LINQ: Get all objects with a matching string property from an incoming IEnumerable in a performant way
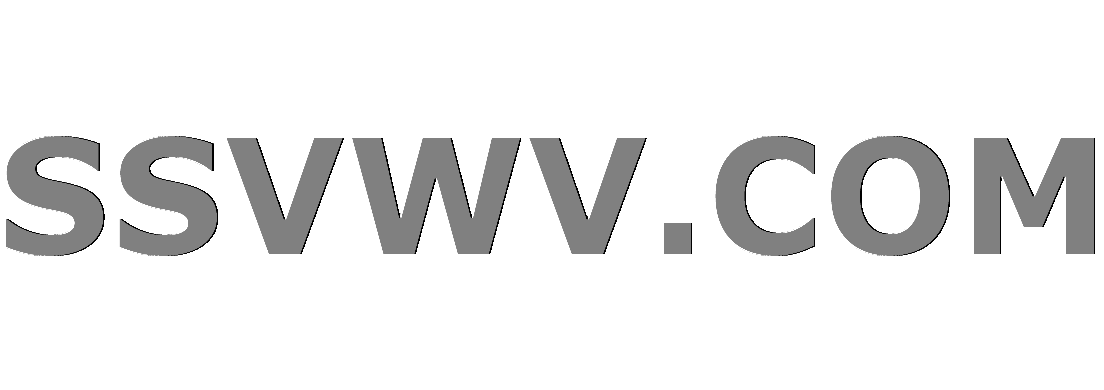
Multi tool use
up vote
0
down vote
favorite
I just saw this piece of code and asked myself how it can be improved to reduce the amount of queries. Tried a few LINQ statements but could not find an answer.
public static Dictionary<string, Computer> GetComputer(IEnumerable<string> workStations)
{
var dict = new Dictionary<string, Computer>();
using (var db = new ComputerContext())
{
foreach (var workStation in workStations)
{
var t = db.Computers.FirstOrDefault(o => o.Id.Equals(workStation));
if (!dict.ContainsKey(workStation))
{
dict.Add(workStation, t);
}
}
return dict;
}
}
When trying someting like this:
var computers = db.Computers.Where(x => workStations.Select(y => y).Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
Intellisense is telling me "Suspicious comparison: there is no type in the solution which is inherited from both
'System.Collections.Generic.IEnumerable' and 'string'" which
leds to an Exception "Cannot compare elements of type
'System.Collections.Generic.IEnumerable`1[[System.String, mscorlib,
Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089]]'.
Only primitive types, enumeration types and entity types are
supported."
c# .net performance entity-framework linq
add a comment |
up vote
0
down vote
favorite
I just saw this piece of code and asked myself how it can be improved to reduce the amount of queries. Tried a few LINQ statements but could not find an answer.
public static Dictionary<string, Computer> GetComputer(IEnumerable<string> workStations)
{
var dict = new Dictionary<string, Computer>();
using (var db = new ComputerContext())
{
foreach (var workStation in workStations)
{
var t = db.Computers.FirstOrDefault(o => o.Id.Equals(workStation));
if (!dict.ContainsKey(workStation))
{
dict.Add(workStation, t);
}
}
return dict;
}
}
When trying someting like this:
var computers = db.Computers.Where(x => workStations.Select(y => y).Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
Intellisense is telling me "Suspicious comparison: there is no type in the solution which is inherited from both
'System.Collections.Generic.IEnumerable' and 'string'" which
leds to an Exception "Cannot compare elements of type
'System.Collections.Generic.IEnumerable`1[[System.String, mscorlib,
Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089]]'.
Only primitive types, enumeration types and entity types are
supported."
c# .net performance entity-framework linq
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Yes, I got that. the problem is with the usage ofSelect
. it basically does nothing in your code. it is like if your wroteworkStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I just saw this piece of code and asked myself how it can be improved to reduce the amount of queries. Tried a few LINQ statements but could not find an answer.
public static Dictionary<string, Computer> GetComputer(IEnumerable<string> workStations)
{
var dict = new Dictionary<string, Computer>();
using (var db = new ComputerContext())
{
foreach (var workStation in workStations)
{
var t = db.Computers.FirstOrDefault(o => o.Id.Equals(workStation));
if (!dict.ContainsKey(workStation))
{
dict.Add(workStation, t);
}
}
return dict;
}
}
When trying someting like this:
var computers = db.Computers.Where(x => workStations.Select(y => y).Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
Intellisense is telling me "Suspicious comparison: there is no type in the solution which is inherited from both
'System.Collections.Generic.IEnumerable' and 'string'" which
leds to an Exception "Cannot compare elements of type
'System.Collections.Generic.IEnumerable`1[[System.String, mscorlib,
Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089]]'.
Only primitive types, enumeration types and entity types are
supported."
c# .net performance entity-framework linq
I just saw this piece of code and asked myself how it can be improved to reduce the amount of queries. Tried a few LINQ statements but could not find an answer.
public static Dictionary<string, Computer> GetComputer(IEnumerable<string> workStations)
{
var dict = new Dictionary<string, Computer>();
using (var db = new ComputerContext())
{
foreach (var workStation in workStations)
{
var t = db.Computers.FirstOrDefault(o => o.Id.Equals(workStation));
if (!dict.ContainsKey(workStation))
{
dict.Add(workStation, t);
}
}
return dict;
}
}
When trying someting like this:
var computers = db.Computers.Where(x => workStations.Select(y => y).Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
Intellisense is telling me "Suspicious comparison: there is no type in the solution which is inherited from both
'System.Collections.Generic.IEnumerable' and 'string'" which
leds to an Exception "Cannot compare elements of type
'System.Collections.Generic.IEnumerable`1[[System.String, mscorlib,
Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089]]'.
Only primitive types, enumeration types and entity types are
supported."
c# .net performance entity-framework linq
c# .net performance entity-framework linq
edited Nov 8 at 8:49


TheGeneral
24.7k53162
24.7k53162
asked Nov 8 at 8:47
bslein
1279
1279
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Yes, I got that. the problem is with the usage ofSelect
. it basically does nothing in your code. it is like if your wroteworkStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53
add a comment |
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Yes, I got that. the problem is with the usage ofSelect
. it basically does nothing in your code. it is like if your wroteworkStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Yes, I got that. the problem is with the usage of
Select
. it basically does nothing in your code. it is like if your wrote workStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53
Yes, I got that. the problem is with the usage of
Select
. it basically does nothing in your code. it is like if your wrote workStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
The problem is in your query. Try this code:
var computers = db.Computers.Where(x => workStations.Any(y => y.Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The problem is in your query. Try this code:
var computers = db.Computers.Where(x => workStations.Any(y => y.Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
add a comment |
up vote
1
down vote
accepted
The problem is in your query. Try this code:
var computers = db.Computers.Where(x => workStations.Any(y => y.Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The problem is in your query. Try this code:
var computers = db.Computers.Where(x => workStations.Any(y => y.Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
The problem is in your query. Try this code:
var computers = db.Computers.Where(x => workStations.Any(y => y.Equals(x.Id)).ToList();
foreach (var computer in computers)
{
if (!dict.ContainsKey(computer.Id))
{
dict.Add(computer.Id, computer);
}
}
edited Nov 8 at 8:56


Bizhan
7,55063254
7,55063254
answered Nov 8 at 8:51
Khai Nguyen
1966
1966
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
add a comment |
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
It works but unfortunately the old code is twice as fast (measured with Stopwatch)
– bslein
Nov 8 at 9:03
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
What is the old code? This is just fixing your error. Basically, it looks query 10 times if workstation has 10 items
– Khai Nguyen
Nov 8 at 9:08
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
I meant the code which i posted in my question with multiple queries is twice as fast as the LINQ code.
– bslein
Nov 8 at 10:03
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53204196%2flinq-get-all-objects-with-a-matching-string-property-from-an-incoming-ienumerab%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
uQex rAM J5hwDrC0NACxKQ2 66SH7xL4 p5GUYbDw9zaHuPDqy,CjFLS,zWW8
Id is just an example (it's a string in this example)
– bslein
Nov 8 at 8:51
Yes, I got that. the problem is with the usage of
Select
. it basically does nothing in your code. it is like if your wroteworkStations.Equals(x.Id)
– Bizhan
Nov 8 at 8:53