React router v4 parameter going as undefined
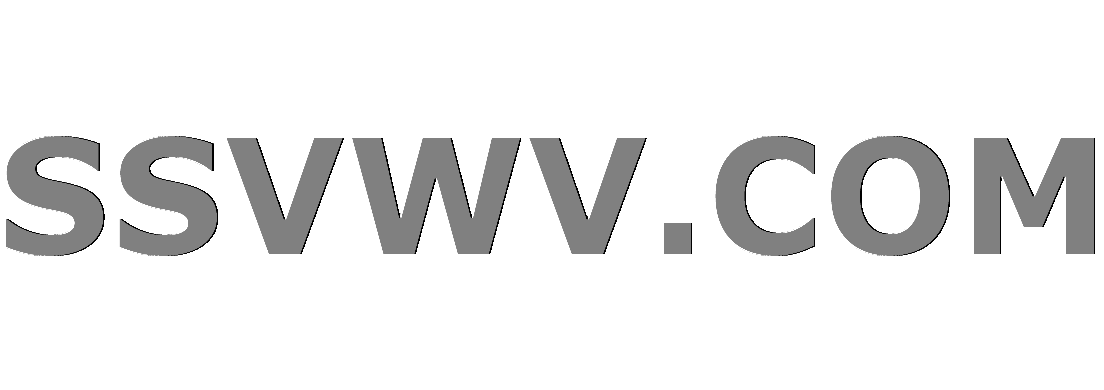
Multi tool use
up vote
1
down vote
favorite
I have a route config like this:
/${URL.PRODUCT}/:category/:brand/:flavour/
, with exact={true}
and it loads the Product component.
On Product component I have this:
constructor(props) {
super(props)
this.state = {
selectedCategory: props.match.params.category || '',
selectedFlavour: props.match.params.flavour || '',
selectedBrand: props.match.params.brand || '',
}
s("PARAMS", JSON.stringify(props.match.params)); //console on server
c("PARAMS", JSON.stringify(props.match.params)); // console on browser
}
Now, on some other component I have a click event, which does this:
clickHandler = (cardData) => {
let path = `/${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/${cardData.slug}`;
cardData.type === CONTENT_TYPE.FLAVOUR && !!cardData.slug && this.props.history.push(path);
}
And cardData
looks like this:
{
id: 3
image: "/some/imagepath/"
name: "Diet Coke"
selectedCard: "cocacola"
selectedCategory: "softdrinnk"
slug: "dietcoke"
type: "flavour"
}
Its resulting in something like this: /product/softdrinnk/cocacola/dietcoke
Now the problem: As I am hitting this.props.history.push()
its making a server call (which it should not) also, with a wrong URL: /product/softdrinnk/cocacola/undefined
This is what my console looks like on client:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"dietcoke"}
And this is what I receive on server:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"undefined"}
I have spend the whole day figuring out why this behaviour is happening.
Update:
Even if when I hardcoded the URL: /${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/dietcoke
, Its ignoring the last parameter and still and passing undefined
to the server
And when I hit browser refresh on this route, it works normally and loads all the data
reactjs react-router-v4
add a comment |
up vote
1
down vote
favorite
I have a route config like this:
/${URL.PRODUCT}/:category/:brand/:flavour/
, with exact={true}
and it loads the Product component.
On Product component I have this:
constructor(props) {
super(props)
this.state = {
selectedCategory: props.match.params.category || '',
selectedFlavour: props.match.params.flavour || '',
selectedBrand: props.match.params.brand || '',
}
s("PARAMS", JSON.stringify(props.match.params)); //console on server
c("PARAMS", JSON.stringify(props.match.params)); // console on browser
}
Now, on some other component I have a click event, which does this:
clickHandler = (cardData) => {
let path = `/${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/${cardData.slug}`;
cardData.type === CONTENT_TYPE.FLAVOUR && !!cardData.slug && this.props.history.push(path);
}
And cardData
looks like this:
{
id: 3
image: "/some/imagepath/"
name: "Diet Coke"
selectedCard: "cocacola"
selectedCategory: "softdrinnk"
slug: "dietcoke"
type: "flavour"
}
Its resulting in something like this: /product/softdrinnk/cocacola/dietcoke
Now the problem: As I am hitting this.props.history.push()
its making a server call (which it should not) also, with a wrong URL: /product/softdrinnk/cocacola/undefined
This is what my console looks like on client:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"dietcoke"}
And this is what I receive on server:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"undefined"}
I have spend the whole day figuring out why this behaviour is happening.
Update:
Even if when I hardcoded the URL: /${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/dietcoke
, Its ignoring the last parameter and still and passing undefined
to the server
And when I hit browser refresh on this route, it works normally and loads all the data
reactjs react-router-v4
What doescardData
look like? You haveselectedCategory
,selectedFlavour
,selectedBrand
in state, but onlyselectedCategory
of those incardData
?
– Tholle
Nov 9 at 13:31
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
Thanks. Is your click handler on a button inside a form? You might have to doclickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.
– Tholle
Nov 9 at 13:36
1
That's not in a form, its just another component with anonCLick
event
– Saurabh Sharma
Nov 9 at 13:37
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a route config like this:
/${URL.PRODUCT}/:category/:brand/:flavour/
, with exact={true}
and it loads the Product component.
On Product component I have this:
constructor(props) {
super(props)
this.state = {
selectedCategory: props.match.params.category || '',
selectedFlavour: props.match.params.flavour || '',
selectedBrand: props.match.params.brand || '',
}
s("PARAMS", JSON.stringify(props.match.params)); //console on server
c("PARAMS", JSON.stringify(props.match.params)); // console on browser
}
Now, on some other component I have a click event, which does this:
clickHandler = (cardData) => {
let path = `/${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/${cardData.slug}`;
cardData.type === CONTENT_TYPE.FLAVOUR && !!cardData.slug && this.props.history.push(path);
}
And cardData
looks like this:
{
id: 3
image: "/some/imagepath/"
name: "Diet Coke"
selectedCard: "cocacola"
selectedCategory: "softdrinnk"
slug: "dietcoke"
type: "flavour"
}
Its resulting in something like this: /product/softdrinnk/cocacola/dietcoke
Now the problem: As I am hitting this.props.history.push()
its making a server call (which it should not) also, with a wrong URL: /product/softdrinnk/cocacola/undefined
This is what my console looks like on client:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"dietcoke"}
And this is what I receive on server:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"undefined"}
I have spend the whole day figuring out why this behaviour is happening.
Update:
Even if when I hardcoded the URL: /${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/dietcoke
, Its ignoring the last parameter and still and passing undefined
to the server
And when I hit browser refresh on this route, it works normally and loads all the data
reactjs react-router-v4
I have a route config like this:
/${URL.PRODUCT}/:category/:brand/:flavour/
, with exact={true}
and it loads the Product component.
On Product component I have this:
constructor(props) {
super(props)
this.state = {
selectedCategory: props.match.params.category || '',
selectedFlavour: props.match.params.flavour || '',
selectedBrand: props.match.params.brand || '',
}
s("PARAMS", JSON.stringify(props.match.params)); //console on server
c("PARAMS", JSON.stringify(props.match.params)); // console on browser
}
Now, on some other component I have a click event, which does this:
clickHandler = (cardData) => {
let path = `/${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/${cardData.slug}`;
cardData.type === CONTENT_TYPE.FLAVOUR && !!cardData.slug && this.props.history.push(path);
}
And cardData
looks like this:
{
id: 3
image: "/some/imagepath/"
name: "Diet Coke"
selectedCard: "cocacola"
selectedCategory: "softdrinnk"
slug: "dietcoke"
type: "flavour"
}
Its resulting in something like this: /product/softdrinnk/cocacola/dietcoke
Now the problem: As I am hitting this.props.history.push()
its making a server call (which it should not) also, with a wrong URL: /product/softdrinnk/cocacola/undefined
This is what my console looks like on client:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"dietcoke"}
And this is what I receive on server:
PARAMS {"category":"softdrinnk","brand":"cocacola","flavour":"undefined"}
I have spend the whole day figuring out why this behaviour is happening.
Update:
Even if when I hardcoded the URL: /${URL.PRODUCT}/${cardData.selectedCategory}/${cardData.selectedCard}/dietcoke
, Its ignoring the last parameter and still and passing undefined
to the server
And when I hit browser refresh on this route, it works normally and loads all the data
reactjs react-router-v4
reactjs react-router-v4
edited Nov 9 at 13:48
asked Nov 9 at 13:25
Saurabh Sharma
1,74421034
1,74421034
What doescardData
look like? You haveselectedCategory
,selectedFlavour
,selectedBrand
in state, but onlyselectedCategory
of those incardData
?
– Tholle
Nov 9 at 13:31
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
Thanks. Is your click handler on a button inside a form? You might have to doclickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.
– Tholle
Nov 9 at 13:36
1
That's not in a form, its just another component with anonCLick
event
– Saurabh Sharma
Nov 9 at 13:37
add a comment |
What doescardData
look like? You haveselectedCategory
,selectedFlavour
,selectedBrand
in state, but onlyselectedCategory
of those incardData
?
– Tholle
Nov 9 at 13:31
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
Thanks. Is your click handler on a button inside a form? You might have to doclickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.
– Tholle
Nov 9 at 13:36
1
That's not in a form, its just another component with anonCLick
event
– Saurabh Sharma
Nov 9 at 13:37
What does
cardData
look like? You have selectedCategory
, selectedFlavour
, selectedBrand
in state, but only selectedCategory
of those in cardData
?– Tholle
Nov 9 at 13:31
What does
cardData
look like? You have selectedCategory
, selectedFlavour
, selectedBrand
in state, but only selectedCategory
of those in cardData
?– Tholle
Nov 9 at 13:31
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
Thanks. Is your click handler on a button inside a form? You might have to do
clickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.– Tholle
Nov 9 at 13:36
Thanks. Is your click handler on a button inside a form? You might have to do
clickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.– Tholle
Nov 9 at 13:36
1
1
That's not in a form, its just another component with an
onCLick
event– Saurabh Sharma
Nov 9 at 13:37
That's not in a form, its just another component with an
onCLick
event– Saurabh Sharma
Nov 9 at 13:37
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226578%2freact-router-v4-parameter-going-as-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s518d,r3OicBmXNFtxqw05V5ygQrfOOw 7oFkrae0ZAI,ms57km9Xi lo4sln0mV,4h,EW4
What does
cardData
look like? You haveselectedCategory
,selectedFlavour
,selectedBrand
in state, but onlyselectedCategory
of those incardData
?– Tholle
Nov 9 at 13:31
@Tholle updated that in my question
– Saurabh Sharma
Nov 9 at 13:35
Thanks. Is your click handler on a button inside a form? You might have to do
clickHandler = (event, cardData) => { event.preventDefault(); ... }
if that's the case.– Tholle
Nov 9 at 13:36
1
That's not in a form, its just another component with an
onCLick
event– Saurabh Sharma
Nov 9 at 13:37