Why Lua direct returning function result cannot be debugged properly?
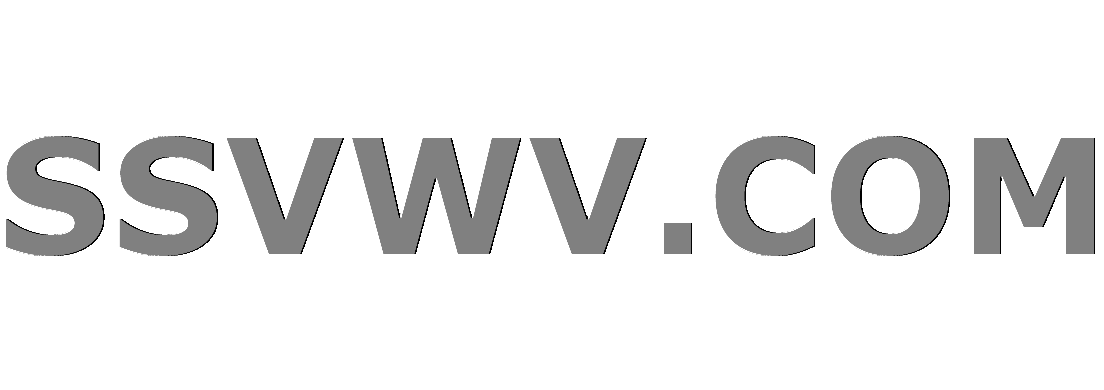
Multi tool use
up vote
1
down vote
favorite
I am trying to do a debugger function, which shows me the exact line the error happens, the function name it happened in and the line where the function is defined. Consider the following code:
function StatusLog(vet,vErr)
local tInfo, sDbg = debug.getinfo(2), ""
sDbg = sDbg.." "..(tInfo.linedefined and "["..tInfo.linedefined.."]" or "X")
sDbg = sDbg..(tInfo.name and tInfo.name or "Main")
sDbg = sDbg..(tInfo.currentline and ("["..tInfo.currentline.."]") or "X")
sDbg = sDbg.."@"..(tInfo.source and (tInfo.source:gsub("^%W", "")) or "N")
print("Debug: "..sDbg)
return vRet
end
local function add(a,b)
if(not a) then return StatusLog(nil, "Missing argument A") end
if(not b) then
StatusLog(nil, "Missing argument B")
return nil
end
return (a + b)
end
print(add(1, 1)) --- Prints: "2" Normal OK
print(add(1, nil)) --- Errors: [11]add[14] OK
print(add(nil, 1)) --- Errors: [0]Main[21] KO
You can clearly see when either of the two arguments is nil
the function add(a,b)
will generate an error, however, when argument B
is missing, the debug information is OK and when argument A
is missing the debug information is kinda broken. It must show the same debug information as B
, but it does not. It is maybe due to the return StatusLog
statement, which breaks the stack.
How can extract the proper debugging information as the function name, the line defined and the line of the error when I'm using the error handling method for argument A
?
debugging error-handling lua dynamic-programming error-messaging
add a comment |
up vote
1
down vote
favorite
I am trying to do a debugger function, which shows me the exact line the error happens, the function name it happened in and the line where the function is defined. Consider the following code:
function StatusLog(vet,vErr)
local tInfo, sDbg = debug.getinfo(2), ""
sDbg = sDbg.." "..(tInfo.linedefined and "["..tInfo.linedefined.."]" or "X")
sDbg = sDbg..(tInfo.name and tInfo.name or "Main")
sDbg = sDbg..(tInfo.currentline and ("["..tInfo.currentline.."]") or "X")
sDbg = sDbg.."@"..(tInfo.source and (tInfo.source:gsub("^%W", "")) or "N")
print("Debug: "..sDbg)
return vRet
end
local function add(a,b)
if(not a) then return StatusLog(nil, "Missing argument A") end
if(not b) then
StatusLog(nil, "Missing argument B")
return nil
end
return (a + b)
end
print(add(1, 1)) --- Prints: "2" Normal OK
print(add(1, nil)) --- Errors: [11]add[14] OK
print(add(nil, 1)) --- Errors: [0]Main[21] KO
You can clearly see when either of the two arguments is nil
the function add(a,b)
will generate an error, however, when argument B
is missing, the debug information is OK and when argument A
is missing the debug information is kinda broken. It must show the same debug information as B
, but it does not. It is maybe due to the return StatusLog
statement, which breaks the stack.
How can extract the proper debugging information as the function name, the line defined and the line of the error when I'm using the error handling method for argument A
?
debugging error-handling lua dynamic-programming error-messaging
1
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
Yep, that's what I thought too. Like when you havereturn
rather than a pointer to a function itdebugs
where the IP goes when thereturn
is executed.
– Деян Добромиров
Nov 8 at 14:37
Because a proper tail call uses no stack space, there is no limit on the number ofnested
tail calls that a program can make. The current stack is reused:http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to do a debugger function, which shows me the exact line the error happens, the function name it happened in and the line where the function is defined. Consider the following code:
function StatusLog(vet,vErr)
local tInfo, sDbg = debug.getinfo(2), ""
sDbg = sDbg.." "..(tInfo.linedefined and "["..tInfo.linedefined.."]" or "X")
sDbg = sDbg..(tInfo.name and tInfo.name or "Main")
sDbg = sDbg..(tInfo.currentline and ("["..tInfo.currentline.."]") or "X")
sDbg = sDbg.."@"..(tInfo.source and (tInfo.source:gsub("^%W", "")) or "N")
print("Debug: "..sDbg)
return vRet
end
local function add(a,b)
if(not a) then return StatusLog(nil, "Missing argument A") end
if(not b) then
StatusLog(nil, "Missing argument B")
return nil
end
return (a + b)
end
print(add(1, 1)) --- Prints: "2" Normal OK
print(add(1, nil)) --- Errors: [11]add[14] OK
print(add(nil, 1)) --- Errors: [0]Main[21] KO
You can clearly see when either of the two arguments is nil
the function add(a,b)
will generate an error, however, when argument B
is missing, the debug information is OK and when argument A
is missing the debug information is kinda broken. It must show the same debug information as B
, but it does not. It is maybe due to the return StatusLog
statement, which breaks the stack.
How can extract the proper debugging information as the function name, the line defined and the line of the error when I'm using the error handling method for argument A
?
debugging error-handling lua dynamic-programming error-messaging
I am trying to do a debugger function, which shows me the exact line the error happens, the function name it happened in and the line where the function is defined. Consider the following code:
function StatusLog(vet,vErr)
local tInfo, sDbg = debug.getinfo(2), ""
sDbg = sDbg.." "..(tInfo.linedefined and "["..tInfo.linedefined.."]" or "X")
sDbg = sDbg..(tInfo.name and tInfo.name or "Main")
sDbg = sDbg..(tInfo.currentline and ("["..tInfo.currentline.."]") or "X")
sDbg = sDbg.."@"..(tInfo.source and (tInfo.source:gsub("^%W", "")) or "N")
print("Debug: "..sDbg)
return vRet
end
local function add(a,b)
if(not a) then return StatusLog(nil, "Missing argument A") end
if(not b) then
StatusLog(nil, "Missing argument B")
return nil
end
return (a + b)
end
print(add(1, 1)) --- Prints: "2" Normal OK
print(add(1, nil)) --- Errors: [11]add[14] OK
print(add(nil, 1)) --- Errors: [0]Main[21] KO
You can clearly see when either of the two arguments is nil
the function add(a,b)
will generate an error, however, when argument B
is missing, the debug information is OK and when argument A
is missing the debug information is kinda broken. It must show the same debug information as B
, but it does not. It is maybe due to the return StatusLog
statement, which breaks the stack.
How can extract the proper debugging information as the function name, the line defined and the line of the error when I'm using the error handling method for argument A
?
debugging error-handling lua dynamic-programming error-messaging
debugging error-handling lua dynamic-programming error-messaging
edited Nov 8 at 14:30
asked Nov 8 at 13:18


Деян Добромиров
3511513
3511513
1
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
Yep, that's what I thought too. Like when you havereturn
rather than a pointer to a function itdebugs
where the IP goes when thereturn
is executed.
– Деян Добромиров
Nov 8 at 14:37
Because a proper tail call uses no stack space, there is no limit on the number ofnested
tail calls that a program can make. The current stack is reused:http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44
add a comment |
1
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
Yep, that's what I thought too. Like when you havereturn
rather than a pointer to a function itdebugs
where the IP goes when thereturn
is executed.
– Деян Добромиров
Nov 8 at 14:37
Because a proper tail call uses no stack space, there is no limit on the number ofnested
tail calls that a program can make. The current stack is reused:http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44
1
1
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
Yep, that's what I thought too. Like when you have
return
rather than a pointer to a function it debugs
where the IP goes when the return
is executed.– Деян Добромиров
Nov 8 at 14:37
Yep, that's what I thought too. Like when you have
return
rather than a pointer to a function it debugs
where the IP goes when the return
is executed.– Деян Добромиров
Nov 8 at 14:37
Because a proper tail call uses no stack space, there is no limit on the number of
nested
tail calls that a program can make. The current stack is reused: http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44
Because a proper tail call uses no stack space, there is no limit on the number of
nested
tail calls that a program can make. The current stack is reused: http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53208572%2fwhy-lua-direct-returning-function-result-cannot-be-debugged-properly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JUXgKTZ bmESWJP,0Av w,NK3Wlwdf,vZTgph
1
This is because of tail call.
– Egor Skriptunoff
Nov 8 at 14:33
Yep, that's what I thought too. Like when you have
return
rather than a pointer to a function itdebugs
where the IP goes when thereturn
is executed.– Деян Добромиров
Nov 8 at 14:37
Because a proper tail call uses no stack space, there is no limit on the number of
nested
tail calls that a program can make. The current stack is reused:http://lua-users.org/wiki/ProperTailRecursion
– Деян Добромиров
Nov 8 at 14:44