Format validation error message as nested associative array
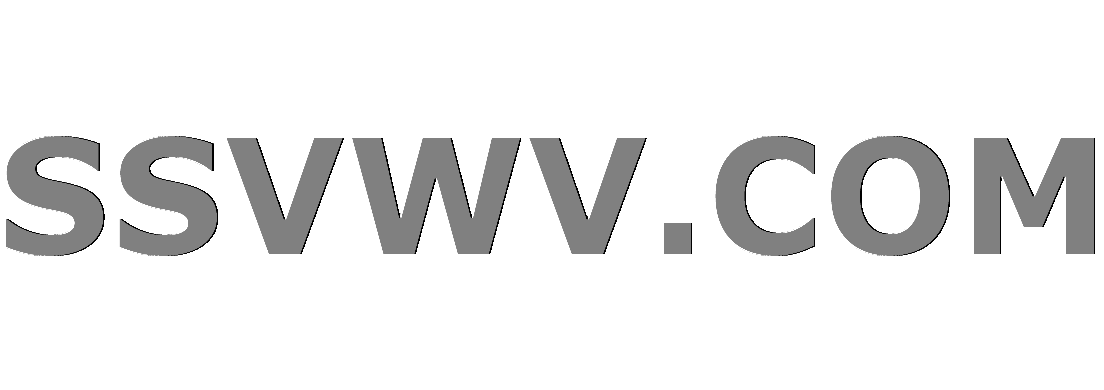
Multi tool use
up vote
0
down vote
favorite
I have a Json object of data as shown below
{
"name": "something",
"location": {
"city": "some where",
"country": "some where",
}
}
Rule used to validate Request is
[
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]
Which returns error message like
{
"name": [
"The name field is required."
],
"location.city": [
"The location.city field is required."
],
"location.county": [
"The location.country field is required."
]
}
How can I format error message as a nested array like the Request.
{
"name": [
"The name field is required."
],
"location": {
"city": [
"The city field is required"
],
"country": [
"The country field is required"
]
}
}
Any default methods available ?
I am using IlluminateFoundationHttpFormRequest
laravel validation laravel-5.7
add a comment |
up vote
0
down vote
favorite
I have a Json object of data as shown below
{
"name": "something",
"location": {
"city": "some where",
"country": "some where",
}
}
Rule used to validate Request is
[
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]
Which returns error message like
{
"name": [
"The name field is required."
],
"location.city": [
"The location.city field is required."
],
"location.county": [
"The location.country field is required."
]
}
How can I format error message as a nested array like the Request.
{
"name": [
"The name field is required."
],
"location": {
"city": [
"The city field is required"
],
"country": [
"The country field is required"
]
}
}
Any default methods available ?
I am using IlluminateFoundationHttpFormRequest
laravel validation laravel-5.7
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a Json object of data as shown below
{
"name": "something",
"location": {
"city": "some where",
"country": "some where",
}
}
Rule used to validate Request is
[
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]
Which returns error message like
{
"name": [
"The name field is required."
],
"location.city": [
"The location.city field is required."
],
"location.county": [
"The location.country field is required."
]
}
How can I format error message as a nested array like the Request.
{
"name": [
"The name field is required."
],
"location": {
"city": [
"The city field is required"
],
"country": [
"The country field is required"
]
}
}
Any default methods available ?
I am using IlluminateFoundationHttpFormRequest
laravel validation laravel-5.7
I have a Json object of data as shown below
{
"name": "something",
"location": {
"city": "some where",
"country": "some where",
}
}
Rule used to validate Request is
[
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]
Which returns error message like
{
"name": [
"The name field is required."
],
"location.city": [
"The location.city field is required."
],
"location.county": [
"The location.country field is required."
]
}
How can I format error message as a nested array like the Request.
{
"name": [
"The name field is required."
],
"location": {
"city": [
"The city field is required"
],
"country": [
"The country field is required"
]
}
}
Any default methods available ?
I am using IlluminateFoundationHttpFormRequest
laravel validation laravel-5.7
laravel validation laravel-5.7
edited yesterday
asked yesterday


Ebin Manuval
605722
605722
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
In your case , you need to build the error message yourself. you can still use the default messages in the ressources/lang/en/validation
messages file.
$validator = Validator::make($request->all(), [
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]);
if ($validator->fails()) {
return response()->json($yourOwnFormat,422);
//you can use $validator->errors() to build it
}
add a comment |
up vote
0
down vote
Is it? Laravel Documentation- Customizing the Error Messages
public function messages()
{
return [
'location.city' => 'The city field is required',
'location.county' => 'The county field is required',
];
}
It will only change the message part likeThe city field is required
I need to change the keylocation.city
. Please have a look at my response sample
– Ebin Manuval
yesterday
add a comment |
up vote
0
down vote
For those who looking for the solution This is how I implemented
<?php
namespace AppHttpRequests;
use IlluminateContractsValidationValidator;
use IlluminateFoundationHttpFormRequest;
use IlluminateHttpExceptionsHttpResponseException;
class UserStoreRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required',
'location.city' => 'required'
'location.country' => 'required'
];
}
public function attributes()
{
return [
'location.city' => 'City'
'location.country' => 'Country'
];
}
protected function failedValidation(Validator $validator)
{
$errors = $validator->errors()->getMessages();
$errors_formated = array();
foreach ($errors as $key => $value) {
array_set($errors_formated, $key, $value);
}
throw new HttpResponseException(response()->json(['error' => $errors_formated], 422));
}
}
The result of $validator->errors()->getMessages()
is just like array_dot()
helper function result. So I did the Opposite of array_dot()
, also altered my attribute name into pretty name
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
In your case , you need to build the error message yourself. you can still use the default messages in the ressources/lang/en/validation
messages file.
$validator = Validator::make($request->all(), [
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]);
if ($validator->fails()) {
return response()->json($yourOwnFormat,422);
//you can use $validator->errors() to build it
}
add a comment |
up vote
1
down vote
In your case , you need to build the error message yourself. you can still use the default messages in the ressources/lang/en/validation
messages file.
$validator = Validator::make($request->all(), [
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]);
if ($validator->fails()) {
return response()->json($yourOwnFormat,422);
//you can use $validator->errors() to build it
}
add a comment |
up vote
1
down vote
up vote
1
down vote
In your case , you need to build the error message yourself. you can still use the default messages in the ressources/lang/en/validation
messages file.
$validator = Validator::make($request->all(), [
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]);
if ($validator->fails()) {
return response()->json($yourOwnFormat,422);
//you can use $validator->errors() to build it
}
In your case , you need to build the error message yourself. you can still use the default messages in the ressources/lang/en/validation
messages file.
$validator = Validator::make($request->all(), [
'name' => 'required',
'location.city' => 'required',
'location.country' => 'required'
]);
if ($validator->fails()) {
return response()->json($yourOwnFormat,422);
//you can use $validator->errors() to build it
}
answered yesterday


N69S
766311
766311
add a comment |
add a comment |
up vote
0
down vote
Is it? Laravel Documentation- Customizing the Error Messages
public function messages()
{
return [
'location.city' => 'The city field is required',
'location.county' => 'The county field is required',
];
}
It will only change the message part likeThe city field is required
I need to change the keylocation.city
. Please have a look at my response sample
– Ebin Manuval
yesterday
add a comment |
up vote
0
down vote
Is it? Laravel Documentation- Customizing the Error Messages
public function messages()
{
return [
'location.city' => 'The city field is required',
'location.county' => 'The county field is required',
];
}
It will only change the message part likeThe city field is required
I need to change the keylocation.city
. Please have a look at my response sample
– Ebin Manuval
yesterday
add a comment |
up vote
0
down vote
up vote
0
down vote
Is it? Laravel Documentation- Customizing the Error Messages
public function messages()
{
return [
'location.city' => 'The city field is required',
'location.county' => 'The county field is required',
];
}
Is it? Laravel Documentation- Customizing the Error Messages
public function messages()
{
return [
'location.city' => 'The city field is required',
'location.county' => 'The county field is required',
];
}
answered yesterday
Mou Hsiao
344
344
It will only change the message part likeThe city field is required
I need to change the keylocation.city
. Please have a look at my response sample
– Ebin Manuval
yesterday
add a comment |
It will only change the message part likeThe city field is required
I need to change the keylocation.city
. Please have a look at my response sample
– Ebin Manuval
yesterday
It will only change the message part like
The city field is required
I need to change the key location.city
. Please have a look at my response sample– Ebin Manuval
yesterday
It will only change the message part like
The city field is required
I need to change the key location.city
. Please have a look at my response sample– Ebin Manuval
yesterday
add a comment |
up vote
0
down vote
For those who looking for the solution This is how I implemented
<?php
namespace AppHttpRequests;
use IlluminateContractsValidationValidator;
use IlluminateFoundationHttpFormRequest;
use IlluminateHttpExceptionsHttpResponseException;
class UserStoreRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required',
'location.city' => 'required'
'location.country' => 'required'
];
}
public function attributes()
{
return [
'location.city' => 'City'
'location.country' => 'Country'
];
}
protected function failedValidation(Validator $validator)
{
$errors = $validator->errors()->getMessages();
$errors_formated = array();
foreach ($errors as $key => $value) {
array_set($errors_formated, $key, $value);
}
throw new HttpResponseException(response()->json(['error' => $errors_formated], 422));
}
}
The result of $validator->errors()->getMessages()
is just like array_dot()
helper function result. So I did the Opposite of array_dot()
, also altered my attribute name into pretty name
add a comment |
up vote
0
down vote
For those who looking for the solution This is how I implemented
<?php
namespace AppHttpRequests;
use IlluminateContractsValidationValidator;
use IlluminateFoundationHttpFormRequest;
use IlluminateHttpExceptionsHttpResponseException;
class UserStoreRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required',
'location.city' => 'required'
'location.country' => 'required'
];
}
public function attributes()
{
return [
'location.city' => 'City'
'location.country' => 'Country'
];
}
protected function failedValidation(Validator $validator)
{
$errors = $validator->errors()->getMessages();
$errors_formated = array();
foreach ($errors as $key => $value) {
array_set($errors_formated, $key, $value);
}
throw new HttpResponseException(response()->json(['error' => $errors_formated], 422));
}
}
The result of $validator->errors()->getMessages()
is just like array_dot()
helper function result. So I did the Opposite of array_dot()
, also altered my attribute name into pretty name
add a comment |
up vote
0
down vote
up vote
0
down vote
For those who looking for the solution This is how I implemented
<?php
namespace AppHttpRequests;
use IlluminateContractsValidationValidator;
use IlluminateFoundationHttpFormRequest;
use IlluminateHttpExceptionsHttpResponseException;
class UserStoreRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required',
'location.city' => 'required'
'location.country' => 'required'
];
}
public function attributes()
{
return [
'location.city' => 'City'
'location.country' => 'Country'
];
}
protected function failedValidation(Validator $validator)
{
$errors = $validator->errors()->getMessages();
$errors_formated = array();
foreach ($errors as $key => $value) {
array_set($errors_formated, $key, $value);
}
throw new HttpResponseException(response()->json(['error' => $errors_formated], 422));
}
}
The result of $validator->errors()->getMessages()
is just like array_dot()
helper function result. So I did the Opposite of array_dot()
, also altered my attribute name into pretty name
For those who looking for the solution This is how I implemented
<?php
namespace AppHttpRequests;
use IlluminateContractsValidationValidator;
use IlluminateFoundationHttpFormRequest;
use IlluminateHttpExceptionsHttpResponseException;
class UserStoreRequest extends FormRequest
{
public function rules()
{
return [
'name' => 'required',
'location.city' => 'required'
'location.country' => 'required'
];
}
public function attributes()
{
return [
'location.city' => 'City'
'location.country' => 'Country'
];
}
protected function failedValidation(Validator $validator)
{
$errors = $validator->errors()->getMessages();
$errors_formated = array();
foreach ($errors as $key => $value) {
array_set($errors_formated, $key, $value);
}
throw new HttpResponseException(response()->json(['error' => $errors_formated], 422));
}
}
The result of $validator->errors()->getMessages()
is just like array_dot()
helper function result. So I did the Opposite of array_dot()
, also altered my attribute name into pretty name
edited yesterday
answered yesterday


Ebin Manuval
605722
605722
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53203641%2fformat-validation-error-message-as-nested-associative-array%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
lk6Ybk4ZLd2A UUwsK