Immutable local values in c# - a specific use case
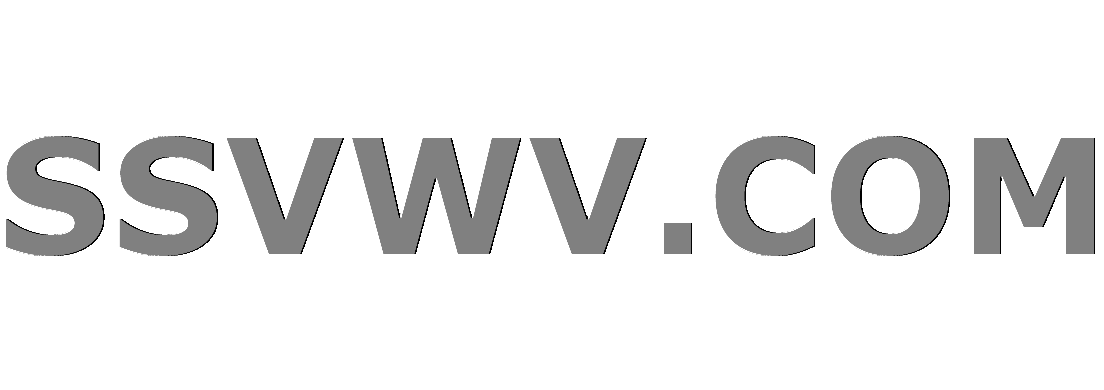
Multi tool use
up vote
4
down vote
favorite
I know that this general topic has been discussed here before. What I am interested in is if there is a good solution for my specific case:
I have a command line tool like this (simplified):
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
string inputFolder = args[0];
string outputFolder = args[1];
// ...
}
I assign names to the argument values to make the code more readable. I would also like to express that these values will not be modified later.
Neither const
nor readonly
can be used here because the values is not known at compile time and because they are local 'variables' and not class members.
So how could I make this code more expressive and readable?
c# const immutability
add a comment |
up vote
4
down vote
favorite
I know that this general topic has been discussed here before. What I am interested in is if there is a good solution for my specific case:
I have a command line tool like this (simplified):
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
string inputFolder = args[0];
string outputFolder = args[1];
// ...
}
I assign names to the argument values to make the code more readable. I would also like to express that these values will not be modified later.
Neither const
nor readonly
can be used here because the values is not known at compile time and because they are local 'variables' and not class members.
So how could I make this code more expressive and readable?
c# const immutability
1
I don't think there is a keyword for that. How about making astruct
or aclass
where you pass your variables toconstructor
and expose them withpublic get
ters? One could argue that this will make your program even more readable. :)
– Eric
Nov 9 at 13:19
1
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28
add a comment |
up vote
4
down vote
favorite
up vote
4
down vote
favorite
I know that this general topic has been discussed here before. What I am interested in is if there is a good solution for my specific case:
I have a command line tool like this (simplified):
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
string inputFolder = args[0];
string outputFolder = args[1];
// ...
}
I assign names to the argument values to make the code more readable. I would also like to express that these values will not be modified later.
Neither const
nor readonly
can be used here because the values is not known at compile time and because they are local 'variables' and not class members.
So how could I make this code more expressive and readable?
c# const immutability
I know that this general topic has been discussed here before. What I am interested in is if there is a good solution for my specific case:
I have a command line tool like this (simplified):
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
string inputFolder = args[0];
string outputFolder = args[1];
// ...
}
I assign names to the argument values to make the code more readable. I would also like to express that these values will not be modified later.
Neither const
nor readonly
can be used here because the values is not known at compile time and because they are local 'variables' and not class members.
So how could I make this code more expressive and readable?
c# const immutability
c# const immutability
edited Nov 9 at 13:23
asked Nov 9 at 13:16


Frank Puffer
6,64511035
6,64511035
1
I don't think there is a keyword for that. How about making astruct
or aclass
where you pass your variables toconstructor
and expose them withpublic get
ters? One could argue that this will make your program even more readable. :)
– Eric
Nov 9 at 13:19
1
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28
add a comment |
1
I don't think there is a keyword for that. How about making astruct
or aclass
where you pass your variables toconstructor
and expose them withpublic get
ters? One could argue that this will make your program even more readable. :)
– Eric
Nov 9 at 13:19
1
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28
1
1
I don't think there is a keyword for that. How about making a
struct
or a class
where you pass your variables to constructor
and expose them with public get
ters? One could argue that this will make your program even more readable. :)– Eric
Nov 9 at 13:19
I don't think there is a keyword for that. How about making a
struct
or a class
where you pass your variables to constructor
and expose them with public get
ters? One could argue that this will make your program even more readable. :)– Eric
Nov 9 at 13:19
1
1
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28
add a comment |
4 Answers
4
active
oldest
votes
up vote
3
down vote
My proposition is creating a class holding your variables
public class Immutable
{
public Immutable(string args)
{
InputFolder = args[0];
OutputFolder = args[1];
}
public readonly string InputFolder;
public readonly string OutputFolder;
}
then
var m = new Immutable(args)
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
You do not have bothreadonly
andget;
. Either it is a field, or it is a property, never a hybrid.
– Jeppe Stig Nielsen
Nov 9 at 13:29
2
Sure you could do that, but then inMain()
you could still change the instance ofImmutable
to reference a different instance later in the method, so I'm not sure how much this really helps.
– Matthew Watson
Nov 9 at 13:38
|
show 1 more comment
up vote
2
down vote
How about the C# 7.2 ref readonly
?
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
ref readonly var inputFolder = ref args[0];
ref readonly var outputFolder = ref args[1];
}
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
add a comment |
up vote
1
down vote
You could create some sort of Inputs class where you could parse the array into its parts and make the properties that expose the parsed values readonly. Simple Example:
public class Inputs {
private string _args;
public string InputFolder { get { return _args[0]; } }
public string OutputFolder { get { return _args[1]; } }
public Inputs(string args) { _args = args.Clone(); }
}
add a comment |
up vote
1
down vote
I'd do something like this:
public class ImmutableObject
{
public ImmutableObject(string inputFolder, string outputFolder)
{
InputFolder = inputFolder;
OutputFolder = outputFolder;
}
public string InputFolder {get;}
public string OutputFolder {get;}
}
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
var folders = new ImmutableObject(args[0], args[1]);
// ...
}
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
My proposition is creating a class holding your variables
public class Immutable
{
public Immutable(string args)
{
InputFolder = args[0];
OutputFolder = args[1];
}
public readonly string InputFolder;
public readonly string OutputFolder;
}
then
var m = new Immutable(args)
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
You do not have bothreadonly
andget;
. Either it is a field, or it is a property, never a hybrid.
– Jeppe Stig Nielsen
Nov 9 at 13:29
2
Sure you could do that, but then inMain()
you could still change the instance ofImmutable
to reference a different instance later in the method, so I'm not sure how much this really helps.
– Matthew Watson
Nov 9 at 13:38
|
show 1 more comment
up vote
3
down vote
My proposition is creating a class holding your variables
public class Immutable
{
public Immutable(string args)
{
InputFolder = args[0];
OutputFolder = args[1];
}
public readonly string InputFolder;
public readonly string OutputFolder;
}
then
var m = new Immutable(args)
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
You do not have bothreadonly
andget;
. Either it is a field, or it is a property, never a hybrid.
– Jeppe Stig Nielsen
Nov 9 at 13:29
2
Sure you could do that, but then inMain()
you could still change the instance ofImmutable
to reference a different instance later in the method, so I'm not sure how much this really helps.
– Matthew Watson
Nov 9 at 13:38
|
show 1 more comment
up vote
3
down vote
up vote
3
down vote
My proposition is creating a class holding your variables
public class Immutable
{
public Immutable(string args)
{
InputFolder = args[0];
OutputFolder = args[1];
}
public readonly string InputFolder;
public readonly string OutputFolder;
}
then
var m = new Immutable(args)
My proposition is creating a class holding your variables
public class Immutable
{
public Immutable(string args)
{
InputFolder = args[0];
OutputFolder = args[1];
}
public readonly string InputFolder;
public readonly string OutputFolder;
}
then
var m = new Immutable(args)
edited Nov 9 at 13:31
answered Nov 9 at 13:22
Antoine V
4,9472424
4,9472424
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
You do not have bothreadonly
andget;
. Either it is a field, or it is a property, never a hybrid.
– Jeppe Stig Nielsen
Nov 9 at 13:29
2
Sure you could do that, but then inMain()
you could still change the instance ofImmutable
to reference a different instance later in the method, so I'm not sure how much this really helps.
– Matthew Watson
Nov 9 at 13:38
|
show 1 more comment
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
You do not have bothreadonly
andget;
. Either it is a field, or it is a property, never a hybrid.
– Jeppe Stig Nielsen
Nov 9 at 13:29
2
Sure you could do that, but then inMain()
you could still change the instance ofImmutable
to reference a different instance later in the method, so I'm not sure how much this really helps.
– Matthew Watson
Nov 9 at 13:38
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
This is how I'd do it. But I would accept and array inside the constructor so that the method signature doesn't need to get changed every time a variable is added.
– Eric
Nov 9 at 13:24
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
Yes, but I don't think it makes the code more readable.
– Frank Puffer
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
@Eric good observation
– Antoine V
Nov 9 at 13:26
2
2
You do not have both
readonly
and get;
. Either it is a field, or it is a property, never a hybrid.– Jeppe Stig Nielsen
Nov 9 at 13:29
You do not have both
readonly
and get;
. Either it is a field, or it is a property, never a hybrid.– Jeppe Stig Nielsen
Nov 9 at 13:29
2
2
Sure you could do that, but then in
Main()
you could still change the instance of Immutable
to reference a different instance later in the method, so I'm not sure how much this really helps.– Matthew Watson
Nov 9 at 13:38
Sure you could do that, but then in
Main()
you could still change the instance of Immutable
to reference a different instance later in the method, so I'm not sure how much this really helps.– Matthew Watson
Nov 9 at 13:38
|
show 1 more comment
up vote
2
down vote
How about the C# 7.2 ref readonly
?
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
ref readonly var inputFolder = ref args[0];
ref readonly var outputFolder = ref args[1];
}
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
add a comment |
up vote
2
down vote
How about the C# 7.2 ref readonly
?
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
ref readonly var inputFolder = ref args[0];
ref readonly var outputFolder = ref args[1];
}
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
add a comment |
up vote
2
down vote
up vote
2
down vote
How about the C# 7.2 ref readonly
?
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
ref readonly var inputFolder = ref args[0];
ref readonly var outputFolder = ref args[1];
}
How about the C# 7.2 ref readonly
?
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
ref readonly var inputFolder = ref args[0];
ref readonly var outputFolder = ref args[1];
}
edited Nov 9 at 14:25
answered Nov 9 at 14:00
Magnus
35.4k75490
35.4k75490
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
add a comment |
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
This comes closest to what I was looking for. Still a bit cryptic for such a simple thing.
– Frank Puffer
Nov 9 at 17:20
add a comment |
up vote
1
down vote
You could create some sort of Inputs class where you could parse the array into its parts and make the properties that expose the parsed values readonly. Simple Example:
public class Inputs {
private string _args;
public string InputFolder { get { return _args[0]; } }
public string OutputFolder { get { return _args[1]; } }
public Inputs(string args) { _args = args.Clone(); }
}
add a comment |
up vote
1
down vote
You could create some sort of Inputs class where you could parse the array into its parts and make the properties that expose the parsed values readonly. Simple Example:
public class Inputs {
private string _args;
public string InputFolder { get { return _args[0]; } }
public string OutputFolder { get { return _args[1]; } }
public Inputs(string args) { _args = args.Clone(); }
}
add a comment |
up vote
1
down vote
up vote
1
down vote
You could create some sort of Inputs class where you could parse the array into its parts and make the properties that expose the parsed values readonly. Simple Example:
public class Inputs {
private string _args;
public string InputFolder { get { return _args[0]; } }
public string OutputFolder { get { return _args[1]; } }
public Inputs(string args) { _args = args.Clone(); }
}
You could create some sort of Inputs class where you could parse the array into its parts and make the properties that expose the parsed values readonly. Simple Example:
public class Inputs {
private string _args;
public string InputFolder { get { return _args[0]; } }
public string OutputFolder { get { return _args[1]; } }
public Inputs(string args) { _args = args.Clone(); }
}
edited Nov 9 at 13:21
answered Nov 9 at 13:20
Ryan Pierce Williams
43719
43719
add a comment |
add a comment |
up vote
1
down vote
I'd do something like this:
public class ImmutableObject
{
public ImmutableObject(string inputFolder, string outputFolder)
{
InputFolder = inputFolder;
OutputFolder = outputFolder;
}
public string InputFolder {get;}
public string OutputFolder {get;}
}
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
var folders = new ImmutableObject(args[0], args[1]);
// ...
}
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
add a comment |
up vote
1
down vote
I'd do something like this:
public class ImmutableObject
{
public ImmutableObject(string inputFolder, string outputFolder)
{
InputFolder = inputFolder;
OutputFolder = outputFolder;
}
public string InputFolder {get;}
public string OutputFolder {get;}
}
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
var folders = new ImmutableObject(args[0], args[1]);
// ...
}
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
add a comment |
up vote
1
down vote
up vote
1
down vote
I'd do something like this:
public class ImmutableObject
{
public ImmutableObject(string inputFolder, string outputFolder)
{
InputFolder = inputFolder;
OutputFolder = outputFolder;
}
public string InputFolder {get;}
public string OutputFolder {get;}
}
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
var folders = new ImmutableObject(args[0], args[1]);
// ...
}
I'd do something like this:
public class ImmutableObject
{
public ImmutableObject(string inputFolder, string outputFolder)
{
InputFolder = inputFolder;
OutputFolder = outputFolder;
}
public string InputFolder {get;}
public string OutputFolder {get;}
}
static void Main(string args)
{
if (args.Length < 2)
{
Console.WriteLine("Usage: MyTool <InputFolder> <OutputFolder>");
return;
}
var folders = new ImmutableObject(args[0], args[1]);
// ...
}
answered Nov 9 at 13:25
Victor Procure
758416
758416
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
add a comment |
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
Bah @antoine-v beat me to it
– Victor Procure
Nov 9 at 13:27
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226428%2fimmutable-local-values-in-c-sharp-a-specific-use-case%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1nENyGG5zC1euTknpl04sgspZlgi,2Y,2YzDQ
1
I don't think there is a keyword for that. How about making a
struct
or aclass
where you pass your variables toconstructor
and expose them withpublic get
ters? One could argue that this will make your program even more readable. :)– Eric
Nov 9 at 13:19
1
not an answer as it is doesnt currently exist, but could in the future infoq.com/news/2017/04/CSharp-Readonly-Locals
– Dave
Nov 9 at 13:28