Saving Inventory with Dependency Injection
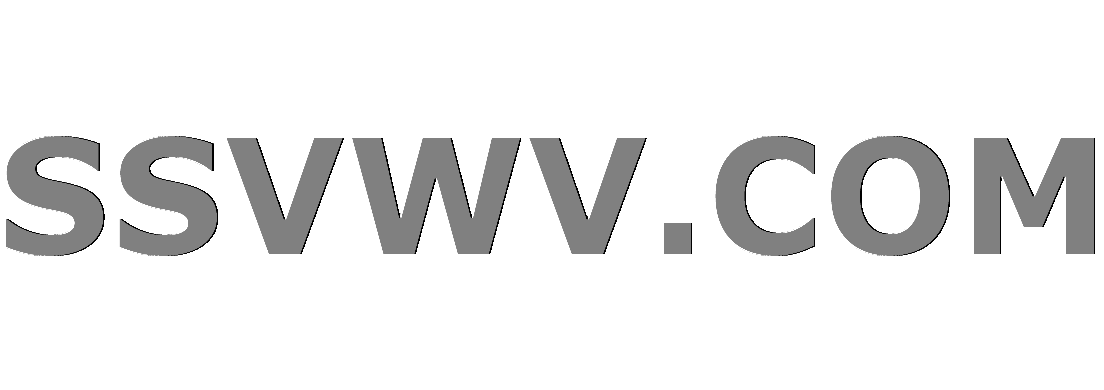
Multi tool use
up vote
0
down vote
favorite
I'm trying to open someone's Inventory in a different class than were I created the Inventory. I don't want to use statics so I tried a workaround with Dependency Injections. I made an ArrayList inventories, after that I add the created Inventory to the ArrayList. Here's the problem though, on reload the ArrayList gets wiped (of course), would there be a solution to still keep the Inventory in life without having it to be written down in a config or database. Because now players won't be able to open this created Inventory anymore after a reload, I really wonder what other plugins use to counter this problem (if they don't use statics of course ;)).
Here's some code I use:
private final Inventory inv;
private ArrayList<Inventory> inventories;
private KitPvP kitPvP;
private String name;
private Material material;
public KitManager(KitPvP kitPvP, String name, Material material, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.name = ChatColor.translateAlternateColorCodes('&', name);
this.material = material;
this.inventories = inventories;
this.inv = Bukkit.createInventory(null, 9, this.name);
}
public void createKit(Player p) {
ItemStack itemStack = new ItemStack(this.material);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(this.name);
itemStack.setItemMeta(itemMeta);
p.getInventory().addItem(itemStack);
this.inventories.add(this.inv);
p.openInventory(this.inv);
}
Event class
private KitPvP kitPvP;
private ArrayList<Inventory> inventories;
public InteractListener(KitPvP kitPvP, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.inventories = inventories;
}
@EventHandler
public void onPlayerInteract(PlayerInteractEvent e) {
Player p = e.getPlayer();
p.sendMessage(this.inventories.toString());
}
java dependency-injection bukkit
add a comment |
up vote
0
down vote
favorite
I'm trying to open someone's Inventory in a different class than were I created the Inventory. I don't want to use statics so I tried a workaround with Dependency Injections. I made an ArrayList inventories, after that I add the created Inventory to the ArrayList. Here's the problem though, on reload the ArrayList gets wiped (of course), would there be a solution to still keep the Inventory in life without having it to be written down in a config or database. Because now players won't be able to open this created Inventory anymore after a reload, I really wonder what other plugins use to counter this problem (if they don't use statics of course ;)).
Here's some code I use:
private final Inventory inv;
private ArrayList<Inventory> inventories;
private KitPvP kitPvP;
private String name;
private Material material;
public KitManager(KitPvP kitPvP, String name, Material material, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.name = ChatColor.translateAlternateColorCodes('&', name);
this.material = material;
this.inventories = inventories;
this.inv = Bukkit.createInventory(null, 9, this.name);
}
public void createKit(Player p) {
ItemStack itemStack = new ItemStack(this.material);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(this.name);
itemStack.setItemMeta(itemMeta);
p.getInventory().addItem(itemStack);
this.inventories.add(this.inv);
p.openInventory(this.inv);
}
Event class
private KitPvP kitPvP;
private ArrayList<Inventory> inventories;
public InteractListener(KitPvP kitPvP, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.inventories = inventories;
}
@EventHandler
public void onPlayerInteract(PlayerInteractEvent e) {
Player p = e.getPlayer();
p.sendMessage(this.inventories.toString());
}
java dependency-injection bukkit
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to open someone's Inventory in a different class than were I created the Inventory. I don't want to use statics so I tried a workaround with Dependency Injections. I made an ArrayList inventories, after that I add the created Inventory to the ArrayList. Here's the problem though, on reload the ArrayList gets wiped (of course), would there be a solution to still keep the Inventory in life without having it to be written down in a config or database. Because now players won't be able to open this created Inventory anymore after a reload, I really wonder what other plugins use to counter this problem (if they don't use statics of course ;)).
Here's some code I use:
private final Inventory inv;
private ArrayList<Inventory> inventories;
private KitPvP kitPvP;
private String name;
private Material material;
public KitManager(KitPvP kitPvP, String name, Material material, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.name = ChatColor.translateAlternateColorCodes('&', name);
this.material = material;
this.inventories = inventories;
this.inv = Bukkit.createInventory(null, 9, this.name);
}
public void createKit(Player p) {
ItemStack itemStack = new ItemStack(this.material);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(this.name);
itemStack.setItemMeta(itemMeta);
p.getInventory().addItem(itemStack);
this.inventories.add(this.inv);
p.openInventory(this.inv);
}
Event class
private KitPvP kitPvP;
private ArrayList<Inventory> inventories;
public InteractListener(KitPvP kitPvP, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.inventories = inventories;
}
@EventHandler
public void onPlayerInteract(PlayerInteractEvent e) {
Player p = e.getPlayer();
p.sendMessage(this.inventories.toString());
}
java dependency-injection bukkit
I'm trying to open someone's Inventory in a different class than were I created the Inventory. I don't want to use statics so I tried a workaround with Dependency Injections. I made an ArrayList inventories, after that I add the created Inventory to the ArrayList. Here's the problem though, on reload the ArrayList gets wiped (of course), would there be a solution to still keep the Inventory in life without having it to be written down in a config or database. Because now players won't be able to open this created Inventory anymore after a reload, I really wonder what other plugins use to counter this problem (if they don't use statics of course ;)).
Here's some code I use:
private final Inventory inv;
private ArrayList<Inventory> inventories;
private KitPvP kitPvP;
private String name;
private Material material;
public KitManager(KitPvP kitPvP, String name, Material material, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.name = ChatColor.translateAlternateColorCodes('&', name);
this.material = material;
this.inventories = inventories;
this.inv = Bukkit.createInventory(null, 9, this.name);
}
public void createKit(Player p) {
ItemStack itemStack = new ItemStack(this.material);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(this.name);
itemStack.setItemMeta(itemMeta);
p.getInventory().addItem(itemStack);
this.inventories.add(this.inv);
p.openInventory(this.inv);
}
Event class
private KitPvP kitPvP;
private ArrayList<Inventory> inventories;
public InteractListener(KitPvP kitPvP, ArrayList<Inventory> inventories) {
this.kitPvP = kitPvP;
this.inventories = inventories;
}
@EventHandler
public void onPlayerInteract(PlayerInteractEvent e) {
Player p = e.getPlayer();
p.sendMessage(this.inventories.toString());
}
java dependency-injection bukkit
java dependency-injection bukkit
asked Nov 8 at 10:59
Ezrab_
207
207
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29
add a comment |
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206366%2fsaving-inventory-with-dependency-injection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3AhCQ QUDfleEgwFavfB8 6xmWEHO,mdWOBh82
You could always use the Cache to store it there. When someone reload then in the init process you can set the Inventory back. Dependency Injection will not help you because every new object which will be injected will not contain the old data you want.
– Σωτήρης Ραφαήλ
Nov 8 at 11:29