How to structure and monitor user posts on blog style app using Firebase
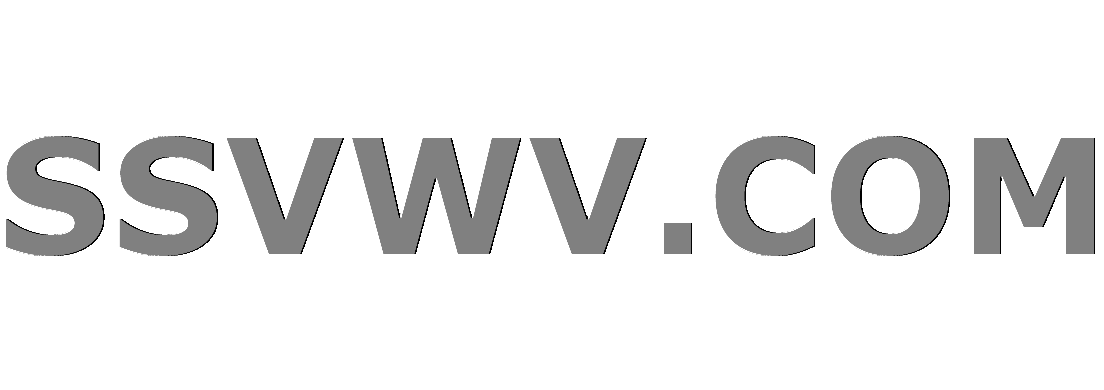
Multi tool use
up vote
0
down vote
favorite
I am building an app that allows users to make public posts, you can make a post and view all new posts on the home page. I am struggling to find the best way to efficiently structure the database data and monitor all new posts that are made, as currently if a user is logged in, they can post anything without restriction. I need an effective way to screen posts for content that is uploaded to Firebase, or even a solution that when a new post is made it is sent for approval, rather than being sent on the database straight away. How should I manage this?
Here is the way that posts are uploaded to the database, I use duplicates of this structure also under authors
and categories
, which I am not sure if it is a good way to do it or not, should I be finding a way to have 1 instance of a post on the database, and instead use some kind of IDs to find it in different situations such as by author or by category?
"posts": {
-childGeneratedKey: {
author: "author",
body: "body",
category: "category",
post_date: "1541784510.682485",
post_id: "childAddedID",
title: "post_title",
votes:"0",
}
-childGeneratedKey: {...
}
Here is how I handle posting in-app:
func createPost(title: String, body: String) {
let timeInterval = NSDate().timeIntervalSince1970
let timeDouble = Double(timeInterval)
ref = Database.database().reference()
let posts = self.ref.child("posts")
let post_id = posts.childByAutoId().key!
let category = self.category
let post_date = timeDouble
if postTitle != "" {
let newPost: Dictionary<String, Any> = [
"title": title,
"body": body,
"votes": 0,
"author": username,
"post_id": post_id,
"category": category,
"post_date": post_date
]
if privatePost == false {
createNewPost(post: newPost, post_id: post_id, category: category)
} else if privatePost == true {
createnewPrivatePost(post: newPost, post_id: post_id, category: category)
}
}
}
func createNewPost(post: Dictionary<String, Any>, post_id: String, category: String) {
let user_id = Auth.auth().currentUser?.uid
let posts = self.ref.child("posts")
let authors = self.ref.child("authors")
let categories = self.ref.child("categories")
let users = self.ref.child("users")
posts.child(post_id).setValue(post)
authors.child(user_id!).child("posts").child(post_id).setValue(post)
categories.child(category).child("posts").child(post_id).setValue(post)
users.child(user_id!).child("posts").child(post_id).setValue(post)
}
I am finding it hard to find the best structure to handle this type of data and an efficient way to screen it to ensure that the content is acceptable (no abusive content etc) Please point me to the best way to handle a blog post type situation like this and how I can best monitor the posts that are set to the database, Thank you.
ios swift

add a comment |
up vote
0
down vote
favorite
I am building an app that allows users to make public posts, you can make a post and view all new posts on the home page. I am struggling to find the best way to efficiently structure the database data and monitor all new posts that are made, as currently if a user is logged in, they can post anything without restriction. I need an effective way to screen posts for content that is uploaded to Firebase, or even a solution that when a new post is made it is sent for approval, rather than being sent on the database straight away. How should I manage this?
Here is the way that posts are uploaded to the database, I use duplicates of this structure also under authors
and categories
, which I am not sure if it is a good way to do it or not, should I be finding a way to have 1 instance of a post on the database, and instead use some kind of IDs to find it in different situations such as by author or by category?
"posts": {
-childGeneratedKey: {
author: "author",
body: "body",
category: "category",
post_date: "1541784510.682485",
post_id: "childAddedID",
title: "post_title",
votes:"0",
}
-childGeneratedKey: {...
}
Here is how I handle posting in-app:
func createPost(title: String, body: String) {
let timeInterval = NSDate().timeIntervalSince1970
let timeDouble = Double(timeInterval)
ref = Database.database().reference()
let posts = self.ref.child("posts")
let post_id = posts.childByAutoId().key!
let category = self.category
let post_date = timeDouble
if postTitle != "" {
let newPost: Dictionary<String, Any> = [
"title": title,
"body": body,
"votes": 0,
"author": username,
"post_id": post_id,
"category": category,
"post_date": post_date
]
if privatePost == false {
createNewPost(post: newPost, post_id: post_id, category: category)
} else if privatePost == true {
createnewPrivatePost(post: newPost, post_id: post_id, category: category)
}
}
}
func createNewPost(post: Dictionary<String, Any>, post_id: String, category: String) {
let user_id = Auth.auth().currentUser?.uid
let posts = self.ref.child("posts")
let authors = self.ref.child("authors")
let categories = self.ref.child("categories")
let users = self.ref.child("users")
posts.child(post_id).setValue(post)
authors.child(user_id!).child("posts").child(post_id).setValue(post)
categories.child(category).child("posts").child(post_id).setValue(post)
users.child(user_id!).child("posts").child(post_id).setValue(post)
}
I am finding it hard to find the best structure to handle this type of data and an efficient way to screen it to ensure that the content is acceptable (no abusive content etc) Please point me to the best way to handle a blog post type situation like this and how I can best monitor the posts that are set to the database, Thank you.
ios swift

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am building an app that allows users to make public posts, you can make a post and view all new posts on the home page. I am struggling to find the best way to efficiently structure the database data and monitor all new posts that are made, as currently if a user is logged in, they can post anything without restriction. I need an effective way to screen posts for content that is uploaded to Firebase, or even a solution that when a new post is made it is sent for approval, rather than being sent on the database straight away. How should I manage this?
Here is the way that posts are uploaded to the database, I use duplicates of this structure also under authors
and categories
, which I am not sure if it is a good way to do it or not, should I be finding a way to have 1 instance of a post on the database, and instead use some kind of IDs to find it in different situations such as by author or by category?
"posts": {
-childGeneratedKey: {
author: "author",
body: "body",
category: "category",
post_date: "1541784510.682485",
post_id: "childAddedID",
title: "post_title",
votes:"0",
}
-childGeneratedKey: {...
}
Here is how I handle posting in-app:
func createPost(title: String, body: String) {
let timeInterval = NSDate().timeIntervalSince1970
let timeDouble = Double(timeInterval)
ref = Database.database().reference()
let posts = self.ref.child("posts")
let post_id = posts.childByAutoId().key!
let category = self.category
let post_date = timeDouble
if postTitle != "" {
let newPost: Dictionary<String, Any> = [
"title": title,
"body": body,
"votes": 0,
"author": username,
"post_id": post_id,
"category": category,
"post_date": post_date
]
if privatePost == false {
createNewPost(post: newPost, post_id: post_id, category: category)
} else if privatePost == true {
createnewPrivatePost(post: newPost, post_id: post_id, category: category)
}
}
}
func createNewPost(post: Dictionary<String, Any>, post_id: String, category: String) {
let user_id = Auth.auth().currentUser?.uid
let posts = self.ref.child("posts")
let authors = self.ref.child("authors")
let categories = self.ref.child("categories")
let users = self.ref.child("users")
posts.child(post_id).setValue(post)
authors.child(user_id!).child("posts").child(post_id).setValue(post)
categories.child(category).child("posts").child(post_id).setValue(post)
users.child(user_id!).child("posts").child(post_id).setValue(post)
}
I am finding it hard to find the best structure to handle this type of data and an efficient way to screen it to ensure that the content is acceptable (no abusive content etc) Please point me to the best way to handle a blog post type situation like this and how I can best monitor the posts that are set to the database, Thank you.
ios swift

I am building an app that allows users to make public posts, you can make a post and view all new posts on the home page. I am struggling to find the best way to efficiently structure the database data and monitor all new posts that are made, as currently if a user is logged in, they can post anything without restriction. I need an effective way to screen posts for content that is uploaded to Firebase, or even a solution that when a new post is made it is sent for approval, rather than being sent on the database straight away. How should I manage this?
Here is the way that posts are uploaded to the database, I use duplicates of this structure also under authors
and categories
, which I am not sure if it is a good way to do it or not, should I be finding a way to have 1 instance of a post on the database, and instead use some kind of IDs to find it in different situations such as by author or by category?
"posts": {
-childGeneratedKey: {
author: "author",
body: "body",
category: "category",
post_date: "1541784510.682485",
post_id: "childAddedID",
title: "post_title",
votes:"0",
}
-childGeneratedKey: {...
}
Here is how I handle posting in-app:
func createPost(title: String, body: String) {
let timeInterval = NSDate().timeIntervalSince1970
let timeDouble = Double(timeInterval)
ref = Database.database().reference()
let posts = self.ref.child("posts")
let post_id = posts.childByAutoId().key!
let category = self.category
let post_date = timeDouble
if postTitle != "" {
let newPost: Dictionary<String, Any> = [
"title": title,
"body": body,
"votes": 0,
"author": username,
"post_id": post_id,
"category": category,
"post_date": post_date
]
if privatePost == false {
createNewPost(post: newPost, post_id: post_id, category: category)
} else if privatePost == true {
createnewPrivatePost(post: newPost, post_id: post_id, category: category)
}
}
}
func createNewPost(post: Dictionary<String, Any>, post_id: String, category: String) {
let user_id = Auth.auth().currentUser?.uid
let posts = self.ref.child("posts")
let authors = self.ref.child("authors")
let categories = self.ref.child("categories")
let users = self.ref.child("users")
posts.child(post_id).setValue(post)
authors.child(user_id!).child("posts").child(post_id).setValue(post)
categories.child(category).child("posts").child(post_id).setValue(post)
users.child(user_id!).child("posts").child(post_id).setValue(post)
}
I am finding it hard to find the best structure to handle this type of data and an efficient way to screen it to ensure that the content is acceptable (no abusive content etc) Please point me to the best way to handle a blog post type situation like this and how I can best monitor the posts that are set to the database, Thank you.
ios swift

ios swift

edited Nov 10 at 11:15


PradyumanDixit
2,4512818
2,4512818
asked Nov 10 at 0:53
Peter Ruppert
79111
79111
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53235073%2fhow-to-structure-and-monitor-user-posts-on-blog-style-app-using-firebase%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IcDIZvT,Il XY8thddEsml6T ek6VR53eLP,a