Set two cropped image from camera separately in image view (Android Studio)
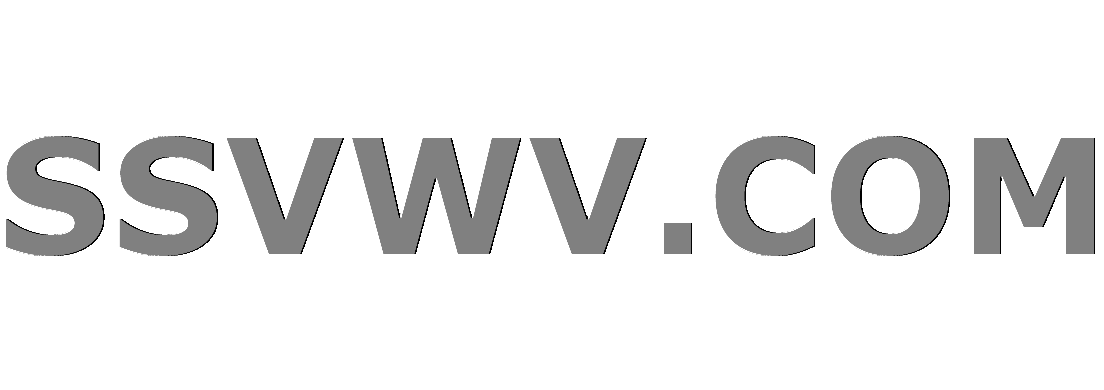
Multi tool use
up vote
2
down vote
favorite
I am try to take two image from camera and crop image then show cropped image in two Imageview
separately.
I have 2 Button
to open camera one for capture first image then cropped to display it in Imageview
and the second do the same thing.
My code in MainActivity
variable in calss
static int CAMERA_REQUEST_CODE = 228;
static int CAMERA_REQUEST_CODE1 = 229;
Uri pictureUri = null;
ImageView iv, iv1;
Button bt, bt1;
onCreate method
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
iv = findViewById(R.id.iv);
bt = findViewById(R.id.bt);
iv1 = findViewById(R.id.iv1);
bt1 = findViewById(R.id.bt1);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera();
}
});
bt1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera1();
}
});
}
invokeCamera() and invokeCamera1() function
public void invokeCamera() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE);
}
public void invokeCamera1() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE1);
}
createImageFile() function
// To create image file in pictures directory
public File createImageFile() {
// the public picture director
File picturesDirectory = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES); // To get pictures directory from android system
// timestamp makes unique name.
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd_HHmmss");
String timestamp = sdf.format(new Date());
// put together the directory and the timestamp to make a unique image location.
File imageFile = new File(picturesDirectory, timestamp + ".jpg");
return imageFile;
}
onActivityResult function
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent
data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) // resultCode: -1
{
if(requestCode == CAMERA_REQUEST_CODE ) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if(requestCode == CAMERA_REQUEST_CODE1)
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
}
if(requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE)
{
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if(resultCode == RESULT_OK)
{
Croppedimage(result, iv); // my problem !
/*
* Here i want to use if or switch statement to can use iv1
for second camera button! HOW?
*
* example
*
* if(for first camera button)
* {
* Croppedimage(result, iv);
* }
*
* if(for second camera button)
* {
* Croppedimage(result, iv1);
* }
*
* */
}
else if(resultCode ==
CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE)
{
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
}
startCropImageActivity function
private void startCropImageActivity(Uri imageUri) {
CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.start(this);
}
Croppedimage function
public void Croppedimage(CropImage.ActivityResult result,ImageView iv)
{
Uri resultUri = null; // get image uri
if (result != null) {
resultUri = result.getUri();
}
//set image to image view
iv.setImageURI(resultUri);
}
____________________________________________________________________________
The problem
The cropped image for second Button
set in first Imageview
.
Need to find way to reach to iv1
in onActivityResult
for second camera Button
.
Any suggestions?
Library use for crop image
THANKS.
java



add a comment |
up vote
2
down vote
favorite
I am try to take two image from camera and crop image then show cropped image in two Imageview
separately.
I have 2 Button
to open camera one for capture first image then cropped to display it in Imageview
and the second do the same thing.
My code in MainActivity
variable in calss
static int CAMERA_REQUEST_CODE = 228;
static int CAMERA_REQUEST_CODE1 = 229;
Uri pictureUri = null;
ImageView iv, iv1;
Button bt, bt1;
onCreate method
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
iv = findViewById(R.id.iv);
bt = findViewById(R.id.bt);
iv1 = findViewById(R.id.iv1);
bt1 = findViewById(R.id.bt1);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera();
}
});
bt1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera1();
}
});
}
invokeCamera() and invokeCamera1() function
public void invokeCamera() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE);
}
public void invokeCamera1() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE1);
}
createImageFile() function
// To create image file in pictures directory
public File createImageFile() {
// the public picture director
File picturesDirectory = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES); // To get pictures directory from android system
// timestamp makes unique name.
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd_HHmmss");
String timestamp = sdf.format(new Date());
// put together the directory and the timestamp to make a unique image location.
File imageFile = new File(picturesDirectory, timestamp + ".jpg");
return imageFile;
}
onActivityResult function
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent
data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) // resultCode: -1
{
if(requestCode == CAMERA_REQUEST_CODE ) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if(requestCode == CAMERA_REQUEST_CODE1)
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
}
if(requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE)
{
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if(resultCode == RESULT_OK)
{
Croppedimage(result, iv); // my problem !
/*
* Here i want to use if or switch statement to can use iv1
for second camera button! HOW?
*
* example
*
* if(for first camera button)
* {
* Croppedimage(result, iv);
* }
*
* if(for second camera button)
* {
* Croppedimage(result, iv1);
* }
*
* */
}
else if(resultCode ==
CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE)
{
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
}
startCropImageActivity function
private void startCropImageActivity(Uri imageUri) {
CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.start(this);
}
Croppedimage function
public void Croppedimage(CropImage.ActivityResult result,ImageView iv)
{
Uri resultUri = null; // get image uri
if (result != null) {
resultUri = result.getUri();
}
//set image to image view
iv.setImageURI(resultUri);
}
____________________________________________________________________________
The problem
The cropped image for second Button
set in first Imageview
.
Need to find way to reach to iv1
in onActivityResult
for second camera Button
.
Any suggestions?
Library use for crop image
THANKS.
java



add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am try to take two image from camera and crop image then show cropped image in two Imageview
separately.
I have 2 Button
to open camera one for capture first image then cropped to display it in Imageview
and the second do the same thing.
My code in MainActivity
variable in calss
static int CAMERA_REQUEST_CODE = 228;
static int CAMERA_REQUEST_CODE1 = 229;
Uri pictureUri = null;
ImageView iv, iv1;
Button bt, bt1;
onCreate method
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
iv = findViewById(R.id.iv);
bt = findViewById(R.id.bt);
iv1 = findViewById(R.id.iv1);
bt1 = findViewById(R.id.bt1);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera();
}
});
bt1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera1();
}
});
}
invokeCamera() and invokeCamera1() function
public void invokeCamera() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE);
}
public void invokeCamera1() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE1);
}
createImageFile() function
// To create image file in pictures directory
public File createImageFile() {
// the public picture director
File picturesDirectory = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES); // To get pictures directory from android system
// timestamp makes unique name.
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd_HHmmss");
String timestamp = sdf.format(new Date());
// put together the directory and the timestamp to make a unique image location.
File imageFile = new File(picturesDirectory, timestamp + ".jpg");
return imageFile;
}
onActivityResult function
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent
data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) // resultCode: -1
{
if(requestCode == CAMERA_REQUEST_CODE ) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if(requestCode == CAMERA_REQUEST_CODE1)
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
}
if(requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE)
{
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if(resultCode == RESULT_OK)
{
Croppedimage(result, iv); // my problem !
/*
* Here i want to use if or switch statement to can use iv1
for second camera button! HOW?
*
* example
*
* if(for first camera button)
* {
* Croppedimage(result, iv);
* }
*
* if(for second camera button)
* {
* Croppedimage(result, iv1);
* }
*
* */
}
else if(resultCode ==
CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE)
{
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
}
startCropImageActivity function
private void startCropImageActivity(Uri imageUri) {
CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.start(this);
}
Croppedimage function
public void Croppedimage(CropImage.ActivityResult result,ImageView iv)
{
Uri resultUri = null; // get image uri
if (result != null) {
resultUri = result.getUri();
}
//set image to image view
iv.setImageURI(resultUri);
}
____________________________________________________________________________
The problem
The cropped image for second Button
set in first Imageview
.
Need to find way to reach to iv1
in onActivityResult
for second camera Button
.
Any suggestions?
Library use for crop image
THANKS.
java



I am try to take two image from camera and crop image then show cropped image in two Imageview
separately.
I have 2 Button
to open camera one for capture first image then cropped to display it in Imageview
and the second do the same thing.
My code in MainActivity
variable in calss
static int CAMERA_REQUEST_CODE = 228;
static int CAMERA_REQUEST_CODE1 = 229;
Uri pictureUri = null;
ImageView iv, iv1;
Button bt, bt1;
onCreate method
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
iv = findViewById(R.id.iv);
bt = findViewById(R.id.bt);
iv1 = findViewById(R.id.iv1);
bt1 = findViewById(R.id.bt1);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera();
}
});
bt1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
invokeCamera1();
}
});
}
invokeCamera() and invokeCamera1() function
public void invokeCamera() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE);
}
public void invokeCamera1() {
// get a file reference
pictureUri = FileProvider.getUriForFile(this, getApplicationContext().getPackageName(), createImageFile()); // Make Uri file example file://storage/emulated/0/Pictures/Civil_ID20180924_180619.jpg
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); // Go to camera
// tell the camera where to save the image.
intent.putExtra(MediaStore.EXTRA_OUTPUT, pictureUri);
// tell the camera to request WRITE permission.
intent.setFlags(Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
startActivityForResult(intent, CAMERA_REQUEST_CODE1);
}
createImageFile() function
// To create image file in pictures directory
public File createImageFile() {
// the public picture director
File picturesDirectory = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES); // To get pictures directory from android system
// timestamp makes unique name.
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd_HHmmss");
String timestamp = sdf.format(new Date());
// put together the directory and the timestamp to make a unique image location.
File imageFile = new File(picturesDirectory, timestamp + ".jpg");
return imageFile;
}
onActivityResult function
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent
data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) // resultCode: -1
{
if(requestCode == CAMERA_REQUEST_CODE ) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if(requestCode == CAMERA_REQUEST_CODE1)
{
Uri picUri = pictureUri;
startCropImageActivity(picUri);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
}
if(requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE)
{
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if(resultCode == RESULT_OK)
{
Croppedimage(result, iv); // my problem !
/*
* Here i want to use if or switch statement to can use iv1
for second camera button! HOW?
*
* example
*
* if(for first camera button)
* {
* Croppedimage(result, iv);
* }
*
* if(for second camera button)
* {
* Croppedimage(result, iv1);
* }
*
* */
}
else if(resultCode ==
CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE)
{
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
}
startCropImageActivity function
private void startCropImageActivity(Uri imageUri) {
CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.start(this);
}
Croppedimage function
public void Croppedimage(CropImage.ActivityResult result,ImageView iv)
{
Uri resultUri = null; // get image uri
if (result != null) {
resultUri = result.getUri();
}
//set image to image view
iv.setImageURI(resultUri);
}
____________________________________________________________________________
The problem
The cropped image for second Button
set in first Imageview
.
Need to find way to reach to iv1
in onActivityResult
for second camera Button
.
Any suggestions?
Library use for crop image
THANKS.
java



java



edited Nov 8 at 13:51
asked Nov 8 at 13:15


SWAT 10101
135
135
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Looking in the issues on the GitHub respository I found this one, that seems similar to yours, you can set a custom request code when starting the crop activity.
So you can start the activity with 2 different request codes and check which one has been used on onActivityResult
private static final RC_CROP = 100;
private static final RC_CROP1 = 200;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) // resultCode: -1
{
if (requestCode == CAMERA_REQUEST_CODE) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == CAMERA_REQUEST_CODE1) {
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP1);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == RC_CROP) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on first ImageView
}
if (requestCode == RC_CROP1) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on second ImageView
}
} else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE) {
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
private void startCropImageActivity(Uri imageUri, int requestCode) {
Intent vCropIntent = CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.getIntent(this);
startActivityForResult(vCropIntent, requestCode)
}
I suggest also to use a switch statement when checking the requestCode
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Looking in the issues on the GitHub respository I found this one, that seems similar to yours, you can set a custom request code when starting the crop activity.
So you can start the activity with 2 different request codes and check which one has been used on onActivityResult
private static final RC_CROP = 100;
private static final RC_CROP1 = 200;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) // resultCode: -1
{
if (requestCode == CAMERA_REQUEST_CODE) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == CAMERA_REQUEST_CODE1) {
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP1);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == RC_CROP) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on first ImageView
}
if (requestCode == RC_CROP1) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on second ImageView
}
} else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE) {
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
private void startCropImageActivity(Uri imageUri, int requestCode) {
Intent vCropIntent = CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.getIntent(this);
startActivityForResult(vCropIntent, requestCode)
}
I suggest also to use a switch statement when checking the requestCode
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
add a comment |
up vote
0
down vote
accepted
Looking in the issues on the GitHub respository I found this one, that seems similar to yours, you can set a custom request code when starting the crop activity.
So you can start the activity with 2 different request codes and check which one has been used on onActivityResult
private static final RC_CROP = 100;
private static final RC_CROP1 = 200;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) // resultCode: -1
{
if (requestCode == CAMERA_REQUEST_CODE) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == CAMERA_REQUEST_CODE1) {
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP1);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == RC_CROP) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on first ImageView
}
if (requestCode == RC_CROP1) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on second ImageView
}
} else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE) {
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
private void startCropImageActivity(Uri imageUri, int requestCode) {
Intent vCropIntent = CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.getIntent(this);
startActivityForResult(vCropIntent, requestCode)
}
I suggest also to use a switch statement when checking the requestCode
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Looking in the issues on the GitHub respository I found this one, that seems similar to yours, you can set a custom request code when starting the crop activity.
So you can start the activity with 2 different request codes and check which one has been used on onActivityResult
private static final RC_CROP = 100;
private static final RC_CROP1 = 200;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) // resultCode: -1
{
if (requestCode == CAMERA_REQUEST_CODE) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == CAMERA_REQUEST_CODE1) {
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP1);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == RC_CROP) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on first ImageView
}
if (requestCode == RC_CROP1) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on second ImageView
}
} else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE) {
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
private void startCropImageActivity(Uri imageUri, int requestCode) {
Intent vCropIntent = CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.getIntent(this);
startActivityForResult(vCropIntent, requestCode)
}
I suggest also to use a switch statement when checking the requestCode
Looking in the issues on the GitHub respository I found this one, that seems similar to yours, you can set a custom request code when starting the crop activity.
So you can start the activity with 2 different request codes and check which one has been used on onActivityResult
private static final RC_CROP = 100;
private static final RC_CROP1 = 200;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) // resultCode: -1
{
if (requestCode == CAMERA_REQUEST_CODE) // requestCode: 288
{
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP);
Toast.makeText(MainActivity.this, "Image 1 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == CAMERA_REQUEST_CODE1) {
Uri picUri = pictureUri;
startCropImageActivity(picUri, RC_CROP1);
Toast.makeText(MainActivity.this, "Image 2 save",
Toast.LENGTH_SHORT).show();
}
if (requestCode == RC_CROP) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on first ImageView
}
if (requestCode == RC_CROP1) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
//put image on second ImageView
}
} else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE) {
// if there is any error show it
Exception error = result.getError();
Toast.makeText(this, "" + error, Toast.LENGTH_LONG).show();
}
}
private void startCropImageActivity(Uri imageUri, int requestCode) {
Intent vCropIntent = CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setMultiTouchEnabled(true)
.getIntent(this);
startActivityForResult(vCropIntent, requestCode)
}
I suggest also to use a switch statement when checking the requestCode
answered Nov 8 at 14:53
Jameido
640614
640614
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
add a comment |
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Thank you my dear it work very well.
– SWAT 10101
Nov 8 at 15:09
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
Glad to hear that, so please mark the answer as accepted
– Jameido
Nov 8 at 15:24
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53208521%2fset-two-cropped-image-from-camera-separately-in-image-view-android-studio%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N0AFrKn0bv8VNU9PSk8,I4Q6DHQ5MPufCK,q0,kl2NHtEleYF 0Q,mp,05yVw4SFSZsW,2aj1TvNWs2Ib,9k79 XfMv6go