What determines what fields can be updated in DjangoRestFramework
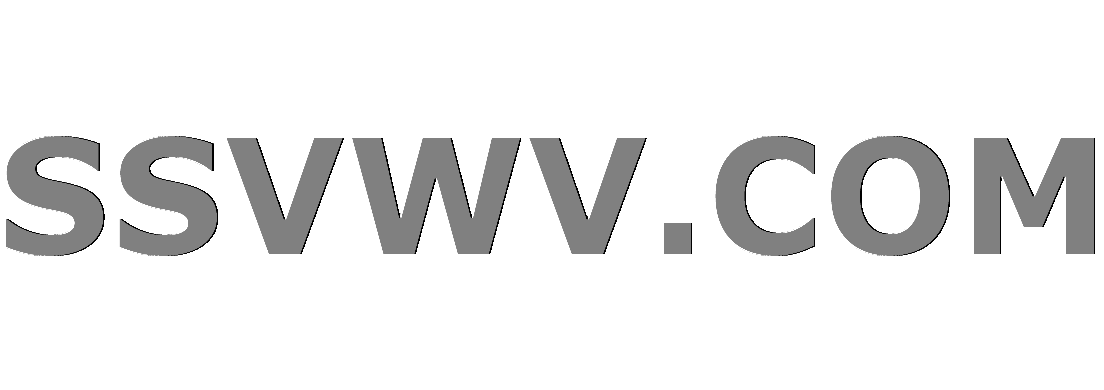
Multi tool use
up vote
0
down vote
favorite
I currently have a serializer with the following fields
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
Serializer
class AssetSerializer(serializers.ModelSerializer):
checkin_status = serializers.SerializerMethodField()
allocation_history = serializers.SerializerMethodField()
assigned_to = UserSerializer(read_only=True)
asset_category = serializers.SerializerMethodField()
asset_sub_category = serializers.SerializerMethodField()
make_label = serializers.SerializerMethodField()
asset_type = serializers.SerializerMethodField()
model_number = serializers.SlugRelatedField(
queryset=AssetModelNumber.objects.all(),
slug_field="model_number"
)
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
depth = 1
read_only_fields = ("uuid",)
View
class ManageAssetViewSet(ModelViewSet):
serializer_class = AssetSerializer
queryset = Asset.objects.all()
# permission_classes = [IsAuthenticated, IsAdminUser]
# authentication_classes = (FirebaseTokenAuthentication,)
http_method_names = ['get', 'post', 'put', 'delete']
filter_backends = (filters.DjangoFilterBackend,)
filterset_class = AssetFilter
def get_object(self):
queryset = Asset.objects.all()
obj = get_object_or_404(queryset, uuid=self.kwargs['pk'])
return obj
Model
Asset Model. Some fields have been ommited
class Asset(models.Model):
"""Stores all assets"""
uuid = models.UUIDField(unique=True, default=uuid.uuid4, editable=False)
asset_code = models.CharField(
unique=True, null=True, blank=True, max_length=50)
serial_number = models.CharField(
unique=True, null=True, blank=True, max_length=50)
created_at = models.DateTimeField(auto_now_add=True, editable=False)
asset_location = models.ForeignKey('AndelaCentre', blank=True, editable=True, null=True,
on_delete=models.PROTECT)
purchase_date = models.DateField(
validators=[validate_date],
null=True, blank=True)
last_modified = models.DateTimeField(auto_now=True, editable=False)
assigned_to = models.ForeignKey('AssetAssignee',
blank=True,
editable=False,
null=True,
on_delete=models.PROTECT)
model_number = models.ForeignKey(AssetModelNumber,
null=True,
on_delete=models.PROTECT)
current_status = models.CharField(editable=False, max_length=50)
notes = models.TextField(editable=False, default=" ", )
However, on the browsable Api, only 4 fields are showing on the UPDATE/PUT
form as shown in the diagram below
What could be the reason some of the other fields are not appearing here. What determines which fields are updatable??
python django django-rest-framework django-rest-viewsets
add a comment |
up vote
0
down vote
favorite
I currently have a serializer with the following fields
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
Serializer
class AssetSerializer(serializers.ModelSerializer):
checkin_status = serializers.SerializerMethodField()
allocation_history = serializers.SerializerMethodField()
assigned_to = UserSerializer(read_only=True)
asset_category = serializers.SerializerMethodField()
asset_sub_category = serializers.SerializerMethodField()
make_label = serializers.SerializerMethodField()
asset_type = serializers.SerializerMethodField()
model_number = serializers.SlugRelatedField(
queryset=AssetModelNumber.objects.all(),
slug_field="model_number"
)
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
depth = 1
read_only_fields = ("uuid",)
View
class ManageAssetViewSet(ModelViewSet):
serializer_class = AssetSerializer
queryset = Asset.objects.all()
# permission_classes = [IsAuthenticated, IsAdminUser]
# authentication_classes = (FirebaseTokenAuthentication,)
http_method_names = ['get', 'post', 'put', 'delete']
filter_backends = (filters.DjangoFilterBackend,)
filterset_class = AssetFilter
def get_object(self):
queryset = Asset.objects.all()
obj = get_object_or_404(queryset, uuid=self.kwargs['pk'])
return obj
Model
Asset Model. Some fields have been ommited
class Asset(models.Model):
"""Stores all assets"""
uuid = models.UUIDField(unique=True, default=uuid.uuid4, editable=False)
asset_code = models.CharField(
unique=True, null=True, blank=True, max_length=50)
serial_number = models.CharField(
unique=True, null=True, blank=True, max_length=50)
created_at = models.DateTimeField(auto_now_add=True, editable=False)
asset_location = models.ForeignKey('AndelaCentre', blank=True, editable=True, null=True,
on_delete=models.PROTECT)
purchase_date = models.DateField(
validators=[validate_date],
null=True, blank=True)
last_modified = models.DateTimeField(auto_now=True, editable=False)
assigned_to = models.ForeignKey('AssetAssignee',
blank=True,
editable=False,
null=True,
on_delete=models.PROTECT)
model_number = models.ForeignKey(AssetModelNumber,
null=True,
on_delete=models.PROTECT)
current_status = models.CharField(editable=False, max_length=50)
notes = models.TextField(editable=False, default=" ", )
However, on the browsable Api, only 4 fields are showing on the UPDATE/PUT
form as shown in the diagram below
What could be the reason some of the other fields are not appearing here. What determines which fields are updatable??
python django django-rest-framework django-rest-viewsets
2
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Just added them
– Esir Kings
Nov 8 at 10:03
add model details?
– a_k_v
Nov 8 at 10:07
Just added them
– Esir Kings
Nov 8 at 10:11
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I currently have a serializer with the following fields
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
Serializer
class AssetSerializer(serializers.ModelSerializer):
checkin_status = serializers.SerializerMethodField()
allocation_history = serializers.SerializerMethodField()
assigned_to = UserSerializer(read_only=True)
asset_category = serializers.SerializerMethodField()
asset_sub_category = serializers.SerializerMethodField()
make_label = serializers.SerializerMethodField()
asset_type = serializers.SerializerMethodField()
model_number = serializers.SlugRelatedField(
queryset=AssetModelNumber.objects.all(),
slug_field="model_number"
)
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
depth = 1
read_only_fields = ("uuid",)
View
class ManageAssetViewSet(ModelViewSet):
serializer_class = AssetSerializer
queryset = Asset.objects.all()
# permission_classes = [IsAuthenticated, IsAdminUser]
# authentication_classes = (FirebaseTokenAuthentication,)
http_method_names = ['get', 'post', 'put', 'delete']
filter_backends = (filters.DjangoFilterBackend,)
filterset_class = AssetFilter
def get_object(self):
queryset = Asset.objects.all()
obj = get_object_or_404(queryset, uuid=self.kwargs['pk'])
return obj
Model
Asset Model. Some fields have been ommited
class Asset(models.Model):
"""Stores all assets"""
uuid = models.UUIDField(unique=True, default=uuid.uuid4, editable=False)
asset_code = models.CharField(
unique=True, null=True, blank=True, max_length=50)
serial_number = models.CharField(
unique=True, null=True, blank=True, max_length=50)
created_at = models.DateTimeField(auto_now_add=True, editable=False)
asset_location = models.ForeignKey('AndelaCentre', blank=True, editable=True, null=True,
on_delete=models.PROTECT)
purchase_date = models.DateField(
validators=[validate_date],
null=True, blank=True)
last_modified = models.DateTimeField(auto_now=True, editable=False)
assigned_to = models.ForeignKey('AssetAssignee',
blank=True,
editable=False,
null=True,
on_delete=models.PROTECT)
model_number = models.ForeignKey(AssetModelNumber,
null=True,
on_delete=models.PROTECT)
current_status = models.CharField(editable=False, max_length=50)
notes = models.TextField(editable=False, default=" ", )
However, on the browsable Api, only 4 fields are showing on the UPDATE/PUT
form as shown in the diagram below
What could be the reason some of the other fields are not appearing here. What determines which fields are updatable??
python django django-rest-framework django-rest-viewsets
I currently have a serializer with the following fields
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
Serializer
class AssetSerializer(serializers.ModelSerializer):
checkin_status = serializers.SerializerMethodField()
allocation_history = serializers.SerializerMethodField()
assigned_to = UserSerializer(read_only=True)
asset_category = serializers.SerializerMethodField()
asset_sub_category = serializers.SerializerMethodField()
make_label = serializers.SerializerMethodField()
asset_type = serializers.SerializerMethodField()
model_number = serializers.SlugRelatedField(
queryset=AssetModelNumber.objects.all(),
slug_field="model_number"
)
class Meta:
model = Asset
fields = ('id', 'uuid', 'asset_category', 'asset_sub_category',
'make_label',
'asset_code', 'serial_number', 'model_number',
'checkin_status', 'created_at',
'last_modified', 'current_status', 'asset_type',
'allocation_history', 'specs', 'purchase_date',
'notes', 'assigned_to', 'asset_location'
)
depth = 1
read_only_fields = ("uuid",)
View
class ManageAssetViewSet(ModelViewSet):
serializer_class = AssetSerializer
queryset = Asset.objects.all()
# permission_classes = [IsAuthenticated, IsAdminUser]
# authentication_classes = (FirebaseTokenAuthentication,)
http_method_names = ['get', 'post', 'put', 'delete']
filter_backends = (filters.DjangoFilterBackend,)
filterset_class = AssetFilter
def get_object(self):
queryset = Asset.objects.all()
obj = get_object_or_404(queryset, uuid=self.kwargs['pk'])
return obj
Model
Asset Model. Some fields have been ommited
class Asset(models.Model):
"""Stores all assets"""
uuid = models.UUIDField(unique=True, default=uuid.uuid4, editable=False)
asset_code = models.CharField(
unique=True, null=True, blank=True, max_length=50)
serial_number = models.CharField(
unique=True, null=True, blank=True, max_length=50)
created_at = models.DateTimeField(auto_now_add=True, editable=False)
asset_location = models.ForeignKey('AndelaCentre', blank=True, editable=True, null=True,
on_delete=models.PROTECT)
purchase_date = models.DateField(
validators=[validate_date],
null=True, blank=True)
last_modified = models.DateTimeField(auto_now=True, editable=False)
assigned_to = models.ForeignKey('AssetAssignee',
blank=True,
editable=False,
null=True,
on_delete=models.PROTECT)
model_number = models.ForeignKey(AssetModelNumber,
null=True,
on_delete=models.PROTECT)
current_status = models.CharField(editable=False, max_length=50)
notes = models.TextField(editable=False, default=" ", )
However, on the browsable Api, only 4 fields are showing on the UPDATE/PUT
form as shown in the diagram below
What could be the reason some of the other fields are not appearing here. What determines which fields are updatable??
python django django-rest-framework django-rest-viewsets
python django django-rest-framework django-rest-viewsets
edited Nov 8 at 10:11
asked Nov 8 at 9:41


Esir Kings
643421
643421
2
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Just added them
– Esir Kings
Nov 8 at 10:03
add model details?
– a_k_v
Nov 8 at 10:07
Just added them
– Esir Kings
Nov 8 at 10:11
add a comment |
2
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Just added them
– Esir Kings
Nov 8 at 10:03
add model details?
– a_k_v
Nov 8 at 10:07
Just added them
– Esir Kings
Nov 8 at 10:11
2
2
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Just added them
– Esir Kings
Nov 8 at 10:03
Just added them
– Esir Kings
Nov 8 at 10:03
add model details?
– a_k_v
Nov 8 at 10:07
add model details?
– a_k_v
Nov 8 at 10:07
Just added them
– Esir Kings
Nov 8 at 10:11
Just added them
– Esir Kings
Nov 8 at 10:11
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
Well, the problem is when you set depth = 1 ModelSerializer tries to generate a NestedSerializer field for any foreignkey related field which you have not explicitly mentioned. And that NestedSerializer field is a read only field. That's why Assest Location is not being displayed. Remove that depth = 1 line and DRF will map the said field with the default mapping i.e. PrimaryKeyRelatedFiel and you will see that the said field is displayed.
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
add a comment |
up vote
0
down vote
In your model, you make editable=False
for many fields. That fields won't display. If you want to display and edit that field remove that option.
For more info refer question
Asset location haseditable
as true but still isn't displaying
– Esir Kings
Nov 8 at 10:43
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Well, the problem is when you set depth = 1 ModelSerializer tries to generate a NestedSerializer field for any foreignkey related field which you have not explicitly mentioned. And that NestedSerializer field is a read only field. That's why Assest Location is not being displayed. Remove that depth = 1 line and DRF will map the said field with the default mapping i.e. PrimaryKeyRelatedFiel and you will see that the said field is displayed.
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
add a comment |
up vote
1
down vote
accepted
Well, the problem is when you set depth = 1 ModelSerializer tries to generate a NestedSerializer field for any foreignkey related field which you have not explicitly mentioned. And that NestedSerializer field is a read only field. That's why Assest Location is not being displayed. Remove that depth = 1 line and DRF will map the said field with the default mapping i.e. PrimaryKeyRelatedFiel and you will see that the said field is displayed.
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Well, the problem is when you set depth = 1 ModelSerializer tries to generate a NestedSerializer field for any foreignkey related field which you have not explicitly mentioned. And that NestedSerializer field is a read only field. That's why Assest Location is not being displayed. Remove that depth = 1 line and DRF will map the said field with the default mapping i.e. PrimaryKeyRelatedFiel and you will see that the said field is displayed.
Well, the problem is when you set depth = 1 ModelSerializer tries to generate a NestedSerializer field for any foreignkey related field which you have not explicitly mentioned. And that NestedSerializer field is a read only field. That's why Assest Location is not being displayed. Remove that depth = 1 line and DRF will map the said field with the default mapping i.e. PrimaryKeyRelatedFiel and you will see that the said field is displayed.
answered Nov 8 at 19:33
dipen bhatt
617
617
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
add a comment |
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
thanks for this
– Esir Kings
Nov 8 at 19:50
thanks for this
– Esir Kings
Nov 8 at 19:50
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
No problem bro!!
– dipen bhatt
Nov 9 at 20:02
add a comment |
up vote
0
down vote
In your model, you make editable=False
for many fields. That fields won't display. If you want to display and edit that field remove that option.
For more info refer question
Asset location haseditable
as true but still isn't displaying
– Esir Kings
Nov 8 at 10:43
add a comment |
up vote
0
down vote
In your model, you make editable=False
for many fields. That fields won't display. If you want to display and edit that field remove that option.
For more info refer question
Asset location haseditable
as true but still isn't displaying
– Esir Kings
Nov 8 at 10:43
add a comment |
up vote
0
down vote
up vote
0
down vote
In your model, you make editable=False
for many fields. That fields won't display. If you want to display and edit that field remove that option.
For more info refer question
In your model, you make editable=False
for many fields. That fields won't display. If you want to display and edit that field remove that option.
For more info refer question
answered Nov 8 at 10:15
a_k_v
57112
57112
Asset location haseditable
as true but still isn't displaying
– Esir Kings
Nov 8 at 10:43
add a comment |
Asset location haseditable
as true but still isn't displaying
– Esir Kings
Nov 8 at 10:43
Asset location has
editable
as true but still isn't displaying– Esir Kings
Nov 8 at 10:43
Asset location has
editable
as true but still isn't displaying– Esir Kings
Nov 8 at 10:43
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53205047%2fwhat-determines-what-fields-can-be-updated-in-djangorestframework%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
rvwo1c1dyjktiJxAW,IQWL9ZTD88T4guwLPHc
2
Share model view and serializer
– a_k_v
Nov 8 at 9:59
Just added them
– Esir Kings
Nov 8 at 10:03
add model details?
– a_k_v
Nov 8 at 10:07
Just added them
– Esir Kings
Nov 8 at 10:11