upload file with Alamofire swift 4
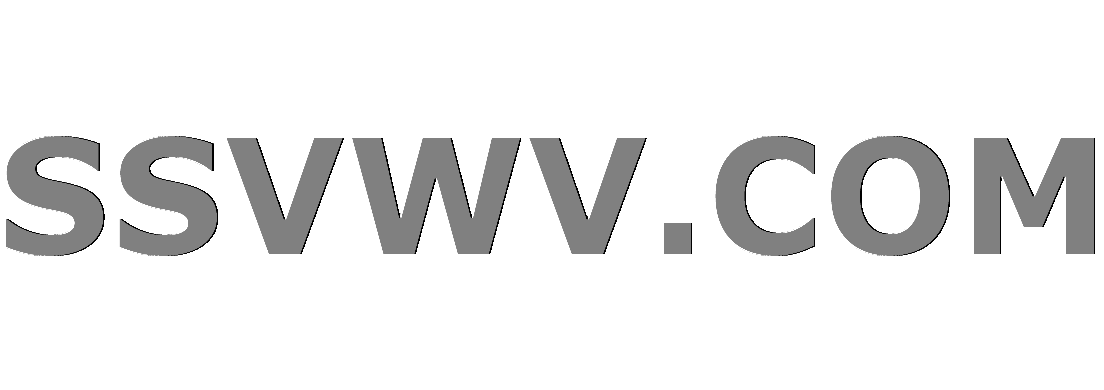
Multi tool use
up vote
-2
down vote
favorite
I'm trying to upload image using Alamofire (swift 4.2), but when converting image to data I got this error
Extra argument 'compressionQuality' in call
the code is
import Foundation
import SwiftyJSON
import Alamofire
class APIUpload {
class func createPhoto(photo: UIImage, completion: @escaping (_ error:Error?, _ success: Bool)->Void){
let url = "http://www.fb.test/photo.php"
Alamofire.upload(multipartFormData: { (form: MultipartFormData) in
let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:Data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
}, usingThreshold: SessionManager.multipartFormDataEncodingMemoryThreshold, to: url, method: .post, headers: nil) { (result: SessionManager.MultipartFormDataEncodingResult) in
switch result {
case .failure(let error):
print(error)
completion(error, false)
case .success(request: let upload, streamingFromDisk: _, streamFileURL: _):
upload.uploadProgress(closure: { (progress: Progress) in
print(progress)
})
upload.responseJSON(completionHandler: { (response: DataResponse<Any>) in
switch response.result{
case .failure(let error):
print(error)
case .success(let value):
let json = JSON(value)
print(json)
completion(nil, true)
}
})
}
}
}
}
error
enter image description here
swift alamofire
add a comment |
up vote
-2
down vote
favorite
I'm trying to upload image using Alamofire (swift 4.2), but when converting image to data I got this error
Extra argument 'compressionQuality' in call
the code is
import Foundation
import SwiftyJSON
import Alamofire
class APIUpload {
class func createPhoto(photo: UIImage, completion: @escaping (_ error:Error?, _ success: Bool)->Void){
let url = "http://www.fb.test/photo.php"
Alamofire.upload(multipartFormData: { (form: MultipartFormData) in
let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:Data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
}, usingThreshold: SessionManager.multipartFormDataEncodingMemoryThreshold, to: url, method: .post, headers: nil) { (result: SessionManager.MultipartFormDataEncodingResult) in
switch result {
case .failure(let error):
print(error)
completion(error, false)
case .success(request: let upload, streamingFromDisk: _, streamFileURL: _):
upload.uploadProgress(closure: { (progress: Progress) in
print(progress)
})
upload.responseJSON(completionHandler: { (response: DataResponse<Any>) in
switch response.result{
case .failure(let error):
print(error)
case .success(let value):
let json = JSON(value)
print(json)
completion(nil, true)
}
})
}
}
}
}
error
enter image description here
swift alamofire
I suspect the error message is a little misleading here. Why isform.append
inside a closure at the end ofjpegData
?
– John Montgomery
Nov 8 at 19:40
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I'm trying to upload image using Alamofire (swift 4.2), but when converting image to data I got this error
Extra argument 'compressionQuality' in call
the code is
import Foundation
import SwiftyJSON
import Alamofire
class APIUpload {
class func createPhoto(photo: UIImage, completion: @escaping (_ error:Error?, _ success: Bool)->Void){
let url = "http://www.fb.test/photo.php"
Alamofire.upload(multipartFormData: { (form: MultipartFormData) in
let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:Data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
}, usingThreshold: SessionManager.multipartFormDataEncodingMemoryThreshold, to: url, method: .post, headers: nil) { (result: SessionManager.MultipartFormDataEncodingResult) in
switch result {
case .failure(let error):
print(error)
completion(error, false)
case .success(request: let upload, streamingFromDisk: _, streamFileURL: _):
upload.uploadProgress(closure: { (progress: Progress) in
print(progress)
})
upload.responseJSON(completionHandler: { (response: DataResponse<Any>) in
switch response.result{
case .failure(let error):
print(error)
case .success(let value):
let json = JSON(value)
print(json)
completion(nil, true)
}
})
}
}
}
}
error
enter image description here
swift alamofire
I'm trying to upload image using Alamofire (swift 4.2), but when converting image to data I got this error
Extra argument 'compressionQuality' in call
the code is
import Foundation
import SwiftyJSON
import Alamofire
class APIUpload {
class func createPhoto(photo: UIImage, completion: @escaping (_ error:Error?, _ success: Bool)->Void){
let url = "http://www.fb.test/photo.php"
Alamofire.upload(multipartFormData: { (form: MultipartFormData) in
let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:Data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
}, usingThreshold: SessionManager.multipartFormDataEncodingMemoryThreshold, to: url, method: .post, headers: nil) { (result: SessionManager.MultipartFormDataEncodingResult) in
switch result {
case .failure(let error):
print(error)
completion(error, false)
case .success(request: let upload, streamingFromDisk: _, streamFileURL: _):
upload.uploadProgress(closure: { (progress: Progress) in
print(progress)
})
upload.responseJSON(completionHandler: { (response: DataResponse<Any>) in
switch response.result{
case .failure(let error):
print(error)
case .success(let value):
let json = JSON(value)
print(json)
completion(nil, true)
}
})
}
}
}
}
error
enter image description here
swift alamofire
swift alamofire
asked Nov 8 at 19:34
ethar
1
1
I suspect the error message is a little misleading here. Why isform.append
inside a closure at the end ofjpegData
?
– John Montgomery
Nov 8 at 19:40
add a comment |
I suspect the error message is a little misleading here. Why isform.append
inside a closure at the end ofjpegData
?
– John Montgomery
Nov 8 at 19:40
I suspect the error message is a little misleading here. Why is
form.append
inside a closure at the end of jpegData
?– John Montgomery
Nov 8 at 19:40
I suspect the error message is a little misleading here. Why is
form.append
inside a closure at the end of jpegData
?– John Montgomery
Nov 8 at 19:40
add a comment |
1 Answer
1
active
oldest
votes
up vote
-1
down vote
Signature of jpegData
func jpegData(compressionQuality: CGFloat) -> Data?
so you need
if let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
Signature of jpegData
func jpegData(compressionQuality: CGFloat) -> Data?
so you need
if let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
add a comment |
up vote
-1
down vote
Signature of jpegData
func jpegData(compressionQuality: CGFloat) -> Data?
so you need
if let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Signature of jpegData
func jpegData(compressionQuality: CGFloat) -> Data?
so you need
if let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
Signature of jpegData
func jpegData(compressionQuality: CGFloat) -> Data?
so you need
if let data = photo.jpegData(compressionQuality: 1.0){
form.append(data:data, withName:"photo",fileName:"photo.jpeg",mimeType:"image/jpeg")
}
answered Nov 8 at 19:44
Sh_Khan
34.2k41125
34.2k41125
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53214928%2fupload-file-with-alamofire-swift-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UrgPD CLVHCa 5zPFF7oLCtQdZ9yVktZd0CnJ5Y,i,CT lKBDCgsxy0gN6aywSbcn,Bm,633uOdE,2S
I suspect the error message is a little misleading here. Why is
form.append
inside a closure at the end ofjpegData
?– John Montgomery
Nov 8 at 19:40