish to retain.
public class LinkedListElement
{
public char Char { get; set; }
public ulong Count { get; set; }
public LinkedListElement Next { get; set; }
}
Then you'll need to order your SortedDictionary<char,ulong>
by it's Value
properties, and select each item out as a LinkedListElement
, assigning the Next
property of the parent as you go, which you could do like this...
LinkedListElement first = null;
LinkedListElement parent = null;
count.OrderByDescending(x => x.Value).ToList().ForEach(x =>
{
var element = new LinkedListElement { Char = x.Key, Count = x.Value };
if (parent == null)
{
first = element;
}
else
{
parent.Next = element;
}
parent = element;
});
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go beforeclass CharacterFrequency
and the logic can go aftervar count = Counter.Count(data);
. Then thefirst
variable will contain the root node of your linked list.
– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
add a comment |
up vote
-1
down vote
First you'll need to declare a class for your linked list, which has a Successor
or Next
property, and contains the other values you wish to retain.
public class LinkedListElement
{
public char Char { get; set; }
public ulong Count { get; set; }
public LinkedListElement Next { get; set; }
}
Then you'll need to order your SortedDictionary<char,ulong>
by it's Value
properties, and select each item out as a LinkedListElement
, assigning the Next
property of the parent as you go, which you could do like this...
LinkedListElement first = null;
LinkedListElement parent = null;
count.OrderByDescending(x => x.Value).ToList().ForEach(x =>
{
var element = new LinkedListElement { Char = x.Key, Count = x.Value };
if (parent == null)
{
first = element;
}
else
{
parent.Next = element;
}
parent = element;
});
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go beforeclass CharacterFrequency
and the logic can go aftervar count = Counter.Count(data);
. Then thefirst
variable will contain the root node of your linked list.
– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
add a comment |
up vote
-1
down vote
up vote
-1
down vote
First you'll need to declare a class for your linked list, which has a Successor
or Next
property, and contains the other values you wish to retain.
public class LinkedListElement
{
public char Char { get; set; }
public ulong Count { get; set; }
public LinkedListElement Next { get; set; }
}
Then you'll need to order your SortedDictionary<char,ulong>
by it's Value
properties, and select each item out as a LinkedListElement
, assigning the Next
property of the parent as you go, which you could do like this...
LinkedListElement first = null;
LinkedListElement parent = null;
count.OrderByDescending(x => x.Value).ToList().ForEach(x =>
{
var element = new LinkedListElement { Char = x.Key, Count = x.Value };
if (parent == null)
{
first = element;
}
else
{
parent.Next = element;
}
parent = element;
});
First you'll need to declare a class for your linked list, which has a Successor
or Next
property, and contains the other values you wish to retain.
public class LinkedListElement
{
public char Char { get; set; }
public ulong Count { get; set; }
public LinkedListElement Next { get; set; }
}
Then you'll need to order your SortedDictionary<char,ulong>
by it's Value
properties, and select each item out as a LinkedListElement
, assigning the Next
property of the parent as you go, which you could do like this...
LinkedListElement first = null;
LinkedListElement parent = null;
count.OrderByDescending(x => x.Value).ToList().ForEach(x =>
{
var element = new LinkedListElement { Char = x.Key, Count = x.Value };
if (parent == null)
{
first = element;
}
else
{
parent.Next = element;
}
parent = element;
});
answered Nov 9 at 15:48


Creyke
45827
45827
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go beforeclass CharacterFrequency
and the logic can go aftervar count = Counter.Count(data);
. Then thefirst
variable will contain the root node of your linked list.
– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
add a comment |
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go beforeclass CharacterFrequency
and the logic can go aftervar count = Counter.Count(data);
. Then thefirst
variable will contain the root node of your linked list.
– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
Where should I put this code at?
– Zane
Nov 9 at 15:56
Where should I put this code at?
– Zane
Nov 9 at 15:56
The class can go before
class CharacterFrequency
and the logic can go after var count = Counter.Count(data);
. Then the first
variable will contain the root node of your linked list.– Creyke
Nov 9 at 16:04
The class can go before
class CharacterFrequency
and the logic can go after var count = Counter.Count(data);
. Then the first
variable will contain the root node of your linked list.– Creyke
Nov 9 at 16:04
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
This code shows how to make the dictionary into a linked list. If the goal is to not use a dictionary at all, the whole code needs to be reformed…
– dumetrulo
Nov 9 at 16:27
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
@dumetrulo could you should how to use without the sorted dictionary?
– Zane
Nov 9 at 16:36
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228679%2fsorted-dictionary-to-linked-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sEjdxIOcIYqmxF 7 yJeVEV7x wV Hw7wnX,Vr0Cwd 19c1V3D,jHPUmgyWEKHODUhCvcLl
2
Why do you want either of those classes? You could just use a dictionary.. it doesn't appear that want anything in any particular order here
– Dave
Nov 9 at 15:36
I do want it in order and for this program I need to use a linked list, not a sorted dictionary.
– Zane
Nov 9 at 15:43
1
Ok.. could you explain why you need to use a linked list? At least IMO for your requirements here, the sorted dictionary is perfect
– Dave
Nov 9 at 15:47
1
@Zane If it's for educational purposes, then why are you asking other people to do it for you instead of trying to do it yourself? Doing it yourself is how you learn, after all.
– Servy
Nov 9 at 17:06
1
@Zane What are you stuck on? You haven't showed literally anything about an attempt to create a linked list, or described any problem you've had with creating one.
– Servy
Nov 9 at 18:09