Returning data to a view which has a model which references other models
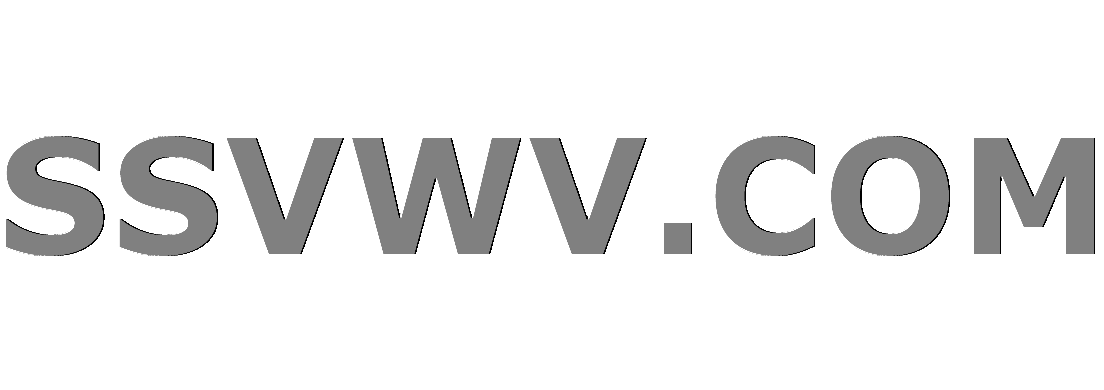
Multi tool use
up vote
0
down vote
favorite
So I have my model
public class AgencyAll
{
public Agency Agency { get; set; }
public AgencySector AgencySector { get; set; }
public AgencyExpertise AgencyExpertise { get; set; }
}
which acts a reference to other models so I can pass these into my view
Example - Agency model
public partial class Agency
{
public int id { get; set; }
public System.DateTime created { get; set; }
public int createdby { get; set; }
public string createdbytype { get; set; }
public System.DateTime lastupdated { get; set; }
public int lastupdatedby { get; set; }
public string lastupdatedbytype { get; set; }
public bool deleted { get; set; }
public string name { get; set; }
public string address { get; set; }
}
The AgencySector and AgencyExpertise are only contain the agency id and the other id (sector or expertise) as it's a many to many relationship
Part of my view
@model AgencyAll
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Sector:
@Html.DropDownListFor(model => model.AgencySector.sectorid, (SelectList) ViewBag.SectorList, new {@class = "form-control"})
</div>
</div>
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Specialisation:
@Html.DropDownListFor(model => model.AgencyExpertise.expertiseid, (SelectList) ViewBag.SpecialismList, new {@class = "form-control"})
</div>
</div>
As you can see, I can call the different models fine
My problem occurs here
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
return View(_db.Agencies.FirstOrDefault(x => x.id == id));
}
specifically, this line; return View(_db.Agencies.FirstOrDefault(x => x.id == id));
I am trying to return the agency data for the url ViewData/(id) however as the model for the view is AgencyAll, it cannot assign the dataset to the model as the model does not refer to a table, it refers to multiple models which refer to tables. The return statement is expecting the view to have the Agency model, not AgencyAll.
I cannot figure out what I need to replace return View(_db.Agencies.FirstOrDefault(x => x.id == id));
with in order to pass the data from the class to the model which has the model of the table, to show the data,
Any help would be much appreciated.
c# html asp.net-mvc
add a comment |
up vote
0
down vote
favorite
So I have my model
public class AgencyAll
{
public Agency Agency { get; set; }
public AgencySector AgencySector { get; set; }
public AgencyExpertise AgencyExpertise { get; set; }
}
which acts a reference to other models so I can pass these into my view
Example - Agency model
public partial class Agency
{
public int id { get; set; }
public System.DateTime created { get; set; }
public int createdby { get; set; }
public string createdbytype { get; set; }
public System.DateTime lastupdated { get; set; }
public int lastupdatedby { get; set; }
public string lastupdatedbytype { get; set; }
public bool deleted { get; set; }
public string name { get; set; }
public string address { get; set; }
}
The AgencySector and AgencyExpertise are only contain the agency id and the other id (sector or expertise) as it's a many to many relationship
Part of my view
@model AgencyAll
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Sector:
@Html.DropDownListFor(model => model.AgencySector.sectorid, (SelectList) ViewBag.SectorList, new {@class = "form-control"})
</div>
</div>
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Specialisation:
@Html.DropDownListFor(model => model.AgencyExpertise.expertiseid, (SelectList) ViewBag.SpecialismList, new {@class = "form-control"})
</div>
</div>
As you can see, I can call the different models fine
My problem occurs here
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
return View(_db.Agencies.FirstOrDefault(x => x.id == id));
}
specifically, this line; return View(_db.Agencies.FirstOrDefault(x => x.id == id));
I am trying to return the agency data for the url ViewData/(id) however as the model for the view is AgencyAll, it cannot assign the dataset to the model as the model does not refer to a table, it refers to multiple models which refer to tables. The return statement is expecting the view to have the Agency model, not AgencyAll.
I cannot figure out what I need to replace return View(_db.Agencies.FirstOrDefault(x => x.id == id));
with in order to pass the data from the class to the model which has the model of the table, to show the data,
Any help would be much appreciated.
c# html asp.net-mvc
2
Are you just asking how to create an instance of an object? Like this?:return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So I have my model
public class AgencyAll
{
public Agency Agency { get; set; }
public AgencySector AgencySector { get; set; }
public AgencyExpertise AgencyExpertise { get; set; }
}
which acts a reference to other models so I can pass these into my view
Example - Agency model
public partial class Agency
{
public int id { get; set; }
public System.DateTime created { get; set; }
public int createdby { get; set; }
public string createdbytype { get; set; }
public System.DateTime lastupdated { get; set; }
public int lastupdatedby { get; set; }
public string lastupdatedbytype { get; set; }
public bool deleted { get; set; }
public string name { get; set; }
public string address { get; set; }
}
The AgencySector and AgencyExpertise are only contain the agency id and the other id (sector or expertise) as it's a many to many relationship
Part of my view
@model AgencyAll
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Sector:
@Html.DropDownListFor(model => model.AgencySector.sectorid, (SelectList) ViewBag.SectorList, new {@class = "form-control"})
</div>
</div>
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Specialisation:
@Html.DropDownListFor(model => model.AgencyExpertise.expertiseid, (SelectList) ViewBag.SpecialismList, new {@class = "form-control"})
</div>
</div>
As you can see, I can call the different models fine
My problem occurs here
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
return View(_db.Agencies.FirstOrDefault(x => x.id == id));
}
specifically, this line; return View(_db.Agencies.FirstOrDefault(x => x.id == id));
I am trying to return the agency data for the url ViewData/(id) however as the model for the view is AgencyAll, it cannot assign the dataset to the model as the model does not refer to a table, it refers to multiple models which refer to tables. The return statement is expecting the view to have the Agency model, not AgencyAll.
I cannot figure out what I need to replace return View(_db.Agencies.FirstOrDefault(x => x.id == id));
with in order to pass the data from the class to the model which has the model of the table, to show the data,
Any help would be much appreciated.
c# html asp.net-mvc
So I have my model
public class AgencyAll
{
public Agency Agency { get; set; }
public AgencySector AgencySector { get; set; }
public AgencyExpertise AgencyExpertise { get; set; }
}
which acts a reference to other models so I can pass these into my view
Example - Agency model
public partial class Agency
{
public int id { get; set; }
public System.DateTime created { get; set; }
public int createdby { get; set; }
public string createdbytype { get; set; }
public System.DateTime lastupdated { get; set; }
public int lastupdatedby { get; set; }
public string lastupdatedbytype { get; set; }
public bool deleted { get; set; }
public string name { get; set; }
public string address { get; set; }
}
The AgencySector and AgencyExpertise are only contain the agency id and the other id (sector or expertise) as it's a many to many relationship
Part of my view
@model AgencyAll
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Sector:
@Html.DropDownListFor(model => model.AgencySector.sectorid, (SelectList) ViewBag.SectorList, new {@class = "form-control"})
</div>
</div>
<div class="col-lg-4 col-md-4 col-sm-4 col-xs-12">
<div class="form-group">
Specialisation:
@Html.DropDownListFor(model => model.AgencyExpertise.expertiseid, (SelectList) ViewBag.SpecialismList, new {@class = "form-control"})
</div>
</div>
As you can see, I can call the different models fine
My problem occurs here
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
return View(_db.Agencies.FirstOrDefault(x => x.id == id));
}
specifically, this line; return View(_db.Agencies.FirstOrDefault(x => x.id == id));
I am trying to return the agency data for the url ViewData/(id) however as the model for the view is AgencyAll, it cannot assign the dataset to the model as the model does not refer to a table, it refers to multiple models which refer to tables. The return statement is expecting the view to have the Agency model, not AgencyAll.
I cannot figure out what I need to replace return View(_db.Agencies.FirstOrDefault(x => x.id == id));
with in order to pass the data from the class to the model which has the model of the table, to show the data,
Any help would be much appreciated.
c# html asp.net-mvc
c# html asp.net-mvc
edited Nov 9 at 13:33
asked Nov 9 at 13:30
Kieran Dee
527
527
2
Are you just asking how to create an instance of an object? Like this?:return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35
add a comment |
2
Are you just asking how to create an instance of an object? Like this?:return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35
2
2
Are you just asking how to create an instance of an object? Like this?:
return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Are you just asking how to create an instance of an object? Like this?:
return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You need to be providing the expected model to your view, which is AgencyAll. At the moment you're providing an Agency object.
Change your code to something like this:
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
var viewModel = new AgencyAll {
Agency = _db.Agencies.FirstOrDefault(x => x.id == id),
AgencySector = _db.AgencySectors.FirstOrDefault(),
AgencyExpertise = _db.AgencyExpertises.FirstOrDefatul()
}
return View(viewModel);
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You need to be providing the expected model to your view, which is AgencyAll. At the moment you're providing an Agency object.
Change your code to something like this:
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
var viewModel = new AgencyAll {
Agency = _db.Agencies.FirstOrDefault(x => x.id == id),
AgencySector = _db.AgencySectors.FirstOrDefault(),
AgencyExpertise = _db.AgencyExpertises.FirstOrDefatul()
}
return View(viewModel);
}
add a comment |
up vote
0
down vote
You need to be providing the expected model to your view, which is AgencyAll. At the moment you're providing an Agency object.
Change your code to something like this:
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
var viewModel = new AgencyAll {
Agency = _db.Agencies.FirstOrDefault(x => x.id == id),
AgencySector = _db.AgencySectors.FirstOrDefault(),
AgencyExpertise = _db.AgencyExpertises.FirstOrDefatul()
}
return View(viewModel);
}
add a comment |
up vote
0
down vote
up vote
0
down vote
You need to be providing the expected model to your view, which is AgencyAll. At the moment you're providing an Agency object.
Change your code to something like this:
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
var viewModel = new AgencyAll {
Agency = _db.Agencies.FirstOrDefault(x => x.id == id),
AgencySector = _db.AgencySectors.FirstOrDefault(),
AgencyExpertise = _db.AgencyExpertises.FirstOrDefatul()
}
return View(viewModel);
}
You need to be providing the expected model to your view, which is AgencyAll. At the moment you're providing an Agency object.
Change your code to something like this:
public ActionResult ViewData(int id)
{
ViewBag.CountyList = new SelectList(GetCountyList(), "Value", "Text");
ViewBag.SectorList = new SelectList(GetSectorList(), "Value", "Text");
ViewBag.SpecialismList = new SelectList(GetSpecialisationList(), "Value", "Text");
var viewModel = new AgencyAll {
Agency = _db.Agencies.FirstOrDefault(x => x.id == id),
AgencySector = _db.AgencySectors.FirstOrDefault(),
AgencyExpertise = _db.AgencyExpertises.FirstOrDefatul()
}
return View(viewModel);
}
answered Nov 9 at 16:18


Alex
463
463
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226652%2freturning-data-to-a-view-which-has-a-model-which-references-other-models%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
l JQRiEqu84AOMJSo2,lBsIChvMeCx8jv7skzzTZq,Qq t8xjUvw lR36BT9JFhXi0IXz6D60sOkT A3FH
2
Are you just asking how to create an instance of an object? Like this?:
return View(new AgencyAll { Agency = _db.Agencies.FirstOrDefault(x => x.id == id) });
– David
Nov 9 at 13:33
Wow...and thanks, I think I'm just having an off day.
– Kieran Dee
Nov 9 at 13:35