Calculate balances in transactions table where users send money to each other
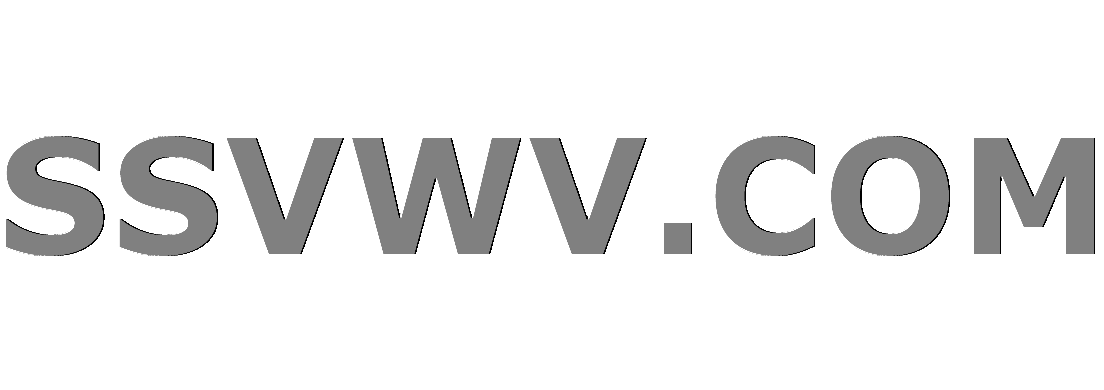
Multi tool use
up vote
1
down vote
favorite
I have a transaction table set up like this:
-- Transactions table
+----+---------+-------+------------------+--------+-----------+
| id | from_id | to_id | transaction_type | amount | card_type |
+----+---------+-------+------------------+--------+-----------+
| 1 | 1 | 1 | deposit | 90 | debit |
| 2 | 1 | 2 | transfer | -60 | debit |
| 3 | 2 | 2 | deposit | 10 | debit |
| 4 | 2 | 2 | deposit | 20 | credit |
+----+---------+-------+------------------+--------+-----------+
If i deposit it should show a positive value to show that money was added to my account, but if i do a transfer it should use a negative balance to show that money was removed from my account. The issue is, I can't think of a query that would add the money to the user 2 account from the transfer of user 1 to produce a view like this (based on card type):
-- Debit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 30 |
| 2 | 70 |
+---------+---------+
-- Credit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 0 |
| 2 | 20 |
+---------+---------+
I know you can't add money to a credit account but just forget that logic for now.
mysql sql
add a comment |
up vote
1
down vote
favorite
I have a transaction table set up like this:
-- Transactions table
+----+---------+-------+------------------+--------+-----------+
| id | from_id | to_id | transaction_type | amount | card_type |
+----+---------+-------+------------------+--------+-----------+
| 1 | 1 | 1 | deposit | 90 | debit |
| 2 | 1 | 2 | transfer | -60 | debit |
| 3 | 2 | 2 | deposit | 10 | debit |
| 4 | 2 | 2 | deposit | 20 | credit |
+----+---------+-------+------------------+--------+-----------+
If i deposit it should show a positive value to show that money was added to my account, but if i do a transfer it should use a negative balance to show that money was removed from my account. The issue is, I can't think of a query that would add the money to the user 2 account from the transfer of user 1 to produce a view like this (based on card type):
-- Debit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 30 |
| 2 | 70 |
+---------+---------+
-- Credit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 0 |
| 2 | 20 |
+---------+---------+
I know you can't add money to a credit account but just forget that logic for now.
mysql sql
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a transaction table set up like this:
-- Transactions table
+----+---------+-------+------------------+--------+-----------+
| id | from_id | to_id | transaction_type | amount | card_type |
+----+---------+-------+------------------+--------+-----------+
| 1 | 1 | 1 | deposit | 90 | debit |
| 2 | 1 | 2 | transfer | -60 | debit |
| 3 | 2 | 2 | deposit | 10 | debit |
| 4 | 2 | 2 | deposit | 20 | credit |
+----+---------+-------+------------------+--------+-----------+
If i deposit it should show a positive value to show that money was added to my account, but if i do a transfer it should use a negative balance to show that money was removed from my account. The issue is, I can't think of a query that would add the money to the user 2 account from the transfer of user 1 to produce a view like this (based on card type):
-- Debit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 30 |
| 2 | 70 |
+---------+---------+
-- Credit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 0 |
| 2 | 20 |
+---------+---------+
I know you can't add money to a credit account but just forget that logic for now.
mysql sql
I have a transaction table set up like this:
-- Transactions table
+----+---------+-------+------------------+--------+-----------+
| id | from_id | to_id | transaction_type | amount | card_type |
+----+---------+-------+------------------+--------+-----------+
| 1 | 1 | 1 | deposit | 90 | debit |
| 2 | 1 | 2 | transfer | -60 | debit |
| 3 | 2 | 2 | deposit | 10 | debit |
| 4 | 2 | 2 | deposit | 20 | credit |
+----+---------+-------+------------------+--------+-----------+
If i deposit it should show a positive value to show that money was added to my account, but if i do a transfer it should use a negative balance to show that money was removed from my account. The issue is, I can't think of a query that would add the money to the user 2 account from the transfer of user 1 to produce a view like this (based on card type):
-- Debit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 30 |
| 2 | 70 |
+---------+---------+
-- Credit Balance Table
+---------+---------+
| user_id | balance |
+---------+---------+
| 1 | 0 |
| 2 | 20 |
+---------+---------+
I know you can't add money to a credit account but just forget that logic for now.
mysql sql
mysql sql
edited Nov 8 at 11:09


Strawberry
25.6k83149
25.6k83149
asked Nov 8 at 11:05


chinloyal
36115
36115
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
For debit
, you can simply do conditional aggregation:
SELECT
all_users.user_id,
SUM (CASE
WHEN t.transaction_type = 'deposit' AND all_users.user_id = t.from_id
THEN ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.from_id
THEN -ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.to_id
THEN ABS(t.amount)
ELSE 0
END
) AS balance
FROM transactions AS t
JOIN (
SELECT from_id AS user_id FROM transactions
UNION
SELECT to_id FROM transactions
) AS all_users
ON t.from_id = all_users.user_id OR
t.to_id = all_users.user_id
WHERE t.card_type = 'debit'
GROUP BY all_users.user_id
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
For debit
, you can simply do conditional aggregation:
SELECT
all_users.user_id,
SUM (CASE
WHEN t.transaction_type = 'deposit' AND all_users.user_id = t.from_id
THEN ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.from_id
THEN -ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.to_id
THEN ABS(t.amount)
ELSE 0
END
) AS balance
FROM transactions AS t
JOIN (
SELECT from_id AS user_id FROM transactions
UNION
SELECT to_id FROM transactions
) AS all_users
ON t.from_id = all_users.user_id OR
t.to_id = all_users.user_id
WHERE t.card_type = 'debit'
GROUP BY all_users.user_id
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
add a comment |
up vote
1
down vote
accepted
For debit
, you can simply do conditional aggregation:
SELECT
all_users.user_id,
SUM (CASE
WHEN t.transaction_type = 'deposit' AND all_users.user_id = t.from_id
THEN ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.from_id
THEN -ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.to_id
THEN ABS(t.amount)
ELSE 0
END
) AS balance
FROM transactions AS t
JOIN (
SELECT from_id AS user_id FROM transactions
UNION
SELECT to_id FROM transactions
) AS all_users
ON t.from_id = all_users.user_id OR
t.to_id = all_users.user_id
WHERE t.card_type = 'debit'
GROUP BY all_users.user_id
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
For debit
, you can simply do conditional aggregation:
SELECT
all_users.user_id,
SUM (CASE
WHEN t.transaction_type = 'deposit' AND all_users.user_id = t.from_id
THEN ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.from_id
THEN -ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.to_id
THEN ABS(t.amount)
ELSE 0
END
) AS balance
FROM transactions AS t
JOIN (
SELECT from_id AS user_id FROM transactions
UNION
SELECT to_id FROM transactions
) AS all_users
ON t.from_id = all_users.user_id OR
t.to_id = all_users.user_id
WHERE t.card_type = 'debit'
GROUP BY all_users.user_id
For debit
, you can simply do conditional aggregation:
SELECT
all_users.user_id,
SUM (CASE
WHEN t.transaction_type = 'deposit' AND all_users.user_id = t.from_id
THEN ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.from_id
THEN -ABS(t.amount)
WHEN t.transaction_type = 'transfer' AND all_users.user_id = t.to_id
THEN ABS(t.amount)
ELSE 0
END
) AS balance
FROM transactions AS t
JOIN (
SELECT from_id AS user_id FROM transactions
UNION
SELECT to_id FROM transactions
) AS all_users
ON t.from_id = all_users.user_id OR
t.to_id = all_users.user_id
WHERE t.card_type = 'debit'
GROUP BY all_users.user_id
answered Nov 8 at 11:15


Madhur Bhaiya
15.5k52136
15.5k52136
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
add a comment |
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
1
1
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
:) Gotta love stackoverflow, thank you.
– chinloyal
Nov 8 at 11:27
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
@chinloyal loved the question. bit tricky +1. Hope u understood what I am trying to do, else I can add explanation.
– Madhur Bhaiya
Nov 8 at 11:46
1
1
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
I'm not an expert at sql but I do understand what your doing, it's a very good solution. @MadhurBhaiya
– chinloyal
Nov 8 at 11:51
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206470%2fcalculate-balances-in-transactions-table-where-users-send-money-to-each-other%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n uCwelClL6c2