MongoDB: active connections are accumulating, what should I do?
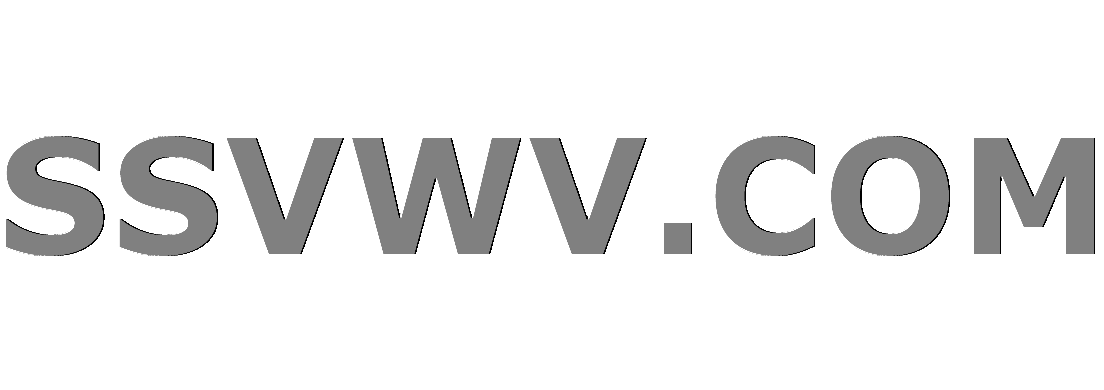
Multi tool use
up vote
0
down vote
favorite
I have a site, implemented on NodeJS, base MongoDB, Mongoose plugin. Recently, the site began to fall about once a day. I recently found out that this is due to the lack of memory, which is due to the fact that active connections are being accumulated (db.serverStatus (). Connections.current
). Perhaps this is not related, but I have a script on NodeJS, which is executed by crown every minute. It checks if there is a post with the current date in the documents. But I close the mongoose connection there, I don’t know what could be the problem. Actually this file contents:
process.env.NODE_TLS_REJECT_UNAUTHORIZED = 0;
const { new_time } = require("lib/functions");
const push = require("lib/push");
const apiCallback = require("middleware/socket/apiCallback");
const mongoose = require("lib/mongoose");
const User = require("models/User");
const Post = require("models/Post");
(async () => {
let currentPost = await Post.findCurrent(1);
if (currentPost) {
await currentPost.setPublished(1);
await apiCallback.call({
roomName: "index",
event : "posts.new",
data : {
post: {
id: currentPost._id.toString()
}
}
});
await push.sendAll({
// unnecessary data
});
}
await mongoose.connection.close();
process.exit(0);
})();
app.js:
const path = require("path");
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const expressSession = require("express-session");
const MongoStore = require("connect-mongo")(expressSession);
const conf = require("conf");
const mongoose = require("lib/mongoose");
const expressSessionConfig = conf.get("session");
expressSessionConfig.cookie.expires = new Date(new Date().getTime() + 60 * 60 * 24 * 30 * 1000);
expressSessionConfig.store = new MongoStore({
mongooseConnection: mongoose.connection
});
const templateDir = path.join(__dirname, conf.get("template_dir"));
app.engine("ejs", require("ejs-locals"));
app.set("views", templateDir);
app.set("view engine", "ejs")
app.use(express.static("frontend"));
app.use(cookieParser());
app.use(expressSession(expressSessionConfig));
app.use(bodyParser.urlencoded({
extended: true
}));
require("routes")(app);
app.listen(conf.get("app_port"));
app.io.js (socket server on socket.io):
const fs = require("fs");
const path = require("path");
const app = require("express")();
const bodyParser = require("body-parser");
const apiCallback = require("middleware/socket/apiCallback");
const conf = require("conf");
const sslPath = conf.get("sslPath");
const sslOptions = {
key : fs.readFileSync(path.join(sslPath, "key.key")),
cert: fs.readFileSync(path.join(sslPath, "crt.crt"))
};
const server = require("https").Server(sslOptions, app);
const io = require("socket.io")(server);
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(conf.get("api_callback:path"), apiCallback.watch(io));
require("routes/socket")(io);
server.listen(conf.get("socket_port"));
routes/socket.js:
const { in_array } = require("lib/functions");
const loadUser = require("middleware/socket/loadUser");
const User = require("models/User");
module.exports = io => {
io.on("connection", async socket => {
let query = socket.handshake.query || {};
let { ssid } = query;
ssid = ssid || "";
let user = socket.user = await loadUser(ssid);
let oldPageName = null;
User.setOnline(user._id, 1);
socket.on("setPageName", pageName => {
if (oldPageName) socket.leave(oldPageName);
oldPageName = pageName;
socket.join(pageName);
});
socket.on("disconnect", () => {
socket.leave(oldPageName);
User.setOnline(user._id, 0);
});
});
};
Tell me how to properly close connections so that they do not remain in memory and do not load the server to such an extent that it kills the process of the MongoDB daemon?
node.js mongodb mongoose
add a comment |
up vote
0
down vote
favorite
I have a site, implemented on NodeJS, base MongoDB, Mongoose plugin. Recently, the site began to fall about once a day. I recently found out that this is due to the lack of memory, which is due to the fact that active connections are being accumulated (db.serverStatus (). Connections.current
). Perhaps this is not related, but I have a script on NodeJS, which is executed by crown every minute. It checks if there is a post with the current date in the documents. But I close the mongoose connection there, I don’t know what could be the problem. Actually this file contents:
process.env.NODE_TLS_REJECT_UNAUTHORIZED = 0;
const { new_time } = require("lib/functions");
const push = require("lib/push");
const apiCallback = require("middleware/socket/apiCallback");
const mongoose = require("lib/mongoose");
const User = require("models/User");
const Post = require("models/Post");
(async () => {
let currentPost = await Post.findCurrent(1);
if (currentPost) {
await currentPost.setPublished(1);
await apiCallback.call({
roomName: "index",
event : "posts.new",
data : {
post: {
id: currentPost._id.toString()
}
}
});
await push.sendAll({
// unnecessary data
});
}
await mongoose.connection.close();
process.exit(0);
})();
app.js:
const path = require("path");
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const expressSession = require("express-session");
const MongoStore = require("connect-mongo")(expressSession);
const conf = require("conf");
const mongoose = require("lib/mongoose");
const expressSessionConfig = conf.get("session");
expressSessionConfig.cookie.expires = new Date(new Date().getTime() + 60 * 60 * 24 * 30 * 1000);
expressSessionConfig.store = new MongoStore({
mongooseConnection: mongoose.connection
});
const templateDir = path.join(__dirname, conf.get("template_dir"));
app.engine("ejs", require("ejs-locals"));
app.set("views", templateDir);
app.set("view engine", "ejs")
app.use(express.static("frontend"));
app.use(cookieParser());
app.use(expressSession(expressSessionConfig));
app.use(bodyParser.urlencoded({
extended: true
}));
require("routes")(app);
app.listen(conf.get("app_port"));
app.io.js (socket server on socket.io):
const fs = require("fs");
const path = require("path");
const app = require("express")();
const bodyParser = require("body-parser");
const apiCallback = require("middleware/socket/apiCallback");
const conf = require("conf");
const sslPath = conf.get("sslPath");
const sslOptions = {
key : fs.readFileSync(path.join(sslPath, "key.key")),
cert: fs.readFileSync(path.join(sslPath, "crt.crt"))
};
const server = require("https").Server(sslOptions, app);
const io = require("socket.io")(server);
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(conf.get("api_callback:path"), apiCallback.watch(io));
require("routes/socket")(io);
server.listen(conf.get("socket_port"));
routes/socket.js:
const { in_array } = require("lib/functions");
const loadUser = require("middleware/socket/loadUser");
const User = require("models/User");
module.exports = io => {
io.on("connection", async socket => {
let query = socket.handshake.query || {};
let { ssid } = query;
ssid = ssid || "";
let user = socket.user = await loadUser(ssid);
let oldPageName = null;
User.setOnline(user._id, 1);
socket.on("setPageName", pageName => {
if (oldPageName) socket.leave(oldPageName);
oldPageName = pageName;
socket.join(pageName);
});
socket.on("disconnect", () => {
socket.leave(oldPageName);
User.setOnline(user._id, 0);
});
});
};
Tell me how to properly close connections so that they do not remain in memory and do not load the server to such an extent that it kills the process of the MongoDB daemon?
node.js mongodb mongoose
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a site, implemented on NodeJS, base MongoDB, Mongoose plugin. Recently, the site began to fall about once a day. I recently found out that this is due to the lack of memory, which is due to the fact that active connections are being accumulated (db.serverStatus (). Connections.current
). Perhaps this is not related, but I have a script on NodeJS, which is executed by crown every minute. It checks if there is a post with the current date in the documents. But I close the mongoose connection there, I don’t know what could be the problem. Actually this file contents:
process.env.NODE_TLS_REJECT_UNAUTHORIZED = 0;
const { new_time } = require("lib/functions");
const push = require("lib/push");
const apiCallback = require("middleware/socket/apiCallback");
const mongoose = require("lib/mongoose");
const User = require("models/User");
const Post = require("models/Post");
(async () => {
let currentPost = await Post.findCurrent(1);
if (currentPost) {
await currentPost.setPublished(1);
await apiCallback.call({
roomName: "index",
event : "posts.new",
data : {
post: {
id: currentPost._id.toString()
}
}
});
await push.sendAll({
// unnecessary data
});
}
await mongoose.connection.close();
process.exit(0);
})();
app.js:
const path = require("path");
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const expressSession = require("express-session");
const MongoStore = require("connect-mongo")(expressSession);
const conf = require("conf");
const mongoose = require("lib/mongoose");
const expressSessionConfig = conf.get("session");
expressSessionConfig.cookie.expires = new Date(new Date().getTime() + 60 * 60 * 24 * 30 * 1000);
expressSessionConfig.store = new MongoStore({
mongooseConnection: mongoose.connection
});
const templateDir = path.join(__dirname, conf.get("template_dir"));
app.engine("ejs", require("ejs-locals"));
app.set("views", templateDir);
app.set("view engine", "ejs")
app.use(express.static("frontend"));
app.use(cookieParser());
app.use(expressSession(expressSessionConfig));
app.use(bodyParser.urlencoded({
extended: true
}));
require("routes")(app);
app.listen(conf.get("app_port"));
app.io.js (socket server on socket.io):
const fs = require("fs");
const path = require("path");
const app = require("express")();
const bodyParser = require("body-parser");
const apiCallback = require("middleware/socket/apiCallback");
const conf = require("conf");
const sslPath = conf.get("sslPath");
const sslOptions = {
key : fs.readFileSync(path.join(sslPath, "key.key")),
cert: fs.readFileSync(path.join(sslPath, "crt.crt"))
};
const server = require("https").Server(sslOptions, app);
const io = require("socket.io")(server);
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(conf.get("api_callback:path"), apiCallback.watch(io));
require("routes/socket")(io);
server.listen(conf.get("socket_port"));
routes/socket.js:
const { in_array } = require("lib/functions");
const loadUser = require("middleware/socket/loadUser");
const User = require("models/User");
module.exports = io => {
io.on("connection", async socket => {
let query = socket.handshake.query || {};
let { ssid } = query;
ssid = ssid || "";
let user = socket.user = await loadUser(ssid);
let oldPageName = null;
User.setOnline(user._id, 1);
socket.on("setPageName", pageName => {
if (oldPageName) socket.leave(oldPageName);
oldPageName = pageName;
socket.join(pageName);
});
socket.on("disconnect", () => {
socket.leave(oldPageName);
User.setOnline(user._id, 0);
});
});
};
Tell me how to properly close connections so that they do not remain in memory and do not load the server to such an extent that it kills the process of the MongoDB daemon?
node.js mongodb mongoose
I have a site, implemented on NodeJS, base MongoDB, Mongoose plugin. Recently, the site began to fall about once a day. I recently found out that this is due to the lack of memory, which is due to the fact that active connections are being accumulated (db.serverStatus (). Connections.current
). Perhaps this is not related, but I have a script on NodeJS, which is executed by crown every minute. It checks if there is a post with the current date in the documents. But I close the mongoose connection there, I don’t know what could be the problem. Actually this file contents:
process.env.NODE_TLS_REJECT_UNAUTHORIZED = 0;
const { new_time } = require("lib/functions");
const push = require("lib/push");
const apiCallback = require("middleware/socket/apiCallback");
const mongoose = require("lib/mongoose");
const User = require("models/User");
const Post = require("models/Post");
(async () => {
let currentPost = await Post.findCurrent(1);
if (currentPost) {
await currentPost.setPublished(1);
await apiCallback.call({
roomName: "index",
event : "posts.new",
data : {
post: {
id: currentPost._id.toString()
}
}
});
await push.sendAll({
// unnecessary data
});
}
await mongoose.connection.close();
process.exit(0);
})();
app.js:
const path = require("path");
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const expressSession = require("express-session");
const MongoStore = require("connect-mongo")(expressSession);
const conf = require("conf");
const mongoose = require("lib/mongoose");
const expressSessionConfig = conf.get("session");
expressSessionConfig.cookie.expires = new Date(new Date().getTime() + 60 * 60 * 24 * 30 * 1000);
expressSessionConfig.store = new MongoStore({
mongooseConnection: mongoose.connection
});
const templateDir = path.join(__dirname, conf.get("template_dir"));
app.engine("ejs", require("ejs-locals"));
app.set("views", templateDir);
app.set("view engine", "ejs")
app.use(express.static("frontend"));
app.use(cookieParser());
app.use(expressSession(expressSessionConfig));
app.use(bodyParser.urlencoded({
extended: true
}));
require("routes")(app);
app.listen(conf.get("app_port"));
app.io.js (socket server on socket.io):
const fs = require("fs");
const path = require("path");
const app = require("express")();
const bodyParser = require("body-parser");
const apiCallback = require("middleware/socket/apiCallback");
const conf = require("conf");
const sslPath = conf.get("sslPath");
const sslOptions = {
key : fs.readFileSync(path.join(sslPath, "key.key")),
cert: fs.readFileSync(path.join(sslPath, "crt.crt"))
};
const server = require("https").Server(sslOptions, app);
const io = require("socket.io")(server);
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(conf.get("api_callback:path"), apiCallback.watch(io));
require("routes/socket")(io);
server.listen(conf.get("socket_port"));
routes/socket.js:
const { in_array } = require("lib/functions");
const loadUser = require("middleware/socket/loadUser");
const User = require("models/User");
module.exports = io => {
io.on("connection", async socket => {
let query = socket.handshake.query || {};
let { ssid } = query;
ssid = ssid || "";
let user = socket.user = await loadUser(ssid);
let oldPageName = null;
User.setOnline(user._id, 1);
socket.on("setPageName", pageName => {
if (oldPageName) socket.leave(oldPageName);
oldPageName = pageName;
socket.join(pageName);
});
socket.on("disconnect", () => {
socket.leave(oldPageName);
User.setOnline(user._id, 0);
});
});
};
Tell me how to properly close connections so that they do not remain in memory and do not load the server to such an extent that it kills the process of the MongoDB daemon?
node.js mongodb mongoose
node.js mongodb mongoose
asked Nov 8 at 19:31


ikerya
1205
1205
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
your code has no issues, you should go for connection pooling. your issue would automatically resolve. you will have a pool of connections whenever any api needs a db conenction. connection would be picked up from the pool and after completing db operation connection wouldn't be destroyed instead it would be returned back to the pool, in this manner your product's performance would be increased along with resolution to this issue.
https://mongoosejs.com/docs/connections.html
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
your code has no issues, you should go for connection pooling. your issue would automatically resolve. you will have a pool of connections whenever any api needs a db conenction. connection would be picked up from the pool and after completing db operation connection wouldn't be destroyed instead it would be returned back to the pool, in this manner your product's performance would be increased along with resolution to this issue.
https://mongoosejs.com/docs/connections.html
add a comment |
up vote
0
down vote
your code has no issues, you should go for connection pooling. your issue would automatically resolve. you will have a pool of connections whenever any api needs a db conenction. connection would be picked up from the pool and after completing db operation connection wouldn't be destroyed instead it would be returned back to the pool, in this manner your product's performance would be increased along with resolution to this issue.
https://mongoosejs.com/docs/connections.html
add a comment |
up vote
0
down vote
up vote
0
down vote
your code has no issues, you should go for connection pooling. your issue would automatically resolve. you will have a pool of connections whenever any api needs a db conenction. connection would be picked up from the pool and after completing db operation connection wouldn't be destroyed instead it would be returned back to the pool, in this manner your product's performance would be increased along with resolution to this issue.
https://mongoosejs.com/docs/connections.html
your code has no issues, you should go for connection pooling. your issue would automatically resolve. you will have a pool of connections whenever any api needs a db conenction. connection would be picked up from the pool and after completing db operation connection wouldn't be destroyed instead it would be returned back to the pool, in this manner your product's performance would be increased along with resolution to this issue.
https://mongoosejs.com/docs/connections.html
answered Nov 8 at 19:50


Asad Mehmood
1196
1196
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53214872%2fmongodb-active-connections-are-accumulating-what-should-i-do%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UQPZdE KMVL A,wf,lEkTFoVa KC,2Bd6t MpniGqQK,Eg3 LR9b O CJSlNH0IIe,O6p5d TCMeolwqUxX,ELac