Symfony + JMSSerializer throw 500 - handleCircularReference
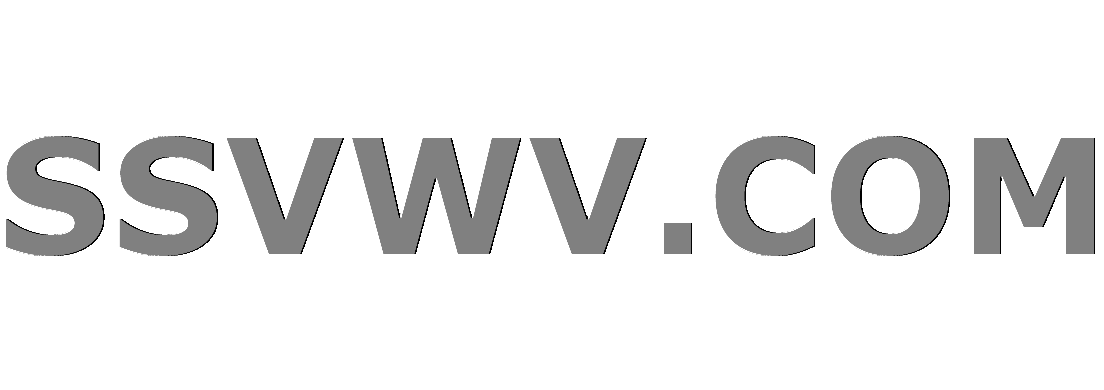
Multi tool use
up vote
1
down vote
favorite
I'm trying to use the JMSSerializer with Symfony to build a simple json api.
So i have 2 simple Entities (1 User can have many Cars, each Car belongs to one User):
class Car
{
/**
* @ORMId()
* @ORMGeneratedValue()
* @ORMColumn(type="integer")
*/
private $id;
/**
* @ORMColumn(type="string", length=255)
*/
private $name;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="cars")
* @ORMJoinColumn(nullable=false)
*/
private $user;
}
class User extends BaseUser
{
/**
* @ORMId
* @ORMColumn(type="integer")
* @ORMGeneratedValue(strategy="AUTO")
*/
protected $id;
/**
* @ORMOneToMany(targetEntity="AppEntityCar", mappedBy="user", orphanRemoval=true)
*/
private $cars;
}
Now i want to get all Cars
with their User
.
My Controller:
class CarController extends AbstractController
{
/**
* @param CarRepository $carRepository
*
* @Route("/", name="car_index", methods="GET")
*
* @return Response
*/
public function index(CarRepository $carRepository)
{
$cars = $carRepository->findAll();
$serializedEntity = $this->container->get('serializer')->serialize($cars, 'json');
return new Response($serializedEntity);
}
}
This will throw a 500 error:
A circular reference has been detected when serializing the object of
class "AppEntityCar" (configured limit: 1)
Ok, sounds clear. JMS is trying to get each car with the user, and go to the cars and user ....
So my question is: How to prevent this behaviour? I just want all cars with their user, and after this, the iteration should be stopped.
symfony symfony4 jmsserializerbundle jms-serializer
add a comment |
up vote
1
down vote
favorite
I'm trying to use the JMSSerializer with Symfony to build a simple json api.
So i have 2 simple Entities (1 User can have many Cars, each Car belongs to one User):
class Car
{
/**
* @ORMId()
* @ORMGeneratedValue()
* @ORMColumn(type="integer")
*/
private $id;
/**
* @ORMColumn(type="string", length=255)
*/
private $name;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="cars")
* @ORMJoinColumn(nullable=false)
*/
private $user;
}
class User extends BaseUser
{
/**
* @ORMId
* @ORMColumn(type="integer")
* @ORMGeneratedValue(strategy="AUTO")
*/
protected $id;
/**
* @ORMOneToMany(targetEntity="AppEntityCar", mappedBy="user", orphanRemoval=true)
*/
private $cars;
}
Now i want to get all Cars
with their User
.
My Controller:
class CarController extends AbstractController
{
/**
* @param CarRepository $carRepository
*
* @Route("/", name="car_index", methods="GET")
*
* @return Response
*/
public function index(CarRepository $carRepository)
{
$cars = $carRepository->findAll();
$serializedEntity = $this->container->get('serializer')->serialize($cars, 'json');
return new Response($serializedEntity);
}
}
This will throw a 500 error:
A circular reference has been detected when serializing the object of
class "AppEntityCar" (configured limit: 1)
Ok, sounds clear. JMS is trying to get each car with the user, and go to the cars and user ....
So my question is: How to prevent this behaviour? I just want all cars with their user, and after this, the iteration should be stopped.
symfony symfony4 jmsserializerbundle jms-serializer
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to use the JMSSerializer with Symfony to build a simple json api.
So i have 2 simple Entities (1 User can have many Cars, each Car belongs to one User):
class Car
{
/**
* @ORMId()
* @ORMGeneratedValue()
* @ORMColumn(type="integer")
*/
private $id;
/**
* @ORMColumn(type="string", length=255)
*/
private $name;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="cars")
* @ORMJoinColumn(nullable=false)
*/
private $user;
}
class User extends BaseUser
{
/**
* @ORMId
* @ORMColumn(type="integer")
* @ORMGeneratedValue(strategy="AUTO")
*/
protected $id;
/**
* @ORMOneToMany(targetEntity="AppEntityCar", mappedBy="user", orphanRemoval=true)
*/
private $cars;
}
Now i want to get all Cars
with their User
.
My Controller:
class CarController extends AbstractController
{
/**
* @param CarRepository $carRepository
*
* @Route("/", name="car_index", methods="GET")
*
* @return Response
*/
public function index(CarRepository $carRepository)
{
$cars = $carRepository->findAll();
$serializedEntity = $this->container->get('serializer')->serialize($cars, 'json');
return new Response($serializedEntity);
}
}
This will throw a 500 error:
A circular reference has been detected when serializing the object of
class "AppEntityCar" (configured limit: 1)
Ok, sounds clear. JMS is trying to get each car with the user, and go to the cars and user ....
So my question is: How to prevent this behaviour? I just want all cars with their user, and after this, the iteration should be stopped.
symfony symfony4 jmsserializerbundle jms-serializer
I'm trying to use the JMSSerializer with Symfony to build a simple json api.
So i have 2 simple Entities (1 User can have many Cars, each Car belongs to one User):
class Car
{
/**
* @ORMId()
* @ORMGeneratedValue()
* @ORMColumn(type="integer")
*/
private $id;
/**
* @ORMColumn(type="string", length=255)
*/
private $name;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="cars")
* @ORMJoinColumn(nullable=false)
*/
private $user;
}
class User extends BaseUser
{
/**
* @ORMId
* @ORMColumn(type="integer")
* @ORMGeneratedValue(strategy="AUTO")
*/
protected $id;
/**
* @ORMOneToMany(targetEntity="AppEntityCar", mappedBy="user", orphanRemoval=true)
*/
private $cars;
}
Now i want to get all Cars
with their User
.
My Controller:
class CarController extends AbstractController
{
/**
* @param CarRepository $carRepository
*
* @Route("/", name="car_index", methods="GET")
*
* @return Response
*/
public function index(CarRepository $carRepository)
{
$cars = $carRepository->findAll();
$serializedEntity = $this->container->get('serializer')->serialize($cars, 'json');
return new Response($serializedEntity);
}
}
This will throw a 500 error:
A circular reference has been detected when serializing the object of
class "AppEntityCar" (configured limit: 1)
Ok, sounds clear. JMS is trying to get each car with the user, and go to the cars and user ....
So my question is: How to prevent this behaviour? I just want all cars with their user, and after this, the iteration should be stopped.
symfony symfony4 jmsserializerbundle jms-serializer
symfony symfony4 jmsserializerbundle jms-serializer
asked Nov 8 at 10:16


rob
1,28351526
1,28351526
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You need to add max depth checks to prevent circular references.
This can be found in the documentation here
Basically you add the @MaxDepth(1)
annotation or configure max_depth
if you're using XML/YML configuration. Then serialize like this:
use JMSSerializerSerializationContext;
$serializer->serialize(
$data,
'json',
SerializationContext::create()->enableMaxDepthChecks()
);
Example Car
class with MaxDepth
annotation:
class Car
{
/**
* @JMSSerializerAnnotationMaxDepth(1)
*
* [..]
*/
private $user;
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You need to add max depth checks to prevent circular references.
This can be found in the documentation here
Basically you add the @MaxDepth(1)
annotation or configure max_depth
if you're using XML/YML configuration. Then serialize like this:
use JMSSerializerSerializationContext;
$serializer->serialize(
$data,
'json',
SerializationContext::create()->enableMaxDepthChecks()
);
Example Car
class with MaxDepth
annotation:
class Car
{
/**
* @JMSSerializerAnnotationMaxDepth(1)
*
* [..]
*/
private $user;
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
add a comment |
up vote
2
down vote
accepted
You need to add max depth checks to prevent circular references.
This can be found in the documentation here
Basically you add the @MaxDepth(1)
annotation or configure max_depth
if you're using XML/YML configuration. Then serialize like this:
use JMSSerializerSerializationContext;
$serializer->serialize(
$data,
'json',
SerializationContext::create()->enableMaxDepthChecks()
);
Example Car
class with MaxDepth
annotation:
class Car
{
/**
* @JMSSerializerAnnotationMaxDepth(1)
*
* [..]
*/
private $user;
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You need to add max depth checks to prevent circular references.
This can be found in the documentation here
Basically you add the @MaxDepth(1)
annotation or configure max_depth
if you're using XML/YML configuration. Then serialize like this:
use JMSSerializerSerializationContext;
$serializer->serialize(
$data,
'json',
SerializationContext::create()->enableMaxDepthChecks()
);
Example Car
class with MaxDepth
annotation:
class Car
{
/**
* @JMSSerializerAnnotationMaxDepth(1)
*
* [..]
*/
private $user;
You need to add max depth checks to prevent circular references.
This can be found in the documentation here
Basically you add the @MaxDepth(1)
annotation or configure max_depth
if you're using XML/YML configuration. Then serialize like this:
use JMSSerializerSerializationContext;
$serializer->serialize(
$data,
'json',
SerializationContext::create()->enableMaxDepthChecks()
);
Example Car
class with MaxDepth
annotation:
class Car
{
/**
* @JMSSerializerAnnotationMaxDepth(1)
*
* [..]
*/
private $user;
edited Nov 8 at 10:28
answered Nov 8 at 10:20


nifr
39.4k7104114
39.4k7104114
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
add a comment |
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
oh great. i didn't found this. thank you
– rob
Nov 8 at 12:24
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53205621%2fsymfony-jmsserializer-throw-500-handlecircularreference%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wA3f GJkO,O 944Xt,V5Fi6pMN7l9Q