How to identify calling method from a called method in swift
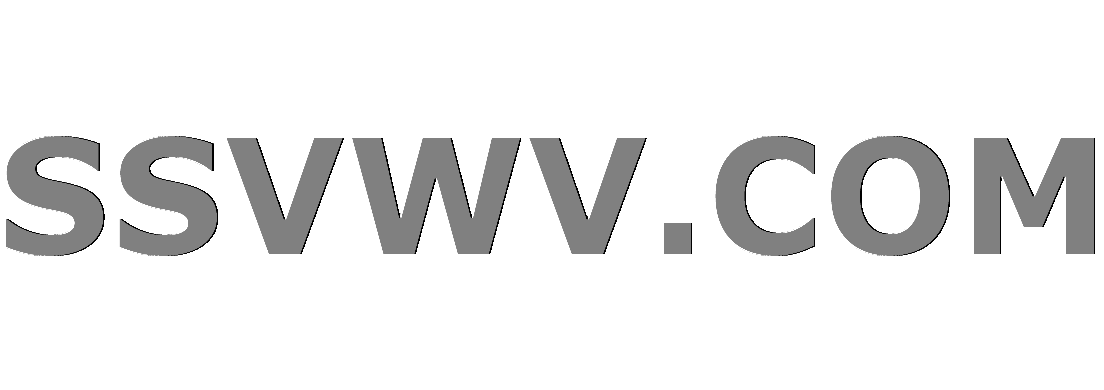
Multi tool use
up vote
0
down vote
favorite
here is a scenario
func callingMethod_A {
self.someCalculation()
}
func callingMethod_B{
self.someCalculation()
}
func someCalculation{
//how to find who called this method? is it callingMethod_A or _B at runtime?
//bla bla
}
how can we get the method name that called it during run time.
thank you.
ios swift
add a comment |
up vote
0
down vote
favorite
here is a scenario
func callingMethod_A {
self.someCalculation()
}
func callingMethod_B{
self.someCalculation()
}
func someCalculation{
//how to find who called this method? is it callingMethod_A or _B at runtime?
//bla bla
}
how can we get the method name that called it during run time.
thank you.
ios swift
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why doessomeCalculation
need to know ifcallingMethod_A
orcallingMethod_B
called it? What happens when you decide to addcallingMethod_C
or changecallingMethod_A
to be callingMethod_A1`?
– dfd
Nov 8 at 13:31
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
here is a scenario
func callingMethod_A {
self.someCalculation()
}
func callingMethod_B{
self.someCalculation()
}
func someCalculation{
//how to find who called this method? is it callingMethod_A or _B at runtime?
//bla bla
}
how can we get the method name that called it during run time.
thank you.
ios swift
here is a scenario
func callingMethod_A {
self.someCalculation()
}
func callingMethod_B{
self.someCalculation()
}
func someCalculation{
//how to find who called this method? is it callingMethod_A or _B at runtime?
//bla bla
}
how can we get the method name that called it during run time.
thank you.
ios swift
ios swift
edited Nov 8 at 13:12


Anbu.karthik
59.1k15121111
59.1k15121111
asked Nov 8 at 13:03


Pavan Kumar
63
63
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why doessomeCalculation
need to know ifcallingMethod_A
orcallingMethod_B
called it? What happens when you decide to addcallingMethod_C
or changecallingMethod_A
to be callingMethod_A1`?
– dfd
Nov 8 at 13:31
add a comment |
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why doessomeCalculation
need to know ifcallingMethod_A
orcallingMethod_B
called it? What happens when you decide to addcallingMethod_C
or changecallingMethod_A
to be callingMethod_A1`?
– dfd
Nov 8 at 13:31
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why does
someCalculation
need to know if callingMethod_A
or callingMethod_B
called it? What happens when you decide to add callingMethod_C
or change callingMethod_A
to be callingMethod_A1`?– dfd
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why does
someCalculation
need to know if callingMethod_A
or callingMethod_B
called it? What happens when you decide to add callingMethod_C
or change callingMethod_A
to be callingMethod_A1`?– dfd
Nov 8 at 13:31
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
I worked out a way to do this, for Swift code anyway:
Define a String
parameter callingFunction
and give it a default value of #function
. Do not pass anything from the caller and the compiler provides the calling function name.
Building on @Anu.Krthik's answer:
func someCalculation (parameter: String, callingMethod: String = #function ) {
print("In `(#function)`, called by `(callingMethod)`")
}
func foo(string: String) {
someCalculation(parameter: string)
}
foo(string: "bar")
The above prints
In `someCalculation(parameter:callingMethod:)`, called by `foo(string:)`
However, beware that this technique can be subverted if the caller provides a value for the callingFunction
parameter. if you call it with:
func foo(string: String) {
someCalculation(parameter: string, callingMethod: "bogusFunctionName()")
}
You get the output
In `someCalculation(parameter:callingMethod:)`, called by `bogusFunctionName()`
instead.
add a comment |
up vote
1
down vote
You can use Thread.callStackSymbols like this
func callingMethod_A() {
self.someCalculation()
}
func callingMethod_B(){
self.someCalculation()
}
func someCalculation(){
let origin = Thread.callStackSymbols
print(origin[0])
print(origin[1])
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I worked out a way to do this, for Swift code anyway:
Define a String
parameter callingFunction
and give it a default value of #function
. Do not pass anything from the caller and the compiler provides the calling function name.
Building on @Anu.Krthik's answer:
func someCalculation (parameter: String, callingMethod: String = #function ) {
print("In `(#function)`, called by `(callingMethod)`")
}
func foo(string: String) {
someCalculation(parameter: string)
}
foo(string: "bar")
The above prints
In `someCalculation(parameter:callingMethod:)`, called by `foo(string:)`
However, beware that this technique can be subverted if the caller provides a value for the callingFunction
parameter. if you call it with:
func foo(string: String) {
someCalculation(parameter: string, callingMethod: "bogusFunctionName()")
}
You get the output
In `someCalculation(parameter:callingMethod:)`, called by `bogusFunctionName()`
instead.
add a comment |
up vote
1
down vote
I worked out a way to do this, for Swift code anyway:
Define a String
parameter callingFunction
and give it a default value of #function
. Do not pass anything from the caller and the compiler provides the calling function name.
Building on @Anu.Krthik's answer:
func someCalculation (parameter: String, callingMethod: String = #function ) {
print("In `(#function)`, called by `(callingMethod)`")
}
func foo(string: String) {
someCalculation(parameter: string)
}
foo(string: "bar")
The above prints
In `someCalculation(parameter:callingMethod:)`, called by `foo(string:)`
However, beware that this technique can be subverted if the caller provides a value for the callingFunction
parameter. if you call it with:
func foo(string: String) {
someCalculation(parameter: string, callingMethod: "bogusFunctionName()")
}
You get the output
In `someCalculation(parameter:callingMethod:)`, called by `bogusFunctionName()`
instead.
add a comment |
up vote
1
down vote
up vote
1
down vote
I worked out a way to do this, for Swift code anyway:
Define a String
parameter callingFunction
and give it a default value of #function
. Do not pass anything from the caller and the compiler provides the calling function name.
Building on @Anu.Krthik's answer:
func someCalculation (parameter: String, callingMethod: String = #function ) {
print("In `(#function)`, called by `(callingMethod)`")
}
func foo(string: String) {
someCalculation(parameter: string)
}
foo(string: "bar")
The above prints
In `someCalculation(parameter:callingMethod:)`, called by `foo(string:)`
However, beware that this technique can be subverted if the caller provides a value for the callingFunction
parameter. if you call it with:
func foo(string: String) {
someCalculation(parameter: string, callingMethod: "bogusFunctionName()")
}
You get the output
In `someCalculation(parameter:callingMethod:)`, called by `bogusFunctionName()`
instead.
I worked out a way to do this, for Swift code anyway:
Define a String
parameter callingFunction
and give it a default value of #function
. Do not pass anything from the caller and the compiler provides the calling function name.
Building on @Anu.Krthik's answer:
func someCalculation (parameter: String, callingMethod: String = #function ) {
print("In `(#function)`, called by `(callingMethod)`")
}
func foo(string: String) {
someCalculation(parameter: string)
}
foo(string: "bar")
The above prints
In `someCalculation(parameter:callingMethod:)`, called by `foo(string:)`
However, beware that this technique can be subverted if the caller provides a value for the callingFunction
parameter. if you call it with:
func foo(string: String) {
someCalculation(parameter: string, callingMethod: "bogusFunctionName()")
}
You get the output
In `someCalculation(parameter:callingMethod:)`, called by `bogusFunctionName()`
instead.
answered Nov 8 at 13:30
Duncan C
90.6k13112192
90.6k13112192
add a comment |
add a comment |
up vote
1
down vote
You can use Thread.callStackSymbols like this
func callingMethod_A() {
self.someCalculation()
}
func callingMethod_B(){
self.someCalculation()
}
func someCalculation(){
let origin = Thread.callStackSymbols
print(origin[0])
print(origin[1])
}
add a comment |
up vote
1
down vote
You can use Thread.callStackSymbols like this
func callingMethod_A() {
self.someCalculation()
}
func callingMethod_B(){
self.someCalculation()
}
func someCalculation(){
let origin = Thread.callStackSymbols
print(origin[0])
print(origin[1])
}
add a comment |
up vote
1
down vote
up vote
1
down vote
You can use Thread.callStackSymbols like this
func callingMethod_A() {
self.someCalculation()
}
func callingMethod_B(){
self.someCalculation()
}
func someCalculation(){
let origin = Thread.callStackSymbols
print(origin[0])
print(origin[1])
}
You can use Thread.callStackSymbols like this
func callingMethod_A() {
self.someCalculation()
}
func callingMethod_B(){
self.someCalculation()
}
func someCalculation(){
let origin = Thread.callStackSymbols
print(origin[0])
print(origin[1])
}
answered Nov 8 at 14:06


Raul Mantilla
595
595
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53208339%2fhow-to-identify-calling-method-from-a-called-method-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sDLtr3Xz9ly hqvU2UEa2I 7RP9M 37EmXZUEg4,x,mcYqR
pass the argument to identify the method
– Anbu.karthik
Nov 8 at 13:09
Add argument, or if it's just for debug purpose, look at the callstack.
– Larme
Nov 8 at 13:10
Larme, how do you look at the call stack in code? Is there a way to get the function signature from the call stack?
– Duncan C
Nov 8 at 13:31
While passing the "caller ID" into a method works, there may well be a better way (delegate, return) to accomplish what you want. Why does
someCalculation
need to know ifcallingMethod_A
orcallingMethod_B
called it? What happens when you decide to addcallingMethod_C
or changecallingMethod_A
to be callingMethod_A1`?– dfd
Nov 8 at 13:31