How to shift contents referenced by numpy array names in python
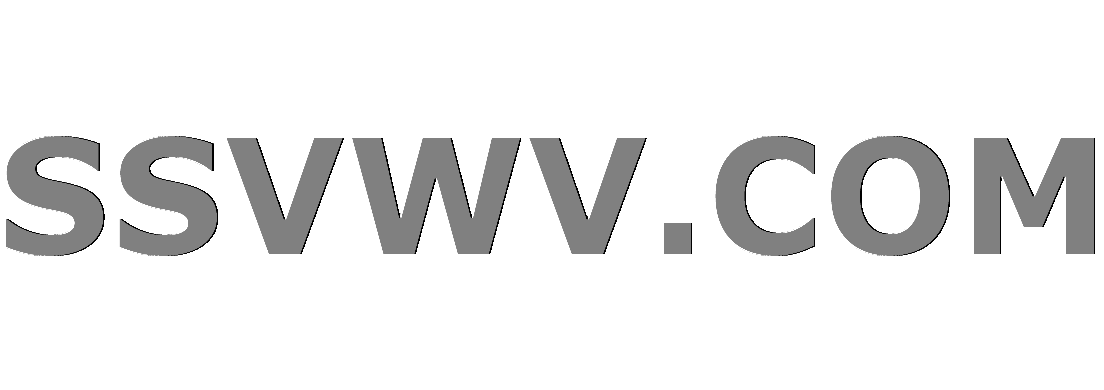
Multi tool use
up vote
0
down vote
favorite
In python, I have numpy arrays a0
, a1
, and a2
, each of which refer to different contents. I want to shift the relations between the reference names and the referred objects, so that a2
will now point to the content pointed by a1
before, and a1
will now point to the content pointed by a0
before. Then, I want to let a0
to point to a new content.
To be more specific, I want to do something like this:
import numpy as np
a2=np.array([1,2,3,4])
a1=np.array([10,20,30,40])
a0=np.array([8,8,8,8])
a2=a1
a1=a0
# I want to assign new values to a0[0], a0[1], .., without affecting a1.
Can I do it without copying values (e.g. by np.copy
) and without memory reallocation (e.g. by del
and np.empty
)?
python numpy
add a comment |
up vote
0
down vote
favorite
In python, I have numpy arrays a0
, a1
, and a2
, each of which refer to different contents. I want to shift the relations between the reference names and the referred objects, so that a2
will now point to the content pointed by a1
before, and a1
will now point to the content pointed by a0
before. Then, I want to let a0
to point to a new content.
To be more specific, I want to do something like this:
import numpy as np
a2=np.array([1,2,3,4])
a1=np.array([10,20,30,40])
a0=np.array([8,8,8,8])
a2=a1
a1=a0
# I want to assign new values to a0[0], a0[1], .., without affecting a1.
Can I do it without copying values (e.g. by np.copy
) and without memory reallocation (e.g. by del
and np.empty
)?
python numpy
Why do you need to reuse thea0
array? And where do the new values come from?
– hpaulj
yesterday
What it comes down to is that you want both an unchangeda0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.
– hpaulj
yesterday
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
In python, I have numpy arrays a0
, a1
, and a2
, each of which refer to different contents. I want to shift the relations between the reference names and the referred objects, so that a2
will now point to the content pointed by a1
before, and a1
will now point to the content pointed by a0
before. Then, I want to let a0
to point to a new content.
To be more specific, I want to do something like this:
import numpy as np
a2=np.array([1,2,3,4])
a1=np.array([10,20,30,40])
a0=np.array([8,8,8,8])
a2=a1
a1=a0
# I want to assign new values to a0[0], a0[1], .., without affecting a1.
Can I do it without copying values (e.g. by np.copy
) and without memory reallocation (e.g. by del
and np.empty
)?
python numpy
In python, I have numpy arrays a0
, a1
, and a2
, each of which refer to different contents. I want to shift the relations between the reference names and the referred objects, so that a2
will now point to the content pointed by a1
before, and a1
will now point to the content pointed by a0
before. Then, I want to let a0
to point to a new content.
To be more specific, I want to do something like this:
import numpy as np
a2=np.array([1,2,3,4])
a1=np.array([10,20,30,40])
a0=np.array([8,8,8,8])
a2=a1
a1=a0
# I want to assign new values to a0[0], a0[1], .., without affecting a1.
Can I do it without copying values (e.g. by np.copy
) and without memory reallocation (e.g. by del
and np.empty
)?
python numpy
python numpy
asked yesterday
norio
1,18921123
1,18921123
Why do you need to reuse thea0
array? And where do the new values come from?
– hpaulj
yesterday
What it comes down to is that you want both an unchangeda0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.
– hpaulj
yesterday
add a comment |
Why do you need to reuse thea0
array? And where do the new values come from?
– hpaulj
yesterday
What it comes down to is that you want both an unchangeda0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.
– hpaulj
yesterday
Why do you need to reuse the
a0
array? And where do the new values come from?– hpaulj
yesterday
Why do you need to reuse the
a0
array? And where do the new values come from?– hpaulj
yesterday
What it comes down to is that you want both an unchanged
a0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.– hpaulj
yesterday
What it comes down to is that you want both an unchanged
a0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.– hpaulj
yesterday
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
Use tuple unpacking to exchanging values kindaof like the a,b=b,a
In [183]: a2=np.array([1,2,3,4])
...: a1=np.array([10,20,30,40])
...: a0=np.array([8,8,8,8])
...:
...:
In [184]:
In [185]: a2,a1=np.copy(a1),np.copy(a0)
In [186]: a0
Out[186]: array([8, 8, 8, 8])
In [187]: a1
Out[187]: array([8, 8, 8, 8])
In [188]: a2
Out[188]: array([10, 20, 30, 40])
You are free to point a0
where ever you want and i dont think you can create get away with changing a0
and not affecting a1
without np.copy
or something else like copy.deepcopy
1
By thata0
anda1
will point to the same contend in memory, so changinga0
will also changea1
. Eventually you want to discarda2
and create a new array so I thing there is no way around copying the content ofa0
you want to keep.
– obachtos
yesterday
add a comment |
up vote
1
down vote
What are you asking is not possible without making a copy of a0
, otherwise a0
and a1
will be pointing to the same object and changing a0
will change a1
. So you should do this:
a2 = np.array([1,2,3,4])
a1 = np.array([10,20,30,40])
a0 = np.array([8,8,8,8])
a2 = a1
a1 = a0.copy()
# let's change a0
a0[0] = 9
# check
a0
Out[31]: array([9, 8, 8, 8])
a1
Out[32]: array([8, 8, 8, 8])
a2
Out[33]: array([10, 20, 30, 40])
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
Use tuple unpacking to exchanging values kindaof like the a,b=b,a
In [183]: a2=np.array([1,2,3,4])
...: a1=np.array([10,20,30,40])
...: a0=np.array([8,8,8,8])
...:
...:
In [184]:
In [185]: a2,a1=np.copy(a1),np.copy(a0)
In [186]: a0
Out[186]: array([8, 8, 8, 8])
In [187]: a1
Out[187]: array([8, 8, 8, 8])
In [188]: a2
Out[188]: array([10, 20, 30, 40])
You are free to point a0
where ever you want and i dont think you can create get away with changing a0
and not affecting a1
without np.copy
or something else like copy.deepcopy
1
By thata0
anda1
will point to the same contend in memory, so changinga0
will also changea1
. Eventually you want to discarda2
and create a new array so I thing there is no way around copying the content ofa0
you want to keep.
– obachtos
yesterday
add a comment |
up vote
2
down vote
Use tuple unpacking to exchanging values kindaof like the a,b=b,a
In [183]: a2=np.array([1,2,3,4])
...: a1=np.array([10,20,30,40])
...: a0=np.array([8,8,8,8])
...:
...:
In [184]:
In [185]: a2,a1=np.copy(a1),np.copy(a0)
In [186]: a0
Out[186]: array([8, 8, 8, 8])
In [187]: a1
Out[187]: array([8, 8, 8, 8])
In [188]: a2
Out[188]: array([10, 20, 30, 40])
You are free to point a0
where ever you want and i dont think you can create get away with changing a0
and not affecting a1
without np.copy
or something else like copy.deepcopy
1
By thata0
anda1
will point to the same contend in memory, so changinga0
will also changea1
. Eventually you want to discarda2
and create a new array so I thing there is no way around copying the content ofa0
you want to keep.
– obachtos
yesterday
add a comment |
up vote
2
down vote
up vote
2
down vote
Use tuple unpacking to exchanging values kindaof like the a,b=b,a
In [183]: a2=np.array([1,2,3,4])
...: a1=np.array([10,20,30,40])
...: a0=np.array([8,8,8,8])
...:
...:
In [184]:
In [185]: a2,a1=np.copy(a1),np.copy(a0)
In [186]: a0
Out[186]: array([8, 8, 8, 8])
In [187]: a1
Out[187]: array([8, 8, 8, 8])
In [188]: a2
Out[188]: array([10, 20, 30, 40])
You are free to point a0
where ever you want and i dont think you can create get away with changing a0
and not affecting a1
without np.copy
or something else like copy.deepcopy
Use tuple unpacking to exchanging values kindaof like the a,b=b,a
In [183]: a2=np.array([1,2,3,4])
...: a1=np.array([10,20,30,40])
...: a0=np.array([8,8,8,8])
...:
...:
In [184]:
In [185]: a2,a1=np.copy(a1),np.copy(a0)
In [186]: a0
Out[186]: array([8, 8, 8, 8])
In [187]: a1
Out[187]: array([8, 8, 8, 8])
In [188]: a2
Out[188]: array([10, 20, 30, 40])
You are free to point a0
where ever you want and i dont think you can create get away with changing a0
and not affecting a1
without np.copy
or something else like copy.deepcopy
edited yesterday
answered yesterday


Albin Paul
1,180517
1,180517
1
By thata0
anda1
will point to the same contend in memory, so changinga0
will also changea1
. Eventually you want to discarda2
and create a new array so I thing there is no way around copying the content ofa0
you want to keep.
– obachtos
yesterday
add a comment |
1
By thata0
anda1
will point to the same contend in memory, so changinga0
will also changea1
. Eventually you want to discarda2
and create a new array so I thing there is no way around copying the content ofa0
you want to keep.
– obachtos
yesterday
1
1
By that
a0
and a1
will point to the same contend in memory, so changing a0
will also change a1
. Eventually you want to discard a2
and create a new array so I thing there is no way around copying the content of a0
you want to keep.– obachtos
yesterday
By that
a0
and a1
will point to the same contend in memory, so changing a0
will also change a1
. Eventually you want to discard a2
and create a new array so I thing there is no way around copying the content of a0
you want to keep.– obachtos
yesterday
add a comment |
up vote
1
down vote
What are you asking is not possible without making a copy of a0
, otherwise a0
and a1
will be pointing to the same object and changing a0
will change a1
. So you should do this:
a2 = np.array([1,2,3,4])
a1 = np.array([10,20,30,40])
a0 = np.array([8,8,8,8])
a2 = a1
a1 = a0.copy()
# let's change a0
a0[0] = 9
# check
a0
Out[31]: array([9, 8, 8, 8])
a1
Out[32]: array([8, 8, 8, 8])
a2
Out[33]: array([10, 20, 30, 40])
add a comment |
up vote
1
down vote
What are you asking is not possible without making a copy of a0
, otherwise a0
and a1
will be pointing to the same object and changing a0
will change a1
. So you should do this:
a2 = np.array([1,2,3,4])
a1 = np.array([10,20,30,40])
a0 = np.array([8,8,8,8])
a2 = a1
a1 = a0.copy()
# let's change a0
a0[0] = 9
# check
a0
Out[31]: array([9, 8, 8, 8])
a1
Out[32]: array([8, 8, 8, 8])
a2
Out[33]: array([10, 20, 30, 40])
add a comment |
up vote
1
down vote
up vote
1
down vote
What are you asking is not possible without making a copy of a0
, otherwise a0
and a1
will be pointing to the same object and changing a0
will change a1
. So you should do this:
a2 = np.array([1,2,3,4])
a1 = np.array([10,20,30,40])
a0 = np.array([8,8,8,8])
a2 = a1
a1 = a0.copy()
# let's change a0
a0[0] = 9
# check
a0
Out[31]: array([9, 8, 8, 8])
a1
Out[32]: array([8, 8, 8, 8])
a2
Out[33]: array([10, 20, 30, 40])
What are you asking is not possible without making a copy of a0
, otherwise a0
and a1
will be pointing to the same object and changing a0
will change a1
. So you should do this:
a2 = np.array([1,2,3,4])
a1 = np.array([10,20,30,40])
a0 = np.array([8,8,8,8])
a2 = a1
a1 = a0.copy()
# let's change a0
a0[0] = 9
# check
a0
Out[31]: array([9, 8, 8, 8])
a1
Out[32]: array([8, 8, 8, 8])
a2
Out[33]: array([10, 20, 30, 40])
answered yesterday
AndyK
781518
781518
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53203678%2fhow-to-shift-contents-referenced-by-numpy-array-names-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
CEJUT2,Gf4tkT
Why do you need to reuse the
a0
array? And where do the new values come from?– hpaulj
yesterday
What it comes down to is that you want both an unchanged
a0
array, and a modified version of it. The fact that you are reusing the names ('shifting') doesn't matter.– hpaulj
yesterday