PHP: array_filter for an object?
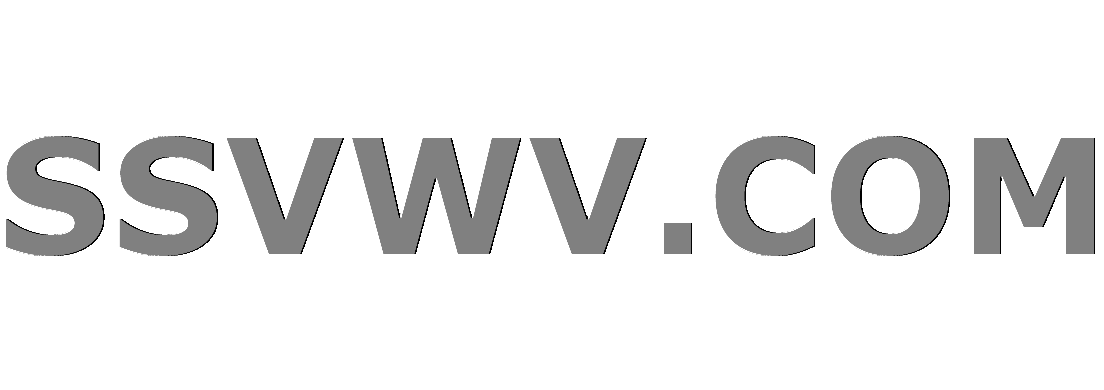
Multi tool use
up vote
1
down vote
favorite
I have an array that I need to filter for certain things, for example, I might only want records that have the day of the week as Friday. As far as I'm aware this has never worked but it's taking an object and using array_filter
on it. Can this work? Is there a better way or a way to do this on with object?
public function filterByDow($object)
{
$current_dow=5;
return array_values(array_filter($object, function ($array) use ($current_dow) {
$array = (array) $array;
if(!empty($array['day_id']) && $array['day_id'] > -1){
if($array['day_id'] != $current_dow){
return false;
}
}
return true;
}));
}
$object = $this->filterByDow($object);
Sample data might be like:
$object = (object) array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 4]);
php object laravel-5 array-filter
|
show 5 more comments
up vote
1
down vote
favorite
I have an array that I need to filter for certain things, for example, I might only want records that have the day of the week as Friday. As far as I'm aware this has never worked but it's taking an object and using array_filter
on it. Can this work? Is there a better way or a way to do this on with object?
public function filterByDow($object)
{
$current_dow=5;
return array_values(array_filter($object, function ($array) use ($current_dow) {
$array = (array) $array;
if(!empty($array['day_id']) && $array['day_id'] > -1){
if($array['day_id'] != $current_dow){
return false;
}
}
return true;
}));
}
$object = $this->filterByDow($object);
Sample data might be like:
$object = (object) array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 4]);
php object laravel-5 array-filter
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
1
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
1
If you have a laravel collection why the hell do you not use$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
1
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16
|
show 5 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have an array that I need to filter for certain things, for example, I might only want records that have the day of the week as Friday. As far as I'm aware this has never worked but it's taking an object and using array_filter
on it. Can this work? Is there a better way or a way to do this on with object?
public function filterByDow($object)
{
$current_dow=5;
return array_values(array_filter($object, function ($array) use ($current_dow) {
$array = (array) $array;
if(!empty($array['day_id']) && $array['day_id'] > -1){
if($array['day_id'] != $current_dow){
return false;
}
}
return true;
}));
}
$object = $this->filterByDow($object);
Sample data might be like:
$object = (object) array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 4]);
php object laravel-5 array-filter
I have an array that I need to filter for certain things, for example, I might only want records that have the day of the week as Friday. As far as I'm aware this has never worked but it's taking an object and using array_filter
on it. Can this work? Is there a better way or a way to do this on with object?
public function filterByDow($object)
{
$current_dow=5;
return array_values(array_filter($object, function ($array) use ($current_dow) {
$array = (array) $array;
if(!empty($array['day_id']) && $array['day_id'] > -1){
if($array['day_id'] != $current_dow){
return false;
}
}
return true;
}));
}
$object = $this->filterByDow($object);
Sample data might be like:
$object = (object) array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 4]);
php object laravel-5 array-filter
php object laravel-5 array-filter
edited Nov 9 at 15:58
asked Nov 9 at 15:44


sdexp
4092616
4092616
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
1
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
1
If you have a laravel collection why the hell do you not use$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
1
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16
|
show 5 more comments
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
1
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
1
If you have a laravel collection why the hell do you not use$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
1
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
1
1
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
1
1
If you have a laravel collection why the hell do you not use
$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
If you have a laravel collection why the hell do you not use
$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
1
1
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16
|
show 5 more comments
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
As from the comments the array is a laravel collection I guess the answer is:
$filtered = $collection->filter(function ($value, $key) {
return $value['day_id'] == 5;
});
https://laravel.com/docs/5.7/collections#method-filter
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
add a comment |
up vote
1
down vote
try this
<?php
$items = array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 5]);
function filterByDow($items, $dow = 5){
return array_filter($items, function($item) use ($dow) {
if($item['day_id'] == $dow){
return true;
}
});
}
$resultArr = filterByDow($items);
print_r($resultArr);
?>
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
add a comment |
up vote
1
down vote
Try to create a collection and implement methods for filtering in it.
class Offer
{
private $dayId;
public function getDayId()
{
return $this->dayId;
}
public function setDayId($dayId)
{
return $this->dayId = $dayId;
}
}
class OfferCollection
{
const FRIDAY = 5;
static $dayIds = [
self::FRIDAY => 'Friday'
];
private $offers = ;
public function addOffer(Offer $offer)
{
$this->offers = $offer;
}
public function getOffersByDay($dayId)
{
$offers = ;
if (in_array($dayId, self::$dayIds)) {
foreach ($this->offers as $offer) {
if ($offer->getDayId == $dayId) $offers = $offer;
}
}
return $offers;
}
}
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
As from the comments the array is a laravel collection I guess the answer is:
$filtered = $collection->filter(function ($value, $key) {
return $value['day_id'] == 5;
});
https://laravel.com/docs/5.7/collections#method-filter
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
add a comment |
up vote
1
down vote
accepted
As from the comments the array is a laravel collection I guess the answer is:
$filtered = $collection->filter(function ($value, $key) {
return $value['day_id'] == 5;
});
https://laravel.com/docs/5.7/collections#method-filter
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
As from the comments the array is a laravel collection I guess the answer is:
$filtered = $collection->filter(function ($value, $key) {
return $value['day_id'] == 5;
});
https://laravel.com/docs/5.7/collections#method-filter
As from the comments the array is a laravel collection I guess the answer is:
$filtered = $collection->filter(function ($value, $key) {
return $value['day_id'] == 5;
});
https://laravel.com/docs/5.7/collections#method-filter
answered Nov 9 at 16:19
Blackbam
4,696113871
4,696113871
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
add a comment |
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
This is exactly what I was looking for. Thanks.
– sdexp
Nov 9 at 16:20
add a comment |
up vote
1
down vote
try this
<?php
$items = array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 5]);
function filterByDow($items, $dow = 5){
return array_filter($items, function($item) use ($dow) {
if($item['day_id'] == $dow){
return true;
}
});
}
$resultArr = filterByDow($items);
print_r($resultArr);
?>
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
add a comment |
up vote
1
down vote
try this
<?php
$items = array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 5]);
function filterByDow($items, $dow = 5){
return array_filter($items, function($item) use ($dow) {
if($item['day_id'] == $dow){
return true;
}
});
}
$resultArr = filterByDow($items);
print_r($resultArr);
?>
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
add a comment |
up vote
1
down vote
up vote
1
down vote
try this
<?php
$items = array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 5]);
function filterByDow($items, $dow = 5){
return array_filter($items, function($item) use ($dow) {
if($item['day_id'] == $dow){
return true;
}
});
}
$resultArr = filterByDow($items);
print_r($resultArr);
?>
try this
<?php
$items = array(['id' => '1', 'day_id' => 3], ['id' => '2', 'day_id' => 5]);
function filterByDow($items, $dow = 5){
return array_filter($items, function($item) use ($dow) {
if($item['day_id'] == $dow){
return true;
}
});
}
$resultArr = filterByDow($items);
print_r($resultArr);
?>
answered Nov 9 at 16:12


Borhan
1381212
1381212
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
add a comment |
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
An object is passed to filterByDow therfore I do not know if this change in the code is allowed?
– Blackbam
Nov 9 at 16:14
add a comment |
up vote
1
down vote
Try to create a collection and implement methods for filtering in it.
class Offer
{
private $dayId;
public function getDayId()
{
return $this->dayId;
}
public function setDayId($dayId)
{
return $this->dayId = $dayId;
}
}
class OfferCollection
{
const FRIDAY = 5;
static $dayIds = [
self::FRIDAY => 'Friday'
];
private $offers = ;
public function addOffer(Offer $offer)
{
$this->offers = $offer;
}
public function getOffersByDay($dayId)
{
$offers = ;
if (in_array($dayId, self::$dayIds)) {
foreach ($this->offers as $offer) {
if ($offer->getDayId == $dayId) $offers = $offer;
}
}
return $offers;
}
}
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
add a comment |
up vote
1
down vote
Try to create a collection and implement methods for filtering in it.
class Offer
{
private $dayId;
public function getDayId()
{
return $this->dayId;
}
public function setDayId($dayId)
{
return $this->dayId = $dayId;
}
}
class OfferCollection
{
const FRIDAY = 5;
static $dayIds = [
self::FRIDAY => 'Friday'
];
private $offers = ;
public function addOffer(Offer $offer)
{
$this->offers = $offer;
}
public function getOffersByDay($dayId)
{
$offers = ;
if (in_array($dayId, self::$dayIds)) {
foreach ($this->offers as $offer) {
if ($offer->getDayId == $dayId) $offers = $offer;
}
}
return $offers;
}
}
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
add a comment |
up vote
1
down vote
up vote
1
down vote
Try to create a collection and implement methods for filtering in it.
class Offer
{
private $dayId;
public function getDayId()
{
return $this->dayId;
}
public function setDayId($dayId)
{
return $this->dayId = $dayId;
}
}
class OfferCollection
{
const FRIDAY = 5;
static $dayIds = [
self::FRIDAY => 'Friday'
];
private $offers = ;
public function addOffer(Offer $offer)
{
$this->offers = $offer;
}
public function getOffersByDay($dayId)
{
$offers = ;
if (in_array($dayId, self::$dayIds)) {
foreach ($this->offers as $offer) {
if ($offer->getDayId == $dayId) $offers = $offer;
}
}
return $offers;
}
}
Try to create a collection and implement methods for filtering in it.
class Offer
{
private $dayId;
public function getDayId()
{
return $this->dayId;
}
public function setDayId($dayId)
{
return $this->dayId = $dayId;
}
}
class OfferCollection
{
const FRIDAY = 5;
static $dayIds = [
self::FRIDAY => 'Friday'
];
private $offers = ;
public function addOffer(Offer $offer)
{
$this->offers = $offer;
}
public function getOffersByDay($dayId)
{
$offers = ;
if (in_array($dayId, self::$dayIds)) {
foreach ($this->offers as $offer) {
if ($offer->getDayId == $dayId) $offers = $offer;
}
}
return $offers;
}
}
answered Nov 9 at 16:18


Yaroslaw
684
684
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
add a comment |
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Nice solution if it is not already existing :-)
– Blackbam
Nov 9 at 16:21
Already exists:)
– Yaroslaw
Nov 9 at 16:23
Already exists:)
– Yaroslaw
Nov 9 at 16:23
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228919%2fphp-array-filter-for-an-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XJX8FRkftfkTdHRP0hMJTRLgcM0YQBscL,iS8GbX,InIq 18JFPnIPw0
can u provide a sample data, so that i can simulate it in fiddler?
– Borhan
Nov 9 at 15:52
Sure. I've edited the question. thank you.
– sdexp
Nov 9 at 15:58
1
What exactly does not work with the given code? Why do you need to cast the inner array into an object?
– Nico Haase
Nov 9 at 15:59
1
If you have a laravel collection why the hell do you not use
$filtered = $collection->filter(function ($value, $key) { return $value == 5; });
– Blackbam
Nov 9 at 16:16
1
laravel.com/docs/5.7/collections#method-filter :-) ?
– Blackbam
Nov 9 at 16:16