How can I check if a value exists in another table's last N rows?
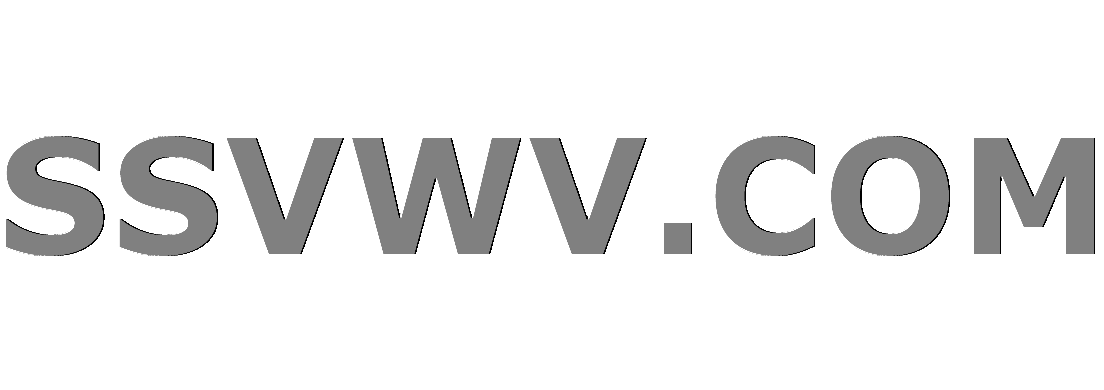
Multi tool use
up vote
0
down vote
favorite
used
contains a list of used items. I don't want the item to be used again if it was one of the last 10 items used.
From some googling, it seems like I need a subquery but can't get it to work. Here is what I tried so far
$check = mysqli_query($db, 'SELECT `id`
FROM (
SELECT `id`
FROM `used`
ORDER BY `id` DESC
LIMIT 10)
WHERE `item` = ' . $id);
Before this I was using the following
$check = mysqli_query($db, 'SELECT `id`
FROM `used`
WHERE `item` = ' . $id . '
ORDER BY `id` DESC
LIMIT 10);
However, seems like that was only limiting the results by 10, so would always return true
if an item had been used, even outside of the last 10
php mysql sql database
add a comment |
up vote
0
down vote
favorite
used
contains a list of used items. I don't want the item to be used again if it was one of the last 10 items used.
From some googling, it seems like I need a subquery but can't get it to work. Here is what I tried so far
$check = mysqli_query($db, 'SELECT `id`
FROM (
SELECT `id`
FROM `used`
ORDER BY `id` DESC
LIMIT 10)
WHERE `item` = ' . $id);
Before this I was using the following
$check = mysqli_query($db, 'SELECT `id`
FROM `used`
WHERE `item` = ' . $id . '
ORDER BY `id` DESC
LIMIT 10);
However, seems like that was only limiting the results by 10, so would always return true
if an item had been used, even outside of the last 10
php mysql sql database
1
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
used
contains a list of used items. I don't want the item to be used again if it was one of the last 10 items used.
From some googling, it seems like I need a subquery but can't get it to work. Here is what I tried so far
$check = mysqli_query($db, 'SELECT `id`
FROM (
SELECT `id`
FROM `used`
ORDER BY `id` DESC
LIMIT 10)
WHERE `item` = ' . $id);
Before this I was using the following
$check = mysqli_query($db, 'SELECT `id`
FROM `used`
WHERE `item` = ' . $id . '
ORDER BY `id` DESC
LIMIT 10);
However, seems like that was only limiting the results by 10, so would always return true
if an item had been used, even outside of the last 10
php mysql sql database
used
contains a list of used items. I don't want the item to be used again if it was one of the last 10 items used.
From some googling, it seems like I need a subquery but can't get it to work. Here is what I tried so far
$check = mysqli_query($db, 'SELECT `id`
FROM (
SELECT `id`
FROM `used`
ORDER BY `id` DESC
LIMIT 10)
WHERE `item` = ' . $id);
Before this I was using the following
$check = mysqli_query($db, 'SELECT `id`
FROM `used`
WHERE `item` = ' . $id . '
ORDER BY `id` DESC
LIMIT 10);
However, seems like that was only limiting the results by 10, so would always return true
if an item had been used, even outside of the last 10
php mysql sql database
php mysql sql database
edited Nov 10 at 1:12


RiggsFolly
69k1764109
69k1764109
asked Nov 10 at 0:53
cantsay
76911026
76911026
1
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09
add a comment |
1
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09
1
1
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
It sounds like you want to check if the item $id
was in the last 10 items in the used
table. This query should do that:
$check = mysqli_query($db, "SELECT $id NOT IN (SELECT item
FROM used
ORDER BY id DESC
LIMIT 10)");
This query will return 1
if the value of $id
was not in the last 10 values of item
in the used
table, or 0
otherwise.
For versions of MySQL which don't support LIMIT
in IN
subqueries, you can use a LEFT JOIN
as in this query which will return the same result:
SELECT IF(b.item IS NULL, 1, 0)
FROM used a
LEFT JOIN (SELECT item
FROM used
ORDER BY id DESC LIMIT 10) b ON b.item = a.item
WHERE a.item = $id
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
add a comment |
up vote
1
down vote
You can do it with a JOIN:
$check = mysqli_query($db, 'SELECT u.id
FROM used AS u
JOIN (
SELECT id
FROM used
ORDER BY id DESC LIMIT 10) AS ub
USING (id)
WHERE item = ' . $id);
add a comment |
up vote
1
down vote
This works if your version does not support 'LIMIT & IN/ALL/ANY/SOME subquery":
$check = mysqli_query($db, 'SELECT `item` FROM
(SELECT `item`
FROM `used`
ORDER BY `id` DESC
LIMIT 10) as useditems
WHERE `item` = ' . $id );
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
It sounds like you want to check if the item $id
was in the last 10 items in the used
table. This query should do that:
$check = mysqli_query($db, "SELECT $id NOT IN (SELECT item
FROM used
ORDER BY id DESC
LIMIT 10)");
This query will return 1
if the value of $id
was not in the last 10 values of item
in the used
table, or 0
otherwise.
For versions of MySQL which don't support LIMIT
in IN
subqueries, you can use a LEFT JOIN
as in this query which will return the same result:
SELECT IF(b.item IS NULL, 1, 0)
FROM used a
LEFT JOIN (SELECT item
FROM used
ORDER BY id DESC LIMIT 10) b ON b.item = a.item
WHERE a.item = $id
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
add a comment |
up vote
2
down vote
It sounds like you want to check if the item $id
was in the last 10 items in the used
table. This query should do that:
$check = mysqli_query($db, "SELECT $id NOT IN (SELECT item
FROM used
ORDER BY id DESC
LIMIT 10)");
This query will return 1
if the value of $id
was not in the last 10 values of item
in the used
table, or 0
otherwise.
For versions of MySQL which don't support LIMIT
in IN
subqueries, you can use a LEFT JOIN
as in this query which will return the same result:
SELECT IF(b.item IS NULL, 1, 0)
FROM used a
LEFT JOIN (SELECT item
FROM used
ORDER BY id DESC LIMIT 10) b ON b.item = a.item
WHERE a.item = $id
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
add a comment |
up vote
2
down vote
up vote
2
down vote
It sounds like you want to check if the item $id
was in the last 10 items in the used
table. This query should do that:
$check = mysqli_query($db, "SELECT $id NOT IN (SELECT item
FROM used
ORDER BY id DESC
LIMIT 10)");
This query will return 1
if the value of $id
was not in the last 10 values of item
in the used
table, or 0
otherwise.
For versions of MySQL which don't support LIMIT
in IN
subqueries, you can use a LEFT JOIN
as in this query which will return the same result:
SELECT IF(b.item IS NULL, 1, 0)
FROM used a
LEFT JOIN (SELECT item
FROM used
ORDER BY id DESC LIMIT 10) b ON b.item = a.item
WHERE a.item = $id
It sounds like you want to check if the item $id
was in the last 10 items in the used
table. This query should do that:
$check = mysqli_query($db, "SELECT $id NOT IN (SELECT item
FROM used
ORDER BY id DESC
LIMIT 10)");
This query will return 1
if the value of $id
was not in the last 10 values of item
in the used
table, or 0
otherwise.
For versions of MySQL which don't support LIMIT
in IN
subqueries, you can use a LEFT JOIN
as in this query which will return the same result:
SELECT IF(b.item IS NULL, 1, 0)
FROM used a
LEFT JOIN (SELECT item
FROM used
ORDER BY id DESC LIMIT 10) b ON b.item = a.item
WHERE a.item = $id
edited Nov 10 at 1:54
answered Nov 10 at 1:15
Nick
20.6k51434
20.6k51434
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
add a comment |
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
Thanks. Can't get this to work due to the following, "This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery" but I think I found a fix for that, didn't know about (NOT) IN.
– cantsay
Nov 10 at 1:24
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
@cantsay I've added an alternate solution for earlier versions of MySQL
– Nick
Nov 10 at 1:55
add a comment |
up vote
1
down vote
You can do it with a JOIN:
$check = mysqli_query($db, 'SELECT u.id
FROM used AS u
JOIN (
SELECT id
FROM used
ORDER BY id DESC LIMIT 10) AS ub
USING (id)
WHERE item = ' . $id);
add a comment |
up vote
1
down vote
You can do it with a JOIN:
$check = mysqli_query($db, 'SELECT u.id
FROM used AS u
JOIN (
SELECT id
FROM used
ORDER BY id DESC LIMIT 10) AS ub
USING (id)
WHERE item = ' . $id);
add a comment |
up vote
1
down vote
up vote
1
down vote
You can do it with a JOIN:
$check = mysqli_query($db, 'SELECT u.id
FROM used AS u
JOIN (
SELECT id
FROM used
ORDER BY id DESC LIMIT 10) AS ub
USING (id)
WHERE item = ' . $id);
You can do it with a JOIN:
$check = mysqli_query($db, 'SELECT u.id
FROM used AS u
JOIN (
SELECT id
FROM used
ORDER BY id DESC LIMIT 10) AS ub
USING (id)
WHERE item = ' . $id);
answered Nov 10 at 1:27
Antonio Alvarez
10214
10214
add a comment |
add a comment |
up vote
1
down vote
This works if your version does not support 'LIMIT & IN/ALL/ANY/SOME subquery":
$check = mysqli_query($db, 'SELECT `item` FROM
(SELECT `item`
FROM `used`
ORDER BY `id` DESC
LIMIT 10) as useditems
WHERE `item` = ' . $id );
add a comment |
up vote
1
down vote
This works if your version does not support 'LIMIT & IN/ALL/ANY/SOME subquery":
$check = mysqli_query($db, 'SELECT `item` FROM
(SELECT `item`
FROM `used`
ORDER BY `id` DESC
LIMIT 10) as useditems
WHERE `item` = ' . $id );
add a comment |
up vote
1
down vote
up vote
1
down vote
This works if your version does not support 'LIMIT & IN/ALL/ANY/SOME subquery":
$check = mysqli_query($db, 'SELECT `item` FROM
(SELECT `item`
FROM `used`
ORDER BY `id` DESC
LIMIT 10) as useditems
WHERE `item` = ' . $id );
This works if your version does not support 'LIMIT & IN/ALL/ANY/SOME subquery":
$check = mysqli_query($db, 'SELECT `item` FROM
(SELECT `item`
FROM `used`
ORDER BY `id` DESC
LIMIT 10) as useditems
WHERE `item` = ' . $id );
answered Nov 10 at 1:29
Manpreet
40016
40016
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53235066%2fhow-can-i-check-if-a-value-exists-in-another-tables-last-n-rows%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JmmMRutfC4GyIi,j7LBIa8s,CYEyrl5t9bAocfspTW,iNkWpf6GDO7PQoC
1
See meta.stackoverflow.com/questions/333952/…
– Strawberry
Nov 10 at 1:09