React - can't stream video from webcam
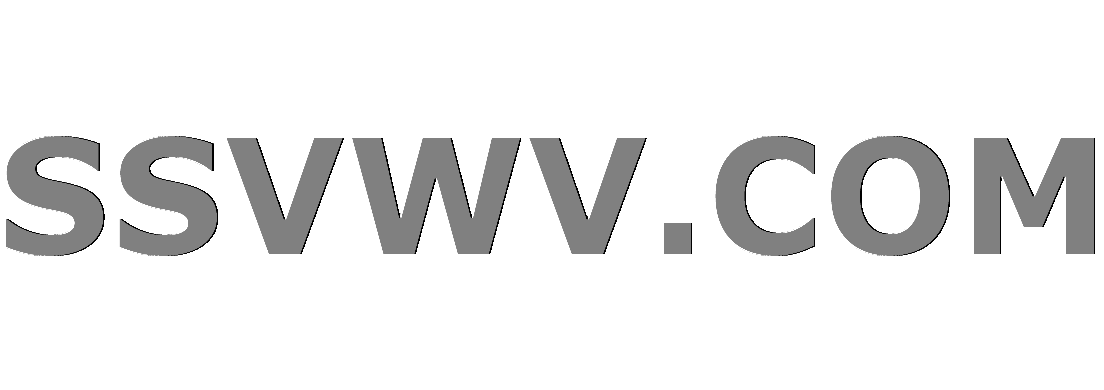
Multi tool use
up vote
0
down vote
favorite
I'm trying to fix my Component to streaming data from webcam. It renders successfully and successfully gets access to webcam. But I have no idea why video tag do not plays anything. How to fix this? What am I missing?
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.state = {
src: null
}
this.videoRef = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.setState({src: stream}))
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={() => this.videoRef.srcObject = this.state.src}
width={this.props.width}
height={this.props.height}
autoPlay="autoplay"
title={this.props.title}></video>
}
}
reactjs html5-video webcam
add a comment |
up vote
0
down vote
favorite
I'm trying to fix my Component to streaming data from webcam. It renders successfully and successfully gets access to webcam. But I have no idea why video tag do not plays anything. How to fix this? What am I missing?
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.state = {
src: null
}
this.videoRef = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.setState({src: stream}))
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={() => this.videoRef.srcObject = this.state.src}
width={this.props.width}
height={this.props.height}
autoPlay="autoplay"
title={this.props.title}></video>
}
}
reactjs html5-video webcam
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to fix my Component to streaming data from webcam. It renders successfully and successfully gets access to webcam. But I have no idea why video tag do not plays anything. How to fix this? What am I missing?
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.state = {
src: null
}
this.videoRef = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.setState({src: stream}))
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={() => this.videoRef.srcObject = this.state.src}
width={this.props.width}
height={this.props.height}
autoPlay="autoplay"
title={this.props.title}></video>
}
}
reactjs html5-video webcam
I'm trying to fix my Component to streaming data from webcam. It renders successfully and successfully gets access to webcam. But I have no idea why video tag do not plays anything. How to fix this? What am I missing?
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.state = {
src: null
}
this.videoRef = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.setState({src: stream}))
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={() => this.videoRef.srcObject = this.state.src}
width={this.props.width}
height={this.props.height}
autoPlay="autoplay"
title={this.props.title}></video>
}
}
reactjs html5-video webcam
reactjs html5-video webcam
asked Nov 9 at 12:55
Sergio Ivanuzzo
1,02931137
1,02931137
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
The ref
is not correctly used in this line:
ref={() => this.videoRef.srcObject = this.state.src}
As in your code just sets the src
to the videoRef
which is not initialized so it never gets to the video tag.
You may try:
ref={this.videoRef.srcObject}
And in the componentDidMount:
.then(stream => {this.videoRef.srcObject = stream})
Or simply:
ref={(e) => e.srcObject = this.state.src}
Thanks for your answer. I've tried to update myrender()
method according to your example, but when I changedref
toref={(e) => e.srcObject = this.state.src}
I got next error:TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates
– Sergio Ivanuzzo
Nov 9 at 15:57
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
add a comment |
up vote
1
down vote
accepted
Well, I have found what was wrong. According to docs I need to use current
property to make the node accessible. So, the full working example of my Webcam component:
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.videoTag = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.videoTag.current.srcObject = stream)
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={this.videoTag}
width={this.props.width}
height={this.props.height}
autoPlay
title={this.props.title}></video>
}
}
this.setState
was removed in prior of direct srcObject
changing from promise, but I'm not sure if this React way. Maybe, more correctly is moving this.videoTag.current.srcObject = stream
code as setState callback?
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use thesrc
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.
– Xizario
Nov 9 at 16:05
add a comment |
up vote
0
down vote
i am also facing same error when using same above code it is showing " _this2.video is undefined; can't access its "current" property" and All things is right , take vedio permission but it is not showing vedio in my page.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
The ref
is not correctly used in this line:
ref={() => this.videoRef.srcObject = this.state.src}
As in your code just sets the src
to the videoRef
which is not initialized so it never gets to the video tag.
You may try:
ref={this.videoRef.srcObject}
And in the componentDidMount:
.then(stream => {this.videoRef.srcObject = stream})
Or simply:
ref={(e) => e.srcObject = this.state.src}
Thanks for your answer. I've tried to update myrender()
method according to your example, but when I changedref
toref={(e) => e.srcObject = this.state.src}
I got next error:TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates
– Sergio Ivanuzzo
Nov 9 at 15:57
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
add a comment |
up vote
1
down vote
The ref
is not correctly used in this line:
ref={() => this.videoRef.srcObject = this.state.src}
As in your code just sets the src
to the videoRef
which is not initialized so it never gets to the video tag.
You may try:
ref={this.videoRef.srcObject}
And in the componentDidMount:
.then(stream => {this.videoRef.srcObject = stream})
Or simply:
ref={(e) => e.srcObject = this.state.src}
Thanks for your answer. I've tried to update myrender()
method according to your example, but when I changedref
toref={(e) => e.srcObject = this.state.src}
I got next error:TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates
– Sergio Ivanuzzo
Nov 9 at 15:57
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
add a comment |
up vote
1
down vote
up vote
1
down vote
The ref
is not correctly used in this line:
ref={() => this.videoRef.srcObject = this.state.src}
As in your code just sets the src
to the videoRef
which is not initialized so it never gets to the video tag.
You may try:
ref={this.videoRef.srcObject}
And in the componentDidMount:
.then(stream => {this.videoRef.srcObject = stream})
Or simply:
ref={(e) => e.srcObject = this.state.src}
The ref
is not correctly used in this line:
ref={() => this.videoRef.srcObject = this.state.src}
As in your code just sets the src
to the videoRef
which is not initialized so it never gets to the video tag.
You may try:
ref={this.videoRef.srcObject}
And in the componentDidMount:
.then(stream => {this.videoRef.srcObject = stream})
Or simply:
ref={(e) => e.srcObject = this.state.src}
answered Nov 9 at 13:58


Xizario
1767
1767
Thanks for your answer. I've tried to update myrender()
method according to your example, but when I changedref
toref={(e) => e.srcObject = this.state.src}
I got next error:TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates
– Sergio Ivanuzzo
Nov 9 at 15:57
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
add a comment |
Thanks for your answer. I've tried to update myrender()
method according to your example, but when I changedref
toref={(e) => e.srcObject = this.state.src}
I got next error:TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates
– Sergio Ivanuzzo
Nov 9 at 15:57
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
Thanks for your answer. I've tried to update my
render()
method according to your example, but when I changed ref
to ref={(e) => e.srcObject = this.state.src}
I got next error: TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
Thanks for your answer. I've tried to update my
render()
method according to your example, but when I changed ref
to ref={(e) => e.srcObject = this.state.src}
I got next error: TypeError: Cannot set property 'srcObject' of null
– Sergio Ivanuzzo
Nov 9 at 14:11
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
And because of this error Component do not renders
– Sergio Ivanuzzo
Nov 9 at 14:13
btw,
ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates– Sergio Ivanuzzo
Nov 9 at 15:57
btw,
ref={(e) => e.srcObject = this.state.src}
harms performance, because each re-render new funcion creates– Sergio Ivanuzzo
Nov 9 at 15:57
1
1
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
Well to be fair, if you are loading a video which already uses tons of resources, and you also not going to re-render the component many times... the creation of the function is the latest one to care optimization for.
– Xizario
Nov 9 at 16:03
add a comment |
up vote
1
down vote
accepted
Well, I have found what was wrong. According to docs I need to use current
property to make the node accessible. So, the full working example of my Webcam component:
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.videoTag = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.videoTag.current.srcObject = stream)
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={this.videoTag}
width={this.props.width}
height={this.props.height}
autoPlay
title={this.props.title}></video>
}
}
this.setState
was removed in prior of direct srcObject
changing from promise, but I'm not sure if this React way. Maybe, more correctly is moving this.videoTag.current.srcObject = stream
code as setState callback?
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use thesrc
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.
– Xizario
Nov 9 at 16:05
add a comment |
up vote
1
down vote
accepted
Well, I have found what was wrong. According to docs I need to use current
property to make the node accessible. So, the full working example of my Webcam component:
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.videoTag = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.videoTag.current.srcObject = stream)
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={this.videoTag}
width={this.props.width}
height={this.props.height}
autoPlay
title={this.props.title}></video>
}
}
this.setState
was removed in prior of direct srcObject
changing from promise, but I'm not sure if this React way. Maybe, more correctly is moving this.videoTag.current.srcObject = stream
code as setState callback?
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use thesrc
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.
– Xizario
Nov 9 at 16:05
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Well, I have found what was wrong. According to docs I need to use current
property to make the node accessible. So, the full working example of my Webcam component:
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.videoTag = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.videoTag.current.srcObject = stream)
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={this.videoTag}
width={this.props.width}
height={this.props.height}
autoPlay
title={this.props.title}></video>
}
}
this.setState
was removed in prior of direct srcObject
changing from promise, but I'm not sure if this React way. Maybe, more correctly is moving this.videoTag.current.srcObject = stream
code as setState callback?
Well, I have found what was wrong. According to docs I need to use current
property to make the node accessible. So, the full working example of my Webcam component:
export class WebcamStream extends React.Component {
constructor(props) {
super(props);
this.videoTag = React.createRef()
}
componentDidMount() {
// getting access to webcam
navigator.mediaDevices
.getUserMedia({video: true})
.then(stream => this.videoTag.current.srcObject = stream)
.catch(console.log);
}
render() {
return <video id={this.props.id}
ref={this.videoTag}
width={this.props.width}
height={this.props.height}
autoPlay
title={this.props.title}></video>
}
}
this.setState
was removed in prior of direct srcObject
changing from promise, but I'm not sure if this React way. Maybe, more correctly is moving this.videoTag.current.srcObject = stream
code as setState callback?
answered Nov 9 at 15:53
Sergio Ivanuzzo
1,02931137
1,02931137
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use thesrc
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.
– Xizario
Nov 9 at 16:05
add a comment |
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use thesrc
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.
– Xizario
Nov 9 at 16:05
1
1
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use the
src
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.– Xizario
Nov 9 at 16:05
It looks clean enough to say it is react way. If you are using the set-state to store the src, you may use the
src
prop of the video tag directly without ref callback. Another solution is to render the video tag on demand after the src is already available. Render null if src is not available, and then render video if src is available.– Xizario
Nov 9 at 16:05
add a comment |
up vote
0
down vote
i am also facing same error when using same above code it is showing " _this2.video is undefined; can't access its "current" property" and All things is right , take vedio permission but it is not showing vedio in my page.
add a comment |
up vote
0
down vote
i am also facing same error when using same above code it is showing " _this2.video is undefined; can't access its "current" property" and All things is right , take vedio permission but it is not showing vedio in my page.
add a comment |
up vote
0
down vote
up vote
0
down vote
i am also facing same error when using same above code it is showing " _this2.video is undefined; can't access its "current" property" and All things is right , take vedio permission but it is not showing vedio in my page.
i am also facing same error when using same above code it is showing " _this2.video is undefined; can't access its "current" property" and All things is right , take vedio permission but it is not showing vedio in my page.
answered Nov 23 at 18:41
khushahal sharma
13
13
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226099%2freact-cant-stream-video-from-webcam%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xO1b3Ggvr,q,Aky iapl,ybavKKZHfNe4JtPxx,2kr,AX,xbUSIIgKHwx